GitHub - cebor/angular-highcharts: Highcharts directive for AngularHighcharts directive for Angular. Contribute to cebor/angular-highcharts development by creating an account on GitHub.
Visit Site
GitHub - cebor/angular-highcharts: Highcharts directive for Angular
angular-highcharts
This is a directive for an easy usage of Highcharts with angular.
Requirements
{
"angular": "^15.1.1",
"highcharts": "^10.3.3"
}
Installation
yarn
# install angular-highcharts and highcharts
yarn add angular-highcharts highcharts
npm
# install angular-highcharts and highcharts
npm i --save angular-highcharts highcharts
Usage Example
// app.module.ts
import { ChartModule } from 'angular-highcharts';
@NgModule({
imports: [
ChartModule // add ChartModule to your imports
]
})
export class AppModule {}
// chart.component.ts
import { Chart } from 'angular-highcharts';
@Component({
template: `
<button (click)="add()">Add Point!</button>
<div [chart]="chart"></div>
`
})
export class ChartComponent {
chart = new Chart({
chart: {
type: 'line'
},
title: {
text: 'Linechart'
},
credits: {
enabled: false
},
series: [
{
name: 'Line 1',
data: [1, 2, 3]
}
]
});
// add point to chart serie
add() {
this.chart.addPoint(Math.floor(Math.random() * 10));
}
}
API Docs
Chart
The Chart object.
Type: class
Constructor
new Chart(options: Options)
Properties
ref: Highcharts.Chart;
Deprecated. Please use ref$
.
ref$: Observable<Highcharts.Chart>
Observable that emits a Highcharts.Chart - Official Chart API Docs
Methods
addPoint(point: Point, [serieIndex: number = 0]): void
Adds a point to a serie
removePoint(pointIndex: number, [serieIndex: number = 0], [redraw: boolean = true], [shift: boolean = false]): void
Removes a point from a serie
addSeries(series: ChartSerie): void
Adds a series to the chart
removeSeries(seriesIndex: number): void
Remove series from the chart
StockChart
The Chart object.
Type: class
Constructor
new StockChart(options);
Properties
ref: Highstock.Chart;
Deprecated. Please use ref$
.
ref$: Observable<Highstock.Chart>
Observable that emits a Highstock.Chart
MapChart
The Chart object.
Type: class
Constructor
new MapChart(options);
Properties
ref;
Deprecated. Please use ref$
.
ref$;
Observable that emits a Highmaps.Chart
Using Highcharts modules
To use Highcharts modules you have to import them and provide them in a factory (required for aot).
You can find the list of available modules in the highcharts folder ls -la node_modules/highcharts/modules
.
Hint: Highcharts-more is a exception, you find this module in the root folder.
Don't forget to use the modules with the .src
suffix, minimized highcharts modules are not importable.
Example
// app.module.ts
import { ChartModule, HIGHCHARTS_MODULES } from 'angular-highcharts';
import * as more from 'highcharts/highcharts-more.src';
import * as exporting from 'highcharts/modules/exporting.src';
@NgModule({
providers: [
{ provide: HIGHCHARTS_MODULES, useFactory: () => [ more, exporting ] } // add as factory to your providers
]
})
export class AppModule { }
Troubleshooting
Compile Issues
If you expiring typing errors while you build/serve your angular app the following could be helpful:
// override options type with <any>
chart = new Chart({ options } as any);
This is very useful when using gauge chart
type.
Loading Highcharts Modules manually
See Official Highcharts Docs: http://www.highcharts.com/docs/getting-started/install-from-npm
Demo
License
MIT © Felix Itzenplitz
More Resourcesto explore the angular.
mail [email protected] to add your project or resources here 🔥.
- 1rxjs-fruits.com
https://www.rxjs-fruits.com/subscribe
A game for learning RxJS 🍎🍌
- 2Learn to become a Angular developer
https://roadmap.sh/angular
Community driven, articles, resources, guides, interview questions, quizzes for angular development. Learn to become a modern Angular developer by following the steps, skills, resources and guides listed in this roadmap.
- 3Join the Angular Community Discord Server!
https://discord.com/invite/angular
Check out the Angular Community community on Discord - hang out with 42037 other members and enjoy free voice and text chat.
- 4Newsletter
https://ultimatecourses.com/newsletter
Join our email community
- 5thePunderWoman 🏳️🌈 (@[email protected])
https://mastodon.social/@jessicajaniuk
16 Posts, 42 Following, 27 Followers · I'm Jessica. Angular Team @Google | Historical Fencer, R2-D2 Builder, Gamer, Cosplayer, Punster, Paladin | She/Her | Opinions are my own | #LLAP 🖖🏻
- 6Angular University
https://blog.angular-university.io/
High-Quality Angular Courses
- 7ZITADEL with Angular | ZITADEL Docs
https://zitadel.com/docs/examples/login/angular
This integration guide demonstrates the recommended way to incorporate ZITADEL into your Angular application.
- 8Angular Air
https://angularair.com/
A live videocast on YouTube about Angular, a JavaScript platform for building mobile and desktop web applications.
- 9Angular Packages Discovery
https://ngx.tools
Discover Angular packages, libraries and schematics
- 10justangular.com
https://justangular.com/
The blog you would like to read if you are a beginner or an expert in Angular. It is a comprehensive resource designed to cater to all levels of proficiency, ensuring a seamless learning experience for newcomers and seasoned developers alike.
- 11NgServe.io - Serving the Angular and Web Developer Community
https://www.ngserve.io/
Angular, NgRx, Firebase, and Google Cloud Tutorials helping serve the angular and web development communities.
- 12Angular - Halodoc Blog
https://blogs.halodoc.io/tag/angular-2-2/
Simplifying Healthcare!
- 13Tomasz Ducin - blog
https://ducin.dev/blog
Independent Software Consultant / Interface Architect / Trainer working in Warsaw, Poland. IT Community Activist.
- 14Translate Angular app with AI - GlobalSeo
https://www.globalseo.ai/integrations/angular
Translate your angular app with GPT-4. Try for free.
- 15axe Accessibility Linter - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=deque-systems.vscode-axe-linter
Extension for Visual Studio Code - Accessibility linting for HTML, Angular, React, Markdown, Vue, and React Native
- 16Practical Angular Newsletter by Angular Mentor
https://angularmentor.io/practical-angular-newsletter
Newsletter for Angular developers that teaches solving typical applications problems - How to do, When to use.
- 17Angular-Buch → Entwickeln Sie moderne Apps für Web und Mobile.
https://angular-buch.com/
📙 Alles zum deutschprachigen Fachbuch vom dpunkt.verlag in der 4. Auflage. Jetzt bestellen. Grundlagen, fortgeschrittene Themen und Best Practices
- 18Angular Experts Podcast - Angular Experts
https://angularexperts.io/podcasts/
Learn all about Angular, NgRx, RxJs & NX and advance your skills with guides, in-depth content and actionable tips and tricks with Tomas Trajan, Kevin Kreuzer and the guests!
- 19Home
https://www.w3.org/WAI/
Accessibility resources free online from the international standards organization: W3C Web Accessibility Initiative (WAI).
- 20Angular on Netlify
https://docs.netlify.com/frameworks/angular/
Learn about Angular on our platform. Rely on our automatic framework detection and add redirects for your Angular application.
- 21simplified Angular Blog
https://blog.simplified.courses/
Tech blog where we explain Simplified Angular principles and proven practices
- 22Angular posts on daily.dev
https://app.daily.dev/tags/angular
Discover Angular, a popular JavaScript framework for building web applications. Learn about Angular components, services, and reactive programming with RxJS. Whether you're a frontend developer, web designer, or JavaScript enthusiast, create dynamic and robust web applications with Angular.
- 23Analog | Analog
https://analogjs.org
The fullstack Angular meta-framework
- 24katsuba.dev
https://blog.katsuba.dev/
Discover the latest in Angular, TypeScript, JavaScript, and web development with Techlead's Diary. Get expert tutorials, tech reviews, and career guidance in IT.
- 25Frontend Handbook | Angular / Introduction
https://infinum.com/handbook/frontend/angular/introduction
Wow users with eye-catching web experience
- 26Angular Material
https://material.angular.io/cdk/a11y/overview
UI component infrastructure and Material Design components for Angular web applications.
- 27webpack
https://webpack.js.org
webpack is a module bundler. Its main purpose is to bundle JavaScript files for usage in a browser, yet it is also capable of transforming, bundling, or packaging just about any resource or asset.
- 28Accessibility for teams
https://digital.gov/guides/accessibility-for-teams/
A ‘quick-start’ guide for embedding accessibility and inclusive design practices into your team’s workflow
- 29Learn how to build apps with modern reactive and declarative code
https://modernangular.com/
Learn how to build apps with modern reactive and declarative code
- 30Angular
https://angular.dev
The web development framework for building modern apps.
- 31Micro Frontends & Moduliths
https://www.angulararchitects.io/en/ebooks/micro-frontends-and-moduliths-with-angular/
Learn how to build enterprise-scale Angular applications which are maintainable in the long run. Free eBook by Manfred Steyer, GDE.
- 32Angular Cookbook - Muhammad Ahsan Ayaz
https://codewithahsan.dev/ng-book
The one stop shop to have fun with amazing people, and to code of course.
- 33Ultimate Angular™ - Learn Everything You Need To Master Angular
https://ultimatecourses.com/courses/angular
Learn latest Angular, RxJS, TypeScript, through to NGRX and beyond. Become an Angular expert online via our online courses.
- 34Best AI tools made with Angular on aitools.fyi
https://aitools.fyi/technology/angular
Explore the ultimate collection of the best AI tools made with Angular on aitools.fyi. Visit aitools.fyi to discover the best ai tools.
- 35Angular Certification | Created by Google Developer Experts
https://certificates.dev/angular
Become a certified Angular Dev. Master components, RxJS, & more with our online certification. 3 levels, guaranteed industry-relevant. Get certified, get hired!
- 36Step-by-Step Guide
https://vishugosain.hashnode.dev/integrating-open-ai-into-angular-application
Check out our article to learn how to integrate ChatGPT into an angular application in three simple steps.
- 37Angular - Solutions – Vercel
https://vercel.com/solutions/angular
Deploy Angular with Vercel, the most approachable deployment platform, with zero configuration. The world's leading companies use Vercel to deploy production-grade websites.
- 38Angular Cheat Sheet + PDF | Zero To Mastery
https://zerotomastery.io/cheatsheets/angular-cheat-sheet/
Learn and remember key functions and concepts of Angular with this handy quick reference guide (+ PDF) to the fundamentals of Angular.
- 39Angular Weekly | Yevhenii Herasymchuk | Substack
https://angularweekly.substack.com/
Angular Weekly is a summary of #angular related topics and news from the last week. Click to read Angular Weekly, by Yevhenii Herasymchuk, a Substack publication with hundreds of subscribers.
- 40Amplify Docs - AWS Amplify Gen 2 Documentation
https://docs.amplify.aws/angular/
AWS Amplify Docs - Develop and deploy cloud-powered web and mobile apps. AWS Amplify Documentation
- 41Blog - qupaya
https://qupaya.com/blog/
We love to spread the word and want to support you even on an open basis without any fees. Enjoy reading our blog about the the Angular and NestJS ecosystem, and insights from our field of industry.
- 42Angular Certification | Angular Academy
https://www.angularacademy.ca/angular-certification
Angular Academy is the #1 provider of hands-on instructor-led classroom training in Canada!
- 43This is Angular — DEV Community Profile
https://dev.to/this-is-angular
Free, open and honest Angular education.
- 44Angular Catch-Up | Jaroslaw Zolnowski
https://angular-catch-up.podbean.com/
Welcome to Angular Catch-Up, your ultimate destination for everything Angular! Whether you’re a seasoned pro or just dipping your toes into the world of web development, our podcast is your one-stop-shop for all things Angular. Join us as we unpack the lat...
- 45Angular
https://angular.dev/tutorials/learn-angular
The web development framework for building modern apps.
- 46Simplified Courses - Angular training
https://www.simplified.courses/angular-training
Brecht provides remote Angular training! We will set up a zoom call and teach you about my experience in Angular in more than 100 projects
- 47Newsletter | Danywalls | Angular | Kendo | Typescript
https://www.danywalls.com/newsletter
Subscribe to Danywalls | Angular | Kendo | Typescript's newsletter.
- 48Zerops — developer first cloud platform
https://zerops.io/
Zerops is a cloud platform that builds, deploys, runs and manages your apps, no matter the size or environment.
- 49Learn to build professional-grade Angular Applications
https://angularstart.com/
Learn to build professional-grade Angular Applications
- 50Angular.Schule → ANGULAR-Schulungen für Ihr Team
https://angular.schule/
Lernen Sie Angular, RxJS & Redux/NgRx. ✅ Schulung aus der Praxis von den Buchautoren. ✅ Auch als Inhouse-Schulung buchbar. ✅ Jetzt Platz sichern!
- 51The Angular Plus Show
https://open.spotify.com/show/1PrLErQHBqBhZsRV1KHhGM
Podcast · [object Object] · The Angular Plus Show is the home of ng-conf's official all-Angular podcast. Come here to stay up to date on the latest changes in the Angular community. Expect to laugh and cry with us as we talk about our experiences as Angular developers.
- 52Angular Academy
https://www.angularacademy.ca/
Angular Academy is the #1 provider of hands-on instructor-led classroom training in Canada!
- 53ngx-tableau
https://www.npmjs.com/package/ngx-tableau
ngx-tableau is an Angular module that allows to embed a [Tableau](https://www.tableau.com) report in an Angular webapp. You can see a working **DEMO** [here](https://stackblitz.com/edit/ngx-tableau).. Latest version: 2.1.0, last published: 6 months ago. Start using ngx-tableau in your project by running `npm i ngx-tableau`. There are no other projects in the npm registry using ngx-tableau.
- 54The Framework Field Guide
https://playfulprogramming.com/collections/framework-field-guide
A practical and free way to teach Angular, React, and Vue all at once, so you can choose the right tool for the job and learn the underlying concepts in depth.
- 55Angular Signals eBook by Kevin Kreuzer - Angular Experts
https://angularexperts.io/products/ebook-signals
Discover the future of Angular with our brand new Angular Signals ebook. Master the API and explore how Signals work behind the scenes. Elevate your development skills and prepare yourself for the future of Angular. Get ahead today!
- 56Learn Angular - Best Angular Tutorials | Hackr.io
https://hackr.io/tutorials/learn-angular
Learning Angular? Check out these best online Angular courses and tutorials recommended by the programming community. Pick the tutorial as per your learning style: video tutorials or a book. Free course or paid. Tutorials for beginners or advanced learners. Check Angular community's reviews & comments.
- 57Angular Blog - Angular Experts
https://angularexperts.io/blog
Learn all about Angular, NgRx, RxJs & NX from our blog and advance your skills with guides, in-depth content and actionable tips and tricks
- 58🪄 OZ 🎩 – Medium
https://medium.com/@eugeniyoz
Read writing from 🪄 OZ 🎩 on Medium. Web dev, Angular/Rust 🗞️ https://twitter.com/eugeniyoz 🗞️ https://jamm.dev/. Every day, 🪄 OZ 🎩 and thousands of other voices read, write, and share important stories on Medium.
- 59Angularidades
https://podcasts.apple.com/us/podcast/angularidades/id1702444448
Listen to Alejandro Cuba Ruiz's Angularidades podcast on Apple Podcasts.
- 60Angular Addicts | Gergely Szerovay | Substack
https://www.angularaddicts.com/
In this publication we collect hand-selected articles & other resources — e.g. books, talks, podcast episodes — about Angular. Whether you are a professional or a beginner, you’ll find valuable Angular knowledge here. Click to read Angular Addicts, by Gergely Szerovay, a Substack publication with thousands of subscribers.
- 61Angular
https://angular.dev/tools/cli
The web development framework for building modern apps.
- 62Younes Jaaidi (Marmicode)
https://www.eventbrite.fr/o/younes-jaaidi-marmicode-29329031085
Younes is a Software Cook born in eXtreme Programming, which made him a passionate advocate of Collective Ownership, TDD, and snacks in the office.He trains and coaches teams like yours to cook better apps. He’s also an NX champion, an Angular GDE, and a average sailor.His favorite command is:sleep 300; git reset --hard; git clean -df
- 63A CLI tool that removes empty style files from your Angular components.
https://github.com/BernardoGiordano/ngx-stylesweep
A CLI tool that removes empty style files from your Angular components. - BernardoGiordano/ngx-stylesweep
- 64Clerk | Authentication and User Management
https://clerk.com
The easiest way to add authentication and user management to your application. Purpose-built for React, Next.js, Remix, and “The Modern Web”.
- 65CLI tool to generate angular chrome extensions
https://github.com/larscom/ng-chrome-extension
CLI tool to generate angular chrome extensions. Contribute to larscom/ng-chrome-extension development by creating an account on GitHub.
- 66Build an AI Image Generator in Angular 17 Using the Clipdrop API || AI Text to Image Generator
https://github.com/desoga10/ng-text-to-image
Build an AI Image Generator in Angular 17 Using the Clipdrop API || AI Text to Image Generator - desoga10/ng-text-to-image
- 67Supabase | The Open Source Firebase Alternative
https://supabase.com/
Build production-grade applications with a Postgres database, Authentication, instant APIs, Realtime, Functions, Storage and Vector embeddings. Start for free.
- 68Angular
https://angular.dev/overview
The web development framework for building modern apps.
- 69Angular Design Patterns and Best Practices, Published by Packt
https://github.com/PacktPublishing/Angular-Design-Patterns-and-Best-Practices
Angular Design Patterns and Best Practices, Published by Packt - PacktPublishing/Angular-Design-Patterns-and-Best-Practices
- 70Tomas Trajan – Medium
https://tomastrajan.medium.com/
Read writing from Tomas Trajan on Medium. 👋 I build, teach, write & speak about #Angular & #NgRx for enterprises 👨💻 Google Developer Expert #GDE 👨🏫 @AngularZurich meetup co-organizer.
- 71Thomas Laforge – Medium
https://medium.com/@thomas.laforge
Read writing from Thomas Laforge on Medium. Software Engineer | GoogleDevExpert in Angular 🅰️ | #AngularChallenges creator. Every day, Thomas Laforge and thousands of other voices read, write, and share important stories on Medium.
- 72google-gemini/angular-webxr-art-sample
https://github.com/google-gemini/angular-webxr-art-sample
Contribute to google-gemini/angular-webxr-art-sample development by creating an account on GitHub.
- 73🤖 Stream realtime generative AI to your Angular app using Google Gemini, HttpClient, RxJS, and Signals
https://github.com/c-o-l-i-n/ng-generative-ai-demo
🤖 Stream realtime generative AI to your Angular app using Google Gemini, HttpClient, RxJS, and Signals - c-o-l-i-n/ng-generative-ai-demo
- 74Analog Node.js on Zerops
https://github.com/zeropsio/recipe-analog-nodejs
Analog Node.js on Zerops. Contribute to zeropsio/recipe-analog-nodejs development by creating an account on GitHub.
- 75DenysVuika/angular-book
https://github.com/DenysVuika/angular-book
Contribute to DenysVuika/angular-book development by creating an account on GitHub.
- 76Helper library for handling JWTs in Angular apps
https://github.com/auth0/angular2-jwt
Helper library for handling JWTs in Angular apps. Contribute to auth0/angular2-jwt development by creating an account on GitHub.
- 77angular-builders/packages/bazel at master · just-jeb/angular-builders
https://github.com/just-jeb/angular-builders/tree/master/packages/bazel
Angular build facade extensions (Jest and custom webpack configuration) - just-jeb/angular-builders
- 78Free ebook
https://houseofangular.io/the-ultimate-guide-to-angular-evolution/
A comprehensive guide to Angular versions and the changes they brought. Discover how to leverage each feature to tackle challenges and improve app performance.
- 79Blog - This Dot Labs - This Dot Labs
https://www.thisdot.co/blog?tags=angular
This Dot provides teams with technical leaders who bring deep knowledge of the web platform. We help teams set new standards, and deliver results predictably.
- 80Angular build facade extensions (Jest and custom webpack configuration)
https://github.com/just-jeb/angular-builders
Angular build facade extensions (Jest and custom webpack configuration) - GitHub - just-jeb/angular-builders: Angular build facade extensions (Jest and custom webpack configuration)
- 81ai-test-gen-angular is a powerful tool that leverages OpenAI's advanced capabilities to automate the generation of unit tests for your Angular components and services
https://github.com/DurgeshRathod/ai-test-gen-angular
ai-test-gen-angular is a powerful tool that leverages OpenAI's advanced capabilities to automate the generation of unit tests for your Angular components and services - DurgeshRathod/ai-test-ge...
- 82Compile and package Angular libraries in Angular Package Format (APF)
https://github.com/ng-packagr/ng-packagr
Compile and package Angular libraries in Angular Package Format (APF) - ng-packagr/ng-packagr
- 83Mini-Bard client for Angular using Gemini Pro via API key from Google AI Studio
https://github.com/gsans/mini-bard-palm2-angular
Mini-Bard client for Angular using Gemini Pro via API key from Google AI Studio - gsans/mini-bard-palm2-angular
- 84An open-source accessibility widget written in Angular
https://github.com/verto-health/astral-accessibility
An open-source accessibility widget written in Angular - verto-health/astral-accessibility
- 85Angular web app for AI-generated image descriptions
https://github.com/slsfi/abbi-ng-ai-image-descriptor
Angular web app for AI-generated image descriptions - slsfi/abbi-ng-ai-image-descriptor
- 86Angular schematics and builder to retrieve values from System Environment (OS) variables and update relevant `environment.ts` file.
https://github.com/danduh/ng-process-env
Angular schematics and builder to retrieve values from System Environment (OS) variables and update relevant `environment.ts` file. - danduh/ng-process-env
- 87A showcase of web apps built with AngularJS
https://github.com/madewithangular/madewithangular.github.io
A showcase of web apps built with AngularJS. Contribute to madewithangular/madewithangular.github.io development by creating an account on GitHub.
- 88Discover Angular packages, libraries and schematics 🚀
https://github.com/ngxtools/ngx.tools
Discover Angular packages, libraries and schematics 🚀 - ngxtools/ngx.tools
- 89Angular Adsense Component
https://github.com/scttcper/ng2-adsense
Angular Adsense Component. Contribute to scttcper/ng2-adsense development by creating an account on GitHub.
- 90M.A.N.T.I.S (MongoDB, Angular with Analog, Nx, Tailwind CSS, Ionic, Storybook) is not just a CLI tool; it's your passport to a seamless full-stack project launch.
https://github.com/mantis-apps/mantis-cli
M.A.N.T.I.S (MongoDB, Angular with Analog, Nx, Tailwind CSS, Ionic, Storybook) is not just a CLI tool; it's your passport to a seamless full-stack project launch. - GitHub - mantis-apps/mantis...
- 91Security directives for your Angular application to show/hide elements based on a user roles / permissions.
https://github.com/mselerin/ngx-security
Security directives for your Angular application to show/hide elements based on a user roles / permissions. - mselerin/ngx-security
- 92An @angular/cli based starter containing common components and services as well as a reference site.
https://github.com/bluehalo/ngx-starter
An @angular/cli based starter containing common components and services as well as a reference site. - bluehalo/ngx-starter
- 93🔥 Curated list of common mistakes made when developing Angular applications
https://github.com/typebytes/angular-checklist
🔥 Curated list of common mistakes made when developing Angular applications - typebytes/angular-checklist
- 94This repo is dedicated to collect all the different kinds of Angular badges / logos. This can be a logo from a conference, a NPM package, ...
https://github.com/maartentibau/angular-logos
This repo is dedicated to collect all the different kinds of Angular badges / logos. This can be a logo from a conference, a NPM package, ... - maartentibau/angular-logos
- 95Builder to generate "src/environments/environment.ts" file based on your environment variables
https://github.com/igorissen/angular-env-builder
Builder to generate "src/environments/environment.ts" file based on your environment variables - igorissen/angular-env-builder
- 96📈 A simple Google analytics integration for Angular apps
https://github.com/hakimio/ngx-google-analytics
📈 A simple Google analytics integration for Angular apps - hakimio/ngx-google-analytics
- 97Extend the Angular CLI's default build behavior without ejecting, e. g. for Angular Elements
https://github.com/manfredsteyer/ngx-build-plus
Extend the Angular CLI's default build behavior without ejecting, e. g. for Angular Elements - manfredsteyer/ngx-build-plus
- 98Curated list of Angular events and communities
https://github.com/angular-sanctuary/angular-hub
Curated list of Angular events and communities. Contribute to angular-sanctuary/angular-hub development by creating an account on GitHub.
- 99This repository hosts an Angular-based project designed as a api integration exercise.
https://github.com/ppsdang/angular-api-challenge
This repository hosts an Angular-based project designed as a api integration exercise. - GitHub - ppsdang/angular-api-challenge: This repository hosts an Angular-based project designed as a api in...
- 100A library for integrating VLibras into Angular applications.
https://github.com/angular-a11y/angular-vlibras
A library for integrating VLibras into Angular applications. - angular-a11y/angular-vlibras
- 101angular-builders/packages/timestamp at master · just-jeb/angular-builders
https://github.com/just-jeb/angular-builders/tree/master/packages/timestamp
Angular build facade extensions (Jest and custom webpack configuration) - just-jeb/angular-builders
- 102casl/packages/casl-angular at master · stalniy/casl
https://github.com/stalniy/casl/tree/master/packages/casl-angular
CASL is an isomorphic authorization JavaScript library which restricts what resources a given user is allowed to access - stalniy/casl
- 103Analytics for developers. Setup Analytics in 30 seconds with just one line of code. Display all your data on an AI-powered dashboard. Fully self-hostable and GDPR compliant.
https://github.com/Litlyx/litlyx
Analytics for developers. Setup Analytics in 30 seconds with just one line of code. Display all your data on an AI-powered dashboard. Fully self-hostable and GDPR compliant. - Litlyx/litlyx
- 104Newest 'angular' Questions
https://stackoverflow.com/questions/tagged/angular
Stack Overflow | The World’s Largest Online Community for Developers
- 105Build a User Management App with Angular | Supabase Docs
https://supabase.com/docs/guides/getting-started/tutorials/with-angular
Learn how to use Supabase in your Angular App.
- 106Seamlessly load environment variables. Supports cli, esbuild, rollup, vite, webpack, angular. ESM and Monorepos.
https://github.com/chihab/dotenv-run
Seamlessly load environment variables. Supports cli, esbuild, rollup, vite, webpack, angular. ESM and Monorepos. - chihab/dotenv-run
- 107Hooks for the angular-cli
https://github.com/smartin85/ng-cli-hooks
Hooks for the angular-cli. Contribute to smartin85/ng-cli-hooks development by creating an account on GitHub.
- 108A Github Action for the Deployment of an Analog.js App on Github Pages
https://github.com/k9n-dev/analog-publish-gh-pages
A Github Action for the Deployment of an Analog.js App on Github Pages - k9n-dev/analog-publish-gh-pages
- 109arolleaguekeng/ng-flexbot
https://github.com/arolleaguekeng/ng-flexbot
Contribute to arolleaguekeng/ng-flexbot development by creating an account on GitHub.
- 110Easy Keycloak setup for Angular applications.
https://github.com/mauriciovigolo/keycloak-angular
Easy Keycloak setup for Angular applications. Contribute to mauriciovigolo/keycloak-angular development by creating an account on GitHub.
- 111A suite of Electron tools for the Angular framework
https://github.com/bampakoa/ngx-electronify
A suite of Electron tools for the Angular framework - bampakoa/ngx-electronify
- 112Vendor-agnostic analytics for Angular2 applications.
https://github.com/angulartics/angulartics2
Vendor-agnostic analytics for Angular2 applications. - angulartics/angulartics2
- 113Showcase and market your AnalogJS apps | Open Source Project
https://github.com/TechShowcase/builtwithanalog
Showcase and market your AnalogJS apps | Open Source Project - TechShowcase/builtwithanalog
- 114Angular Full Course | Advanced Online Training & Certification
https://www.koenig-solutions.com/angularjs-training-certification-courses
Master Angular with Koenig Solutions! Offering Angular full course, advanced Angular training, and online certification. Boost your IT skills today.
- 115Angular Certification Training Course Online
https://www.edureka.co/angular-training
Edureka's Angular Certification Training Course is designed to help you master front-end web development with Angular and become a certified Angular Developer.
- 116CLI tool for Angular
https://github.com/angular/angular-cli
CLI tool for Angular. Contribute to angular/angular-cli development by creating an account on GitHub.
- 117Deploy on the fastest full-stack cloud. Experience the autoscaling of near-zero latency serverless without rewriting your code.
https://github.com/Genez-io/genezio
Deploy on the fastest full-stack cloud. Experience the autoscaling of near-zero latency serverless without rewriting your code. - Genez-io/genezio
- 118npm package for OpenID Connect, OAuth Code Flow with PKCE, Refresh tokens, Implicit Flow
https://github.com/damienbod/angular-auth-oidc-client
npm package for OpenID Connect, OAuth Code Flow with PKCE, Refresh tokens, Implicit Flow - damienbod/angular-auth-oidc-client
- 119An Angular application that demonstrates best practices for user authentication & authorization flows.
https://github.com/nikosanif/angular-authentication
An Angular application that demonstrates best practices for user authentication & authorization flows. - nikosanif/angular-authentication
- 120Fully customizable AI chatbot component for your website
https://github.com/OvidijusParsiunas/deep-chat
Fully customizable AI chatbot component for your website - OvidijusParsiunas/deep-chat
- 121angular-builders/packages/custom-esbuild at master · just-jeb/angular-builders
https://github.com/just-jeb/angular-builders/tree/master/packages/custom-esbuild
Angular build facade extensions (Jest and custom webpack configuration) - just-jeb/angular-builders
- 122Auth0 SDK for Angular Single Page Applications
https://github.com/auth0/auth0-angular
Auth0 SDK for Angular Single Page Applications. Contribute to auth0/auth0-angular development by creating an account on GitHub.
- 123A collection of Angular memes
https://github.com/dzhavat/angular-memes
A collection of Angular memes. Contribute to dzhavat/angular-memes development by creating an account on GitHub.
- 124Analog Static on Zerops
https://github.com/zeropsio/recipe-analog-static
Analog Static on Zerops. Contribute to zeropsio/recipe-analog-static development by creating an account on GitHub.
- 125Deliver web apps with confidence 🚀
https://github.com/angular/angular
Deliver web apps with confidence 🚀. Contribute to angular/angular development by creating an account on GitHub.
- 126This repository hosts an Angular-based project designed as a coding and debugging exercise.
https://github.com/ppsdang/angular-debugging-challenge
This repository hosts an Angular-based project designed as a coding and debugging exercise. - GitHub - ppsdang/angular-debugging-challenge: This repository hosts an Angular-based project designed ...
- 127A chapterwise tutorial that will take you through the fundamentals of modern authentication with Microsoft identity platform in Angular using MSAL Angular v2
https://github.com/Azure-Samples/ms-identity-javascript-angular-tutorial
A chapterwise tutorial that will take you through the fundamentals of modern authentication with Microsoft identity platform in Angular using MSAL Angular v2 - Azure-Samples/ms-identity-javascript-...
- 128Social login and authentication module for Angular 17
https://github.com/abacritt/angularx-social-login
Social login and authentication module for Angular 17 - abacritt/angularx-social-login
- 129angular | Software Development » Web Development » Javascript | Gumroad
https://gumroad.com/software-development/web-development/javascript?tags=angular
Browse over 1.6 million free and premium digital products in education, tech, design, and more categories from Gumroad creators and online entrepreneurs.
- 130The Angular Runtime allows Angular to run on Netlify with zero configuration
https://github.com/netlify/angular-runtime
The Angular Runtime allows Angular to run on Netlify with zero configuration - netlify/angular-runtime
- 131Google Developer Experts - Google for Developers
https://developers.google.com/experts/all/technology/web-technologies
Apply to join the Google Developer Experts community, a global network of professionals full of technology experts, influencers, and thought leaders.
- 132Netanel Basal – Medium
https://medium.com/@netbasal
Read writing from Netanel Basal on Medium. A FrontEnd Tech Lead, blogger, and open source maintainer. The founder of ngneat, husband and father. Every day, Netanel Basal and thousands of other voices read, write, and share important stories on Medium.
- 133Rainer Hahnekamp – Medium
https://medium.com/@rainer-hahnekamp
Read writing from Rainer Hahnekamp on Medium. Rainer Hahnekamp is a Google Developer Expert, working as a trainer and consultant in the expert network of Angular Architects..
- 134Angular Universal module for Nest framework (node.js) 🌷
https://github.com/nestjs/ng-universal
Angular Universal module for Nest framework (node.js) 🌷 - nestjs/ng-universal
- 135Convert Designs to Clean Angular Code in a Click
https://www.builder.io/blog/figma-to-angular
Use Visual Copilot to swiftly and accurately convert Figma designs to clean and responsive Angular code using AI.
- 136Online Angular Certification Course | Angular Training
https://www.simplilearn.com/angular-certification-training-course
Our online Angular Certification Training Course includes ✔️50 hrs of blended learning ✔️3 industry projects to help you learn Angular concepts. Enroll now!
- 137Angular Blog
https://blog.angular.dev/
The latest news and tips from the Angular team.
- 138Angular SDK
https://dev.fingerprint.com/docs/angular
The Fingerprint Angular SDK is an easy way to integrate Fingerprint into your Angular application. It supports all capabilities of the JS agent and provides a built-in caching mechanism. How to install Add @fingerprintjs/fingerprintjs-pro-angular as a dependency to your application via npm or yarn. ...
- 139Imagen on Vertex AI | AI Image Generator | Generative AI on Vertex AI | Google Cloud
https://cloud.google.com/vertex-ai/generative-ai/docs/image/overview
Explore Imagen on Vertex AI, a text-to-image generator that brings Google's image generation AI capabilities to application developers.
- 140Angular + Firebase = ❤️
https://github.com/angular/angularfire
Angular + Firebase = ❤️. Contribute to angular/angularfire development by creating an account on GitHub.
- 141MarkTechson/angular-fundamentals-lessons
https://github.com/MarkTechson/angular-fundamentals-lessons
Contribute to MarkTechson/angular-fundamentals-lessons development by creating an account on GitHub.
- 142🚀 Deploy your 🅰️Angular app to GitHub pages, Cloudflare Pages or any other Git repo directly from the Angular CLI! Available on NPM.
https://github.com/angular-schule/angular-cli-ghpages
🚀 Deploy your 🅰️Angular app to GitHub pages, Cloudflare Pages or any other Git repo directly from the Angular CLI! Available on NPM. - angular-schule/angular-cli-ghpages
- 143Angular & TypeScript Intensiv-Schulung | Inhouse & Remote | workshops.de
https://workshops.de/seminare-schulungen-kurse/angular-typescript/
In 6 Tagen Angular lernen - von den Expert:innen von Workshops.DE und Angular.DE. Auch als Inhouse-Schulung oder Remote buchbar. Jetzt mehr erfahren!
- 144CASL is an isomorphic authorization JavaScript library which restricts what resources a given user is allowed to access
https://github.com/stalniy/casl
CASL is an isomorphic authorization JavaScript library which restricts what resources a given user is allowed to access - stalniy/casl
- 145Angular Copilot & GPTs for AI-Assisted Development Like Angular Copilot (2024)
https://www.whatplugin.ai/gpts/angular-copilot
Chat with Angular Copilot: Your personal Angular assistant and code generator with a focus on responsive, efficient, and scalable UI. Write clean code and become a much faster developer. Explore the best AI-Assisted Development GPTs in ChatGPT!
- 146Easily upgrade your Angular CLI applications from one version to another 🚀
https://github.com/cexbrayat/angular-cli-diff
Easily upgrade your Angular CLI applications from one version to another 🚀 - cexbrayat/angular-cli-diff
- 147A service library for integrate google tag manager in your angular project
https://github.com/mzuccaroli/angular-google-tag-manager
A service library for integrate google tag manager in your angular project - mzuccaroli/angular-google-tag-manager
- 148Matomo analytics client for Angular applications
https://github.com/EmmanuelRoux/ngx-matomo-client
Matomo analytics client for Angular applications. Contribute to EmmanuelRoux/ngx-matomo-client development by creating an account on GitHub.
- 149HackerRank
https://www.hackerrank.com/skills-verification/angular_basic
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews.
- 150Angular Material UI component for firebase authentication
https://github.com/AnthonyNahas/ngx-auth-firebaseui
Angular Material UI component for firebase authentication - AnthonyNahas/ngx-auth-firebaseui
- 151Cryptr Angular SDK
https://github.com/cryptr-auth/cryptr-angular
Cryptr Angular SDK. Contribute to cryptr-auth/cryptr-angular development by creating an account on GitHub.
- 152mgechev/gemini-angular-drawing-demo
https://github.com/mgechev/gemini-angular-drawing-demo
Contribute to mgechev/gemini-angular-drawing-demo development by creating an account on GitHub.
- 153An unofficial project that aims to provide a seamless integration of Clerk features into Angular applications.
https://github.com/anagstef/ngx-clerk
An unofficial project that aims to provide a seamless integration of Clerk features into Angular applications. - anagstef/ngx-clerk
- 154The Complete Course
https://www.udemy.com/course/the-complete-guide-to-angular-2
Master Angular (formerly "Angular 2") and build awesome, reactive web apps with the successor of Angular.js
- 155Serve your Angular CLI project then run a command on top of it
https://github.com/dot-build/angular-serve-and-run
Serve your Angular CLI project then run a command on top of it - dot-build/angular-serve-and-run
- 156angular-builders/packages/jest at master · just-jeb/angular-builders
https://github.com/just-jeb/angular-builders/tree/master/packages/jest
Angular build facade extensions (Jest and custom webpack configuration) - just-jeb/angular-builders
- 157☁️🚀 Deploy your Angular app to Amazon S3 directly from the Angular CLI 🚀☁️
https://github.com/Jefiozie/ngx-aws-deploy
☁️🚀 Deploy your Angular app to Amazon S3 directly from the Angular CLI 🚀☁️ - Jefiozie/ngx-aws-deploy
- 158Code Deck
https://www.youtube.com/@codedeck
Detailed programming tutorials on a broad spectrum of topics
- 159Babatunde Lamidi
https://www.youtube.com/@babatundelmd
Mastering Modern Frontend: Angular, Vue, and beyond. Practical tutorials, coding tips, and tech unboxing. Elevating web development skills one video at a time.
- 160Zoaib Khan
https://www.youtube.com/@ZoaibKhan
I love creating beautiful Angular apps! Join me as I teach you how to be a professional Angular developer by developing real world projects. I will also cover all the new changes coming up to Angular including the new Signals API, Deferrable views and other fun stuff! 🥳 So hit the subscribe button - and let's get coding together!
- 161Esther White {{ MonaCodeLisa }}
https://www.youtube.com/@MonaCodeLisa
Hi there!
- 162Startup Angular
https://www.youtube.com/@StartupAngular
Startup Angularとは? Angularをメインに使っているスタートアップによる、Angular開発の知見などを発信するイベントです。 国内でAngularを使っているスタートアップの知見の共有や情報交換のきっかけ作りとしてイベントを始めました。 Angularに興味がある方、Angularを使っているスタートアップに興味がある方にぴったりです。ぜひご参加ください! 各イベントはこちらで告知しています。 https://voicy.connpass.com/
- 163Angular Supabase components
https://github.com/rustygreen/ng-supabase
Angular Supabase components. Contribute to rustygreen/ng-supabase development by creating an account on GitHub.
- 164UX Trendz
https://www.youtube.com/@uxtrendz
🚀 Welcome to my channel, where coding is my passion! My courses on Angular, RxJS, NgRx, Ngxs, HTML, SCSS, and CSS are designed to help you become a coding superstar. 💥 With real-world examples and practical tips, you'll be able to build amazing apps in no time. 🔔Subscribe now and join our community of like-minded coders!
- 165ng-news
https://www.youtube.com/@ng-news
The latest updates from the Angular community within 100 seconds every week.
- 166Igor Sedov
https://www.youtube.com/@theigorsedov
I'm a full-stack developer with over ten years of experience in web development. I'm dedicated to helping people of all skill levels learn programming in a simple and easy-to-understand way. My focus is on creating step-by-step tutorials with a great visual style that will guide beginners toward becoming adept coders. I'm here to help make learning programming easy and accessible for everyone. Whether you are new to programming or an experienced professional looking to enhance your skills, my channel is the perfect resource. My channel focuses on three key topics: Angular, JavaScript, and TypeScript. Throughout my career, I have held senior positions in technology and telecommunications companies, where I have been responsible for driving company development, technology, and product development. I gained invaluable experience in managing teams, working with suppliers, contractors, and clients. Additionally, I have had the privilege of serving as a CEO and founder of a startup.
- 167Brandon Roberts
https://www.youtube.com/@BrandonRobertsDev
Web Development, Open Source, Angular Brandon is an OSS Advocate, focused on community engagement, content creation, and collaboration. He enjoys learning new things, helping other developers be successful, speaking at conferences, and contributing to open source. He is a GDE, technical writer, and a maintainer of the NgRx project building libraries for reactive Angular applications, and creator of AnalogJS, the fullstack meta-framework for Angular.
- 168Demystify Frontend
https://www.youtube.com/@DemystifyFrontend
"Frontend Insights: Unveiling JS, Angular, React" Join Pardeep on a journey into the core concepts of JavaScript, Angular, and React. Gain deep insights and unravel the intricacies of these technologies. Elevate your frontend skills and discover the secrets behind building robust applications. Let's dive into the world of the front end together!
- 169Angular University
https://www.youtube.com/@AngularUniversity
High quality Angular courses - https://angular-university.io Learn and keep up with the fast moving Angular ecosystem. - Learn the Angular framework from beginner to intermediate or advanced. - Stay up-to-date with the latest developments and features via small bite-sized videos of around 5 minutes. Follow us: Twitter - https://twitter.com/AngularUniv Facebook - https://www.facebook.com/angular.university
- 170angular-builders/packages/custom-webpack at master · just-jeb/angular-builders
https://github.com/just-jeb/angular-builders/tree/master/packages/custom-webpack
Angular build facade extensions (Jest and custom webpack configuration) - just-jeb/angular-builders
- 171Permission and roles based access control for your angular(angular 2,4,5,6,7,9+) applications(AOT, lazy modules compatible
https://github.com/AlexKhymenko/ngx-permissions
Permission and roles based access control for your angular(angular 2,4,5,6,7,9+) applications(AOT, lazy modules compatible - AlexKhymenko/ngx-permissions
- 172Tech Stack Nation
https://www.youtube.com/@techstacknation
Tech Stack Nation is a free, private community for developers and software architects. Formerly Angular Nation, now welcoming devs from all Tech Stacks (including Angular!). Beginners and Introverts are always welcome here! 🥰 🥺 Are you tired of doom scrolling but still interested in tech updates? 🧐 Have you outgrown video tutorials and need something more interactive? 😢 Do you miss conference camaraderie and hallway track networking? 🥰 You've come to the right place!
- 173Angular Japan User Group | Angular 日本ユーザー会
https://www.youtube.com/@ng_japan
Angular日本ユーザー会は日本のAngular開発者によるユーザーコミュニティです! ナレッジの共有やイベントの開催、ドキュメントの翻訳などを中心に活動しています。 https://community.angular.jp
- 174Learning Partner
https://www.youtube.com/@LearningPartnerDigital
Started In 1st Lockdown as wanted to try something different. With great response made it regular thing. Here you will be getting Technologies tutorial videos mainly on Dot Net Mvc, Dot Net Core, Angular - All versions, Html, Css, Jquery and Javascript. All Example will be Realtime and useful in daily developer life. Please reach out if you have anything specific idea for video. All code will be at https://github.com/gerasimitsolutions
- 175Code Shots With Profanis
https://www.youtube.com/@CodeShotsWithProfanis
Hello everybody and welcome to my channel! My name is Fanis Prodromou, I am a Google Developer Expert for Angular and a Senior Front End Developer. In this channel, I am sharing my Angular knowledge for all skill levels.
- 176procademy
https://www.youtube.com/@procademy
I strongly believe that the best way to gain knowledge is by "sharing knowledge" and the best gift you can give is the "Gift of education". My goal is to create online and free quality software courses for everyone. These courses are complete courses just like you expect it from any other eLearning website, but these courses are absolutely free. We want to help those students who have the potential but cannot afford expensive software training. Money should not be a barrier for anyone to learn.
- 177Angular - There's An AI For That
https://theresanaiforthat.com/s/angular/
Browse 63 Angular AIs. Includes tasks such as Angular migration advice, Angular troubleshooting, Angular components, Angular guidance and Angular development assistance.
- 178Angular Master Podcast
https://www.youtube.com/playlist?list=PLYJFRoKhU5SNcu5GBjIn4X3oVpy4fP1wV
Introducing the Angular Master Podcast, your one-stop destination for all things Angular. This podcast is designed for Angular developers at every level, off...
- 179Support for OAuth 2 and OpenId Connect (OIDC) in Angular.
https://github.com/manfredsteyer/angular-oauth2-oidc
Support for OAuth 2 and OpenId Connect (OIDC) in Angular. - GitHub - manfredsteyer/angular-oauth2-oidc: Support for OAuth 2 and OpenId Connect (OIDC) in Angular.
- 180Simon Grimm
https://www.youtube.com/@galaxies_dev
Helping web developers build fantastic mobile apps 🔥 Your weekly dose of web, mobile and cross-platform development with React Native, Ionic, Capacitor, or Flutter. Subscribe for motivating tutorials, projects, and live streams!
- 181Angular
https://www.youtube.com/@Angular
Angular an application development platform that lets you extend HTML vocabulary for your application. The resulting environment is extraordinarily expressive, readable, and quick to develop. For more info, visit http://angular.io
- 182Brian Treese
https://www.youtube.com/@briantreese
Hello my name is Brian Treese and I'm the Chief of UX at SoCreate, a company building a fun and easy way to turn great ideas into movie & TV show scripts. I build things for the web daily and make videos about the stuff I use, discover, or encounter. On this channel, you'll mostly find front-end web development topics about things like HTML, CSS, SCSS, JavaScript, Angular, Accessibility, and more.
- 183Generative AI web development with Angular
https://www.youtube.com/watch?v=5FdtPwZrkGw
Ready to deploy lightning-fast AI apps to the web? Angular v18 brings a set of forward-thinking features to the web, setting new standards for performance an...
- 184Joshua Morony
https://www.youtube.com/@JoshuaMorony
I think the web is cool and like to use it wherever I can. Check out my advanced Ionic tutorials at http://eliteionic.com.
- 185Monsterlessons Academy
https://www.youtube.com/@MonsterlessonsAcademy
Welcome to MonsterlessonsAcademy! Here you will learn programming with focus on web development and frontend development (especially Javasscript). Also if you want to improve your skills as a developer Monsterlessons Academy is the right place for you. My channel covers only the needed and popular technologies like Javascript, Typescript, React, Angular, Vue, Git, Docker, Node JS and much more. If you like my content don't forget to subscribe to the channel!
- 186nivek
https://www.youtube.com/@nivekDev
Coding is more than a job, it's a passion! On this channel, you will learn new cool things about modern frontend technologies.
- 187Nihira Techiees
https://www.youtube.com/@NihiraTechiees
Nihira Techiees providing tutorial for ANGULAR, REACT JS & DOTNET Core etc... #angular #dotnetcore #reactjs #nihiratechiees For contact send me mail at [email protected] Telegram : https://t.me/nihiratechiees
- 188The Code Angle
https://www.youtube.com/@TheCodeAngle
Welcome to The Code Angle. Here we provide online web development and programming tutorials on web technologies like HTML, CSS, JavasScript, React, Vue, Angular, WordPress, Node.js, REST API integration and other Frontend Development, and Backend Development related technologies. Mile Stone / Goals: 100 Subscribers - May 2021 ✔ 500 Subscribers - January 2022 ✔ 1000 Subscribers - May 2022 ✔ 1500 Subscribers - January 2023 ✔ 2000 Subscribers - May 2023 ✔ 2500 Subscribers - December 2023
- 189Deborah Kurata
https://www.youtube.com/@deborah_kurata
Presenting new/interesting features in Angular and basics of Web development (HTML, CSS, JavaScript) with a bit of C# thrown in.
- 190AyyazTech
https://www.youtube.com/@AyyazTech
Welcome to AyyazTech. My name is Ayyaz Zafar. I am a full-stack developer with more than 14 years of professional experience. The purpose of this channel is to share all of my knowledge with you. Angular Course ( Build Blog & CMS / Admin, Node.js, MySQL, Sequelize, Tailwind CSS, Typescript ): https://bit.ly/angular-18-course Please subscribe to my youtube channel to educate yourself. https://www.youtube.com/channel/UCL5nKCmpReJZZMe9_bYR89w?sub_confirmation=1 My Blog Websites: https://ayyazzafar.medium.com https://www.AyyazTech.com Become part of our community on discord: https://discord.com/invite/ABnvB7AF Stores: Gumroad: https://ayyaztech.gumroad.com 🌟 Exclusive Hosting Deal from Hostinger 🌟 Ready to launch your own website? Use my affiliate link to get an exclusive discount on Hostinger's reliable & high-performance hosting plans: https://www.hostg.xyz/SHEyO Hostinger offers: - Easy-to-use control panel - 24/7 customer support - 30-day money-back guarantee - And more!
- 191Rainer Hahnekamp
https://www.youtube.com/@RainerHahnekamp
Welcome to my YouTube channel! I'm Rainer, a full-stack developer from Austria specializing in Angular and Spring. As a Google Developer Expert and a trainer/consultant at AngularArchitects.io, I'm here to share my expertise with you. Subscribe and stay updated with my latest content! Don't forget to connect with me on X (@rainerhahnekamp).
- 192Angular Love
https://www.youtube.com/@angularlove
Polish blog for fans of Angular 2+ framework
- 193Leela Web Dev
https://www.youtube.com/@LeelaWebDev
Hi Friends This channel is all about providing the complete information on Web Development technologies. Please subscribe to my channel: https://youtube.com/c/leelawebdev Support the channel by Donating: PayPal: https://paypal.me/leelanarasimha UPI Id: leelawebdev@ybl
- 194Web Tech Talk
https://www.youtube.com/@WebTechTalk
Welcome to this YouTube channel, your ultimate destination for mastering web development with comprehensive tutorials and courses! Whether you're a beginner or an advanced developer, this channel offers step-by-step guides, full courses, and interview preparation for a wide range of technologies including Angular, JavaScript, CSS, HTML, and React. This channel features: Angular: From beginner tutorials to full courses and advanced interview questions and answers. JavaScript: Start with beginner-friendly tutorials and progress to full courses. Learn the fundamentals, advanced concepts, and prepare for interviews. CSS: Master the art of styling with our CSS tutorials. Perfect for beginners who want to create visually stunning websites. HTML: Begin your web development journey with HTML tutorials and full courses. React: Learn to build powerful user interfaces with React tutorials. #angular #react #javascript #css #html Subscribe now and turn your coding dreams into reality!
- 195ng-conf
https://www.youtube.com/@ngconfonline
The world's original Angular conference, with talks from the Angular Team and top experts from around the world.
- 196Decoded Frontend
https://www.youtube.com/@DecodedFrontend
Decoded Frontend is a source of advanced, in-depth, and pragmatic video tutorials about Angular and Web Development. Every second Tuesday I publish a new video focusing on really advanced topics and tricks that might kick off your carrier and help you to quickly grow from Middle to Senior level. If you already have some experience with the Angular framework and you are bothered by shallow videos made mostly for beginners - then you are at the right place. Consider subscribing to my channel and I promise that you will not be disappointed! My name is Dmytro Mezhenskyi, I am a Google Developer Expert in Angular and I will be happy to share my 12 years of experience in Web Development with you. Thanks for your attention and see you in my next video 👋 For any questions, collaboration or suggestions please reach me at [email protected]
- 197Angular Certification Program
https://altitudecsi.org/products/angular-certification-program
Altitude Career Skills Institute
- 198Angular Mastery Book
https://christianlydemann.com/angular-mastery-book/
Angular Mastery Free Ebook From principles to practice. Accelerate your path to Angular mastery by learning the principles of the industry’s best practices and how you can apply them to your …
- 199List of 300 Angular Interview Questions and answers
https://github.com/sudheerj/angular-interview-questions
List of 300 Angular Interview Questions and answers - sudheerj/angular-interview-questions
- 200GrapesJS - Free and Open Source Web Template Editor Framework
https://grapesjs.com
Free and Open source Web Template Editor - Next generation tool for building templates without coding
- 201Angular - Partytown
https://partytown.builder.io/angular
Run scripts from a web worker.
- 202Scuri Code - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=gparlakov.scuri-code
Extension for Visual Studio Code - Run Scuri from VS Code
- 203Angular Jester - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=michael-fontecchio.angular-jester
Extension for Visual Studio Code - Generate Jest spec files for Angular projects.
- 204Install Tailwind CSS with Angular - Tailwind CSS
https://tailwindcss.com/docs/guides/angular
Setting up Tailwind CSS in an Angular project.
- 205Project IDX
https://idx.dev/
Project IDX is an entirely web-based workspace for full-stack application development, complete with the latest generative AI from Gemini, and full-fidelity app previews, powered by cloud emulators.
- 206vscode-angular-html - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=ghaschel.vscode-angular-html
Extension for Visual Studio Code - Syntax highlighting for angular HTML Template files
- 207Nx Console - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=nrwl.angular-console
Extension for Visual Studio Code - The UI for Nx & Lerna
- 208Angular Testing Library | Testing Library
https://testing-library.com/docs/angular-testing-library/intro/
Angular Testing Library
- 209Angular Schematics - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=cyrilletuzi.angular-schematics
Extension for Visual Studio Code - Ultimate Angular code generation in Visual Studio Code.
- 210Angular 17 Snippets - TypeScript, Html, Angular Material, ngRx, RxJS & Flex Layout - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=Mikael.Angular-BeastCode
Extension for Visual Studio Code - 258 Angular Snippets (TypeScript, Html, Angular Material, Flex Layout, ngRx, RxJS, PWA & Testing)
- 211Analog | Analog
https://analogjs.org/
The fullstack Angular meta-framework
- 212Angular Extension Pack - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=loiane.angular-extension-pack
Extension for Visual Studio Code - Some of the most popular (and some I find very useful) Angular extensions.
- 213Angular
https://www.gooddata.com/blog/frontend-integration-series-angular/
Master GoodData integration with Angular: A step-by-step guide covering installation, environment setup, codebase configuration, and CORS management.
- 214@nx/angular | Nx
https://nx.dev/nx-api/angular
The Nx Plugin for Angular contains executors, generators, and utilities for managing Angular applications and libraries within an Nx workspace. It provides: - Integration with libraries such as Storybook, Jest, ESLint, Tailwind CSS, Playwright and Cypress. - Generators to help scaffold code quickly (like: Micro Frontends, Libraries, both internal to your codebase and publishable to npm) - Single Component Application Modules (SCAMs) - NgRx helpers. - Utilities for automatic workspace refactoring.
- 215Foblex Flow
https://flow.foblex.com/
An Angular library designed to simplify the creation and manipulation of dynamic flow. Provides components for flows, nodes, and connections, automating node manipulation and inter-node connections.
- 216Home
https://ngx-meta.dev
Set your Angular site's metadata: standard meta tags, Open Graph, Twitter Cards, JSON-LD structured data and more. Supports SSR (and Angular Universal). Use a service. Use routes' data. Set it up in a flash! 🚀
- 217StackBlitz | Instant Dev Environments | Click. Code. Done.
https://stackblitz.com
StackBlitz is the collaborative browser-based IDE for web developers. StackBlitz eliminates time-consuming local configuration and lets developers spend more time building.
- 218Easiest JavaScript charting library for web & mobile
https://www.fusioncharts.com
JavaScript charts for web and mobile apps. 95+ chart types, 1400+ maps and 20+ business dashboards with pre-built themes for any business use-case. Build fast, responsive and highly customizable data visualizations trusted by over 28,000 customers and 750,000 developers worldwide...
- 219AutoAnimate - Add motion to your apps with a single line of code
https://auto-animate.formkit.com
A zero-config, drop-in animation utility that automatically adds smooth transitions to your web app. Use it with React, Solid, Vue, Svelte, or any other JavaScript application.
- 220Angular SEO Done Right with Server-Side Rendering [Live Demo]
https://snipcart.com/blog/angular-seo-universal-server-side-rendering
Need SEO-friendly Angular applications? Read this post to learn what is server-side rendering and how to use it on your Angular SPA using Universal. Code repo & live demo included.
- 221Developer security | Snyk
https://snyk.io/
Enable developers to build securely from the start while giving security teams complete visibility and comprehensive controls.
- 222Angular Material
https://material.angular.io/cdk/test-harnesses/overview
UI component infrastructure and Material Design components for Angular web applications.
- 223Testing Angular – A Guide to Robust Angular Applications.
https://testing-angular.com
Unit, integration and end-to-end tests for Angular web applications. Free online book and e-book.
- 224Free Angular Templates - HTMLrev
https://htmlrev.com/free-angular-templates.html
Best free Angular templates for admin dashboards and blogs.
- 225Web & Mobile Templates for Business Software
https://flatlogic.com/templates?framework%5B%5D=angular
Over 25,000 companies worldwide chose our Vue, Angular, React and React Native templates to build their business software faster.
- 226The Content Operating System
https://www.sanity.io/.
Sanity is the modern CMS that transforms content into a competitive advantage. Customize, collaborate, and scale your digital experiences seamlessly.
- 227Nightwatch V3 | Node.js powered End-to-End testing framework
https://nightwatchjs.org/
Write efficient end-to-end tests in Node.js and run them against W3C WebDriver.
- 228ngify/packages/http at main · ngify/ngify
https://github.com/ngify/ngify/tree/main/packages/http
Pretend to be using Angular. Contribute to ngify/ngify development by creating an account on GitHub.
- 229Tailwind CSS Components, Templates and Tools - Tailkit
https://tailkit.com/
Carefully crafted UI Components, Templates and Tools for your next Tailwind CSS based project.
- 23015+ Angular Templates & Themes Admin Dashboards @WrapPixel
https://www.wrappixel.com/templates/category/angular-templates/
Top Angular Templates to create modern Web Applications and Products. Download Free & Premium Angular Templates & Dashboards.
- 231Most complete Angular SAAS starter kit | Full-Stack Boilerplate
https://nzoni.app/
Ship your SAAS project in days with our Angular SAAS Boilerplate. Customizable, scalable, and efficient. Gain over 30 hours of work
- 232GoJS - Interactive Diagrams for the Web in JavaScript and TypeScript
https://gojs.net/latest/index.html
GoJS is a library for building powerful interactive diagrams for every industry. Build apps with flowcharts, org charts, BPMN, UML, modeling, and other visual graph types. Interactivity, data-binding, layouts and many node and link concepts are built-in to GoJS.
- 233Integrations | Sentry for Angular
https://docs.sentry.io/platforms/javascript/guides/angular/configuration/integrations/
Learn more about how integrations extend the functionality of our SDK to cover common libraries and environments automatically.
- 234Angular Integration with Stencil | Stencil
https://stenciljs.com/docs/angular
Learn how to wrap your components so that people can use them natively in Angular
- 235A set of Angular templates for common web apps
https://github.com/hawkgs/angular-templates
A set of Angular templates for common web apps. Contribute to hawkgs/angular-templates development by creating an account on GitHub.
- 236Jspreadsheet with Angular
https://bossanova.uk/jspreadsheet/v4/examples/angular
A full example on how to integrate Jspreadsheet with Angular
- 237A tool for generating code based on a GraphQL schema and GraphQL operations (query/mutation/subscription), with flexible support for custom plugins.
https://github.com/dotansimha/graphql-code-generator
A tool for generating code based on a GraphQL schema and GraphQL operations (query/mutation/subscription), with flexible support for custom plugins. - GitHub - dotansimha/graphql-code-generator: A...
- 238Angular Renderer for THREE.js
https://github.com/angular-threejs/angular-three
Angular Renderer for THREE.js. Contribute to angular-threejs/angular-three development by creating an account on GitHub.
- 239A command line tool to prerender Angular Apps.
https://github.com/chrisguttandin/angular-prerender
A command line tool to prerender Angular Apps. Contribute to chrisguttandin/angular-prerender development by creating an account on GitHub.
- 240Plus Admin Angular | Best Angular Bootstrap Admin Template
https://www.bootstrapdash.com/product/plus-admin-angular
Plus admin angular bootstrap admin template is compatible with Angular-based features and components, facilitating development and making it easier for developers
- 241Angular
https://angular.dev/tools/devtools
The web development framework for building modern apps.
- 242Helper library for handling JWTs in Angular apps
https://github.com/auth0/angular2-jwt
Helper library for handling JWTs in Angular apps. Contribute to auth0/angular2-jwt development by creating an account on GitHub.
- 243Papa Parse wrapper for Angular
https://github.com/alberthaff/ngx-papaparse
Papa Parse wrapper for Angular. Contribute to alberthaff/ngx-papaparse development by creating an account on GitHub.
- 244MaterialPro is the Most Powerful & Comprehensive free Angular admin template based on Material Angular !! 🚀
https://github.com/wrappixel/material-pro-angular-lite
MaterialPro is the Most Powerful & Comprehensive free Angular admin template based on Material Angular !! 🚀 - wrappixel/material-pro-angular-lite
- 245Extract and merge i18n xliff translation files for angular projects.
https://github.com/daniel-sc/ng-extract-i18n-merge
Extract and merge i18n xliff translation files for angular projects. - daniel-sc/ng-extract-i18n-merge
- 246ASP.NET Core / Angular startup project template with complete login, user and role management. Plus other useful services for Quick Application Development
https://github.com/emonney/QuickApp
ASP.NET Core / Angular startup project template with complete login, user and role management. Plus other useful services for Quick Application Development - emonney/QuickApp
- 247Gradient able free bootstrap, angular, react admin template
https://github.com/codedthemes/gradient-able-free-admin-template
Gradient able free bootstrap, angular, react admin template - codedthemes/gradient-able-free-admin-template
- 248Xtreme is the Most Powerful & Comprehensive free Angular admin template based on Bootstrap Angular !! 🚀
https://github.com/wrappixel/xtreme-admin-angular-lite
Xtreme is the Most Powerful & Comprehensive free Angular admin template based on Bootstrap Angular !! 🚀 - wrappixel/xtreme-admin-angular-lite
- 249Google Pay button - React, Angular, and custom element
https://github.com/google-pay/google-pay-button
Google Pay button - React, Angular, and custom element - google-pay/google-pay-button
- 250Mixalloff/ngx-http-rest
https://github.com/Mixalloff/ngx-http-rest
Contribute to Mixalloff/ngx-http-rest development by creating an account on GitHub.
- 251Angular shareable config for Renovate
https://github.com/d-koppenhagen/renovate-config-angular
Angular shareable config for Renovate. Contribute to d-koppenhagen/renovate-config-angular development by creating an account on GitHub.
- 252A bare-bones Angular template to get you deployed to Netlify fast!
https://github.com/netlify-templates/angular-quickstart
A bare-bones Angular template to get you deployed to Netlify fast! - netlify-templates/angular-quickstart
- 253A light slider with no external dependencies
https://github.com/Jaspero/ng-slider
A light slider with no external dependencies. Contribute to Jaspero/ng-slider development by creating an account on GitHub.
- 254Ionic for JHipster ✨
https://github.com/jhipster/generator-jhipster-ionic
Ionic for JHipster ✨. Contribute to jhipster/generator-jhipster-ionic development by creating an account on GitHub.
- 255Angular & Tailwind CSS Admin Dashboard Starter Kit, Free and Open Source
https://github.com/lannodev/angular-tailwind
Angular & Tailwind CSS Admin Dashboard Starter Kit, Free and Open Source - lannodev/angular-tailwind
- 256Scaffold an Angular project with all tooling you need for production projects
https://github.com/EPAM-JS-Competency-center/angular-scaffold
Scaffold an Angular project with all tooling you need for production projects - EPAM-JS-Competency-center/angular-scaffold
- 257A Phaser 3 TypeScript project template that uses the Angular framework and Vite for bundling
https://github.com/phaserjs/template-angular
A Phaser 3 TypeScript project template that uses the Angular framework and Vite for bundling - phaserjs/template-angular
- 258AngularTools is a collection of tools for exploring a Angular project, help you with documenting, reverse engineering a project or help when refactoring.
https://github.com/CoderAllan/vscode-angulartools
AngularTools is a collection of tools for exploring a Angular project, help you with documenting, reverse engineering a project or help when refactoring. - CoderAllan/vscode-angulartools
- 259Angular and NestJS Monorepo setup with npm Workspaces and a Github Actions
https://github.com/JangoCG/angular-nestjs-starter-kit
Angular and NestJS Monorepo setup with npm Workspaces and a Github Actions - JangoCG/angular-nestjs-starter-kit
- 260Contains pipes to transform internationalization data using Intl.* browser APIs
https://github.com/json-derulo/angular-ecmascript-intl
Contains pipes to transform internationalization data using Intl.* browser APIs - json-derulo/angular-ecmascript-intl
- 261A set of Angular components to manage GoJS Diagrams, Palettes, and Overviews
https://github.com/NorthwoodsSoftware/gojs-angular
A set of Angular components to manage GoJS Diagrams, Palettes, and Overviews - NorthwoodsSoftware/gojs-angular
- 262A small library to convert RxJs Observables into Angular Signals
https://github.com/alcfeoh/ngx-signalify
A small library to convert RxJs Observables into Angular Signals - alcfeoh/ngx-signalify
- 263A generated wails template, currently support Angular 17.
https://github.com/mJehanno/wails-template-angular-latest
A generated wails template, currently support Angular 17. - mJehanno/wails-template-angular-latest
- 264Angular 6+ wrapper for StripeJS
https://github.com/richnologies/ngx-stripe
Angular 6+ wrapper for StripeJS. Contribute to richnologies/ngx-stripe development by creating an account on GitHub.
- 265:notebook_with_decorative_cover: The missing documentation tool for your Angular, Nest & Stencil application
https://github.com/compodoc/compodoc
:notebook_with_decorative_cover: The missing documentation tool for your Angular, Nest & Stencil application - compodoc/compodoc
- 266Karma jasmine matcher that performs image comparisons based on jest-image-snapshot for visual regression testing
https://github.com/maksimr/karma-image-snapshot
Karma jasmine matcher that performs image comparisons based on jest-image-snapshot for visual regression testing - maksimr/karma-image-snapshot
- 26715 Free Angular Templates For Your Admin 2024
https://colorlib.com/wp/free-angular-templates/
With these outstanding, versatile and resourceful free Angular templates, you can speed up the process of building a powerful admin.
- 268Angular wrapper library for GrapesJS
https://github.com/Developer-Plexscape/ngx-grapesjs
Angular wrapper library for GrapesJS. Contribute to Developer-Plexscape/ngx-grapesjs development by creating an account on GitHub.
- 269The Ultimate Showdown | Tailcall
https://tailcall.run/blog/graphql-angular-client/
We pushed each method to its limits. Here's what we discovered.
- 270📖 A modern, powerful and out of the box documentation generator for Angular components lib and markdown docs.(现代化的、强大的、开箱即用的 Angular 组件文档生成工具)
https://github.com/docgeni/docgeni
📖 A modern, powerful and out of the box documentation generator for Angular components lib and markdown docs.(现代化的、强大的、开箱即用的 Angular 组件文档生成工具) - docgeni/docgeni
- 271Angular Material admin template.
https://github.com/ng-matero/ng-matero
Angular Material admin template. Contribute to ng-matero/ng-matero development by creating an account on GitHub.
- 272This is a basic template project to start with Angular 17.x and ThreeJS
https://github.com/JohnnyDevNull/ng-three-template
This is a basic template project to start with Angular 17.x and ThreeJS - JohnnyDevNull/ng-three-template
- 273This is a generic sample app, built in Angular, that showcases Descope Authentication using the WebJS SDK.
https://github.com/descope-sample-apps/angular-sample-app
This is a generic sample app, built in Angular, that showcases Descope Authentication using the WebJS SDK. - descope-sample-apps/angular-sample-app
- 274Modular HTTP extensions for Angular.
https://github.com/jscutlery/convoyr
Modular HTTP extensions for Angular. Contribute to jscutlery/convoyr development by creating an account on GitHub.
- 275Flexy is the Most Powerful & Comprehensive free Angular admin template based on Material Angular !! 🚀
https://github.com/wrappixel/Flexy-admin-angular-lite
Flexy is the Most Powerful & Comprehensive free Angular admin template based on Material Angular !! 🚀 - wrappixel/Flexy-admin-angular-lite
- 276A Native Angular 2+ Typing Animation Library , Angular SSR , Angular Universal Friendly
https://github.com/SkyZeroZx/ngx-typed-writer
A Native Angular 2+ Typing Animation Library , Angular SSR , Angular Universal Friendly - SkyZeroZx/ngx-typed-writer
- 277Angular 18 & React 18 Examples SEO (Search engine optimization)
https://github.com/ganatan/angular-react-seo
Angular 18 & React 18 Examples SEO (Search engine optimization) - ganatan/angular-react-seo
- 278Smart Monorepos · Fast CI
https://github.com/nrwl/nx
Smart Monorepos · Fast CI. Contribute to nrwl/nx development by creating an account on GitHub.
- 279Postcat 是一个可扩展的 API 工具平台。集合基础的 API 管理和测试功能,并且可以通过插件简化你的 API 开发工作,让你可以更快更好地创建 API。An extensible API tool.
https://github.com/Postcatlab/postcat
Postcat 是一个可扩展的 API 工具平台。集合基础的 API 管理和测试功能,并且可以通过插件简化你的 API 开发工作,让你可以更快更好地创建 API。An extensible API tool. - Postcatlab/postcat
- 280Angular Snippets for VS Code
https://github.com/johnpapa/vscode-angular-snippets
Angular Snippets for VS Code. Contribute to johnpapa/vscode-angular-snippets development by creating an account on GitHub.
- 281A lightweight responsive Angular carousel library
https://github.com/bfwg/ngx-drag-scroll
A lightweight responsive Angular carousel library. Contribute to bfwg/ngx-drag-scroll development by creating an account on GitHub.
- 282🛠️ Provides high-level utilities and reduces boilerplate for testing Angular applications
https://github.com/remscodes/ngx-testing-tools
🛠️ Provides high-level utilities and reduces boilerplate for testing Angular applications - remscodes/ngx-testing-tools
- 283A repo with guides and examples for unit tests in Angular applications using ng-mocks.
https://github.com/help-me-mom/ng-mocks-sandbox
A repo with guides and examples for unit tests in Angular applications using ng-mocks. - help-me-mom/ng-mocks-sandbox
- 284Kwerri OSS: samvloeberghs.be + ngx-seo
https://github.com/samvloeberghs/kwerri-oss/tree/main
Kwerri OSS: samvloeberghs.be + ngx-seo. Contribute to samvloeberghs/kwerri-oss development by creating an account on GitHub.
- 285Responsive Application Layout Templates based on DevExtreme Angular Components
https://github.com/DevExpress/devextreme-angular-template
Responsive Application Layout Templates based on DevExtreme Angular Components - DevExpress/devextreme-angular-template
- 286The library for analyze a HTML file to show all of the SEO defects
https://github.com/maddevsio/seo-analyzer
The library for analyze a HTML file to show all of the SEO defects - maddevsio/seo-analyzer
- 287Spike is the Most Powerful & Comprehensive free Angular admin template based on Material Angular !! 🚀
https://github.com/wrappixel/spike-angular-free
Spike is the Most Powerful & Comprehensive free Angular admin template based on Material Angular !! 🚀 - wrappixel/spike-angular-free
- 288Angular 17+ wrapper for slick plugin
https://github.com/leo6104/ngx-slick-carousel
Angular 17+ wrapper for slick plugin . Contribute to leo6104/ngx-slick-carousel development by creating an account on GitHub.
- 289Nice is the Most Powerful & Comprehensive free Angular admin template based on Bootstrap Angular !! 🚀
https://github.com/wrappixel/nice-angular-lite
Nice is the Most Powerful & Comprehensive free Angular admin template based on Bootstrap Angular !! 🚀 - wrappixel/nice-angular-lite
- 290Simple web app template with Angular v18 + NestJS v10 + ng-openapi-gen / Deployable on Heroku, Render.com, Google App Engine and others (incl. Docker) / CI with GitHub Actions / Public Domain
https://github.com/mugifly/angular-nest
Simple web app template with Angular v18 + NestJS v10 + ng-openapi-gen / Deployable on Heroku, Render.com, Google App Engine and others (incl. Docker) / CI with GitHub Actions / Public Domain - mug...
- 291A collection of code generators for modern JavaScript applications
https://github.com/teleporthq/teleport-code-generators
A collection of code generators for modern JavaScript applications - teleporthq/teleport-code-generators
- 292✨ Jest matcher for image comparisons. Most commonly used for visual regression testing.
https://github.com/americanexpress/jest-image-snapshot
✨ Jest matcher for image comparisons. Most commonly used for visual regression testing. - americanexpress/jest-image-snapshot
- 293Disqus for Angular 💬
https://github.com/MurhafSousli/ngx-disqus
Disqus for Angular 💬. Contribute to MurhafSousli/ngx-disqus development by creating an account on GitHub.
- 294This repository contains a plugin for the Angular framework, that integrates with Split JS SDK and offers an integrated experience with the Angular framework
https://github.com/splitio/angular-sdk-plugin
This repository contains a plugin for the Angular framework, that integrates with Split JS SDK and offers an integrated experience with the Angular framework - splitio/angular-sdk-plugin
- 295Client side OData typescript library for Angular
https://github.com/diegomvh/angular-odata
Client side OData typescript library for Angular. Contribute to diegomvh/angular-odata development by creating an account on GitHub.
- 296🚀 😍 The internationalization (i18n) library for Angular
https://github.com/jsverse/transloco/
🚀 😍 The internationalization (i18n) library for Angular - jsverse/transloco
- 297ZingGrid - A fully-featured, native, web-component, data table and data grid for Javascript applications.
https://github.com/ZingGrid/zinggrid
ZingGrid - A fully-featured, native, web-component, data table and data grid for Javascript applications. - ZingGrid/zinggrid
- 298A loader for ngx-translate that loads translations with http calls
https://github.com/rbalet/ngx-translate-multi-http-loader
A loader for ngx-translate that loads translations with http calls - rbalet/ngx-translate-multi-http-loader
- 299Rapidly scaffold out a new tauri app project.
https://github.com/tauri-apps/create-tauri-app
Rapidly scaffold out a new tauri app project. Contribute to tauri-apps/create-tauri-app development by creating an account on GitHub.
- 300Pure Angular 2+ date range picker with material design theme, a demo here:
https://github.com/fetrarij/ngx-daterangepicker-material
Pure Angular 2+ date range picker with material design theme, a demo here: - GitHub - fetrarij/ngx-daterangepicker-material: Pure Angular 2+ date range picker with material design theme, a demo here:
- 301:bar_chart: Declarative Charting Framework for Angular
https://github.com/swimlane/ngx-charts
:bar_chart: Declarative Charting Framework for Angular - swimlane/ngx-charts
- 302oOps1627/angular-calendar-timeline
https://github.com/oOps1627/angular-calendar-timeline
Contribute to oOps1627/angular-calendar-timeline development by creating an account on GitHub.
- 303Monster is the Most Powerful & Comprehensive free Angular admin template based on Bootstrap Angular !! 🚀
https://github.com/wrappixel/monster-angular-lite
Monster is the Most Powerful & Comprehensive free Angular admin template based on Bootstrap Angular !! 🚀 - wrappixel/monster-angular-lite
- 304Codemods for migrating to Jest https://github.com/facebook/jest 👾
https://github.com/skovhus/jest-codemods
Codemods for migrating to Jest https://github.com/facebook/jest 👾 - skovhus/jest-codemods
- 305Socket.IO module for Angular
https://github.com/rodgc/ngx-socket-io
Socket.IO module for Angular. Contribute to rodgc/ngx-socket-io development by creating an account on GitHub.
- 306The Angular-V17-Template is a template designed for Angular 17 that emphasizes clean code practices and test-friendly development.
https://github.com/GabrielToth/Angular-V17-Template
The Angular-V17-Template is a template designed for Angular 17 that emphasizes clean code practices and test-friendly development. - GabrielToth/Angular-V17-Template
- 307Angular library for injecting JWT tokens to HTTP requests.
https://github.com/rars/ngx-jwt
Angular library for injecting JWT tokens to HTTP requests. - rars/ngx-jwt
- 308🅰 AnguHashBlog DEMOs and Documentation
https://github.com/AnguHashBlog/anguhashblog
🅰 AnguHashBlog DEMOs and Documentation. Contribute to AnguHashBlog/anguhashblog development by creating an account on GitHub.
- 309A simple starter app for bootstrapping applications with Carbon
https://github.com/carbon-design-system/carbon-angular-starter
A simple starter app for bootstrapping applications with Carbon - carbon-design-system/carbon-angular-starter
- 310ngworker/packages/spectacular at main · ngworker/ngworker
https://github.com/ngworker/ngworker/tree/main/packages/spectacular
Monorepo for the @ngworker NPM organization. Packages for Angular applications and testing. - ngworker/ngworker
- 311High-Performance React Grid, Angular Grid, JavaScript Grid
https://www.ag-grid.com/
AG Grid is a feature-rich datagrid for major JavaScript frameworks, offering filtering, grouping, pivoting, and more. Free Community version or 2-month Enterprise trial available.
- 312Angular
https://angular.dev/best-practices/security
The web development framework for building modern apps.
- 313Angular6+ Unit Test Generator For Components, Directive, Services, and Pipes
https://github.com/allenhwkim/ngentest
Angular6+ Unit Test Generator For Components, Directive, Services, and Pipes - allenhwkim/ngentest
- 314Real world GraphQL tutorials for frontend developers with deadlines!
https://github.com/hasura/learn-graphql
Real world GraphQL tutorials for frontend developers with deadlines! - hasura/learn-graphql
- 315Angular flow diagram library
https://github.com/uiuniversal/ngu-flow
Angular flow diagram library. Contribute to uiuniversal/ngu-flow development by creating an account on GitHub.
- 316Angular Starter Kit for using Tailkit UI components out of the box in your project.
https://github.com/pixelcave/tailkit-starter-kit-angular
Angular Starter Kit for using Tailkit UI components out of the box in your project. - pixelcave/tailkit-starter-kit-angular
- 317tolgee-js/packages/ngx/projects/ngx-tolgee at main · tolgee/tolgee-js
https://github.com/tolgee/tolgee-js/tree/main/packages/ngx/projects/ngx-tolgee
Tolgee JavaScript libraries monorepo. Contribute to tolgee/tolgee-js development by creating an account on GitHub.
- 318Official CKEditor 4 Angular component.
https://github.com/ckeditor/ckeditor4-angular
Official CKEditor 4 Angular component. Contribute to ckeditor/ckeditor4-angular development by creating an account on GitHub.
- 319Ample is the Most Powerful & Comprehensive free Angular admin template based on Bootstrap Angular !! 🚀
https://github.com/wrappixel/ample-angular-lite
Ample is the Most Powerful & Comprehensive free Angular admin template based on Bootstrap Angular !! 🚀 - wrappixel/ample-angular-lite
- 320Getting Started with Datadog
https://docs.datadoghq.com/integrations/rum_angular/
Datadog, the leading service for cloud-scale monitoring.
- 321The next-generation AngularDart documentation website.
https://github.com/angulardart-community/website
The next-generation AngularDart documentation website. - angulardart-community/website
- 322Framework agnostic table component for editable data experience.
https://github.com/OvidijusParsiunas/active-table
Framework agnostic table component for editable data experience. - OvidijusParsiunas/active-table
- 323🚀 Angular project template with Prettier, Linter, Git-Hooks and VS Code settings
https://github.com/svierk/angular-starter-kit
🚀 Angular project template with Prettier, Linter, Git-Hooks and VS Code settings - svierk/angular-starter-kit
- 324Angular component to add rough notation animation when element is visible.
https://github.com/namitoyokota/ngx-notation-reveal
Angular component to add rough notation animation when element is visible. - namitoyokota/ngx-notation-reveal
- 325Angular TanStack table with shadcn Theme
https://github.com/radix-ng/datagrid
Angular TanStack table with shadcn Theme. Contribute to radix-ng/datagrid development by creating an account on GitHub.
- 326Ultra-fast bootstrapping with Angular and Electron :speedboat:
https://github.com/maximegris/angular-electron
Ultra-fast bootstrapping with Angular and Electron :speedboat: - maximegris/angular-electron
- 327Ultra-fast bootstrapping with Angular and Tauri :speedboat:
https://github.com/maximegris/angular-tauri
Ultra-fast bootstrapping with Angular and Tauri :speedboat: - maximegris/angular-tauri
- 328A powerful and performant Angular year calendar library built with ❤️
https://github.com/IOMechs/angular-year-calendar
A powerful and performant Angular year calendar library built with ❤️ - IOMechs/angular-year-calendar
- 329manudss/ngx-http-annotations
https://github.com/manudss/ngx-http-annotations
Contribute to manudss/ngx-http-annotations development by creating an account on GitHub.
- 330Angular library to translate texts, dates and numbers
https://github.com/robisim74/angular-l10n
Angular library to translate texts, dates and numbers - robisim74/angular-l10n
- 331The Easiest Angular Table. 12kb gzipped! Tree-shakeable. 55 features and growing!
https://github.com/ssuperczynski/ngx-easy-table
The Easiest Angular Table. 12kb gzipped! Tree-shakeable. 55 features and growing! - ssuperczynski/ngx-easy-table
- 332:telephone: Angular module for intl-tel-input integration (https://github.com/jackocnr/intl-tel-input)
https://github.com/mpalourdio/intl-tel-input-ng
:telephone: Angular module for intl-tel-input integration (https://github.com/jackocnr/intl-tel-input) - mpalourdio/intl-tel-input-ng
- 333A clean Angular SPA that gets content from Sanity
https://github.com/sanity-io/sanity-template-angular-clean
A clean Angular SPA that gets content from Sanity. Contribute to sanity-io/sanity-template-angular-clean development by creating an account on GitHub.
- 334Angular lib for identify which time is associated which blockchain block in the past (and format it)
https://github.com/antonioconselheiro/time2blocks-ngx
Angular lib for identify which time is associated which blockchain block in the past (and format it) - antonioconselheiro/time2blocks-ngx
- 335🔥 Angular HTTP cache interceptor
https://github.com/patrikx3/angular-http-cache-interceptor
🔥 Angular HTTP cache interceptor. Contribute to patrikx3/angular-http-cache-interceptor development by creating an account on GitHub.
- 336A lightning fast JavaScript grid/spreadsheet
https://github.com/mleibman/SlickGrid
A lightning fast JavaScript grid/spreadsheet. Contribute to mleibman/SlickGrid development by creating an account on GitHub.
- 337:dango: Smart angular HTTP interceptor - Intercepts automagically HTTP requests and shows a spinkit spinner / loader / progress bar
https://github.com/mpalourdio/ng-http-loader
:dango: Smart angular HTTP interceptor - Intercepts automagically HTTP requests and shows a spinkit spinner / loader / progress bar - mpalourdio/ng-http-loader
- 338devkit/packages/cypress-harness at main · jscutlery/devkit
https://github.com/jscutlery/devkit/tree/main/packages/cypress-harness
Tools that make Angular developer's life easier. Contribute to jscutlery/devkit development by creating an account on GitHub.
- 339Free Angular Admin Template by PrimeNG
https://github.com/primefaces/sakai-ng
Free Angular Admin Template by PrimeNG. Contribute to primefaces/sakai-ng development by creating an account on GitHub.
- 340Localess is a powerful translation management tool and content management system built using Angular and Firebase. With Localess, you can easily manage and translate your website or app content into multiple languages, and it uses Artificial Intelligence to translate faster.
https://github.com/Lessify/localess
Localess is a powerful translation management tool and content management system built using Angular and Firebase. With Localess, you can easily manage and translate your website or app content int...
- 341Modular data visualization framework for React, Angular, Svelte, Vue, and vanilla TypeScript or JavaScript
https://github.com/f5/unovis
Modular data visualization framework for React, Angular, Svelte, Vue, and vanilla TypeScript or JavaScript - f5/unovis
- 342📦 🔍 👀 A comparison tool to compare JavaScript data grid and spreadsheet libraries
https://github.com/statico/jsgrids
📦 🔍 👀 A comparison tool to compare JavaScript data grid and spreadsheet libraries - statico/jsgrids
- 343Gentleman-Programming/Angular-18-boilerplate
https://github.com/Gentleman-Programming/Angular-18-boilerplate
Contribute to Gentleman-Programming/Angular-18-boilerplate development by creating an account on GitHub.
- 344Angular NgRx powered frontend template for Symfony (or similar) backend
https://github.com/tarlepp/angular-ngrx-frontend
Angular NgRx powered frontend template for Symfony (or similar) backend - tarlepp/angular-ngrx-frontend
- 345🧸 A resource to showcase the different animations that you could do with Angular
https://github.com/williamjuan027/angular-animations-explorer
🧸 A resource to showcase the different animations that you could do with Angular - williamjuan027/angular-animations-explorer
- 346NGCC has been removed in Angular 16. Quickly check which dependencies stop you from upgrading!
https://github.com/danielglejzner/ng16-dep-audit
NGCC has been removed in Angular 16. Quickly check which dependencies stop you from upgrading! - danielglejzner/ng16-dep-audit
- 347Angular
https://angular.dev/cli/update
The web development framework for building modern apps.
- 348a light carousel for Angular18+, support mobile touch by hammerJs
https://github.com/ZouYouShun/ngx-hm-carousel
a light carousel for Angular18+, support mobile touch by hammerJs - ZouYouShun/ngx-hm-carousel
- 349Splide.js integration to angular
https://github.com/JustCommunication-ru/ngx-splide
Splide.js integration to angular. Contribute to JustCommunication-ru/ngx-splide development by creating an account on GitHub.
- 350Angular library containing components used for handling datetime
https://github.com/ressurectit/ng-datetime
Angular library containing components used for handling datetime - ressurectit/ng-datetime
- 351JavaScript event calendar. Modern alternative to fullcalendar and react-big-calendar.
https://github.com/schedule-x/schedule-x
JavaScript event calendar. Modern alternative to fullcalendar and react-big-calendar. - schedule-x/schedule-x
- 352Debug your Jest tests. Effortlessly.🛠🖼
https://github.com/nvh95/jest-preview
Debug your Jest tests. Effortlessly.🛠🖼 . Contribute to nvh95/jest-preview development by creating an account on GitHub.
- 353Shiki Magic Move example with Angular
https://github.com/tutkli/angular-shiki-magic-move
Shiki Magic Move example with Angular. Contribute to tutkli/angular-shiki-magic-move development by creating an account on GitHub.
- 354Image Gallery built with Angular 18+, node.js and GraphicsMagick
https://github.com/BenjaminBrandmeier/angular2-image-gallery
Image Gallery built with Angular 18+, node.js and GraphicsMagick - BenjaminBrandmeier/angular2-image-gallery
- 355Nx Workspace with all outdated & unmaintained Angular libs that are stuck using ViewEngine. Let's make them work with Angular 16+ !
https://github.com/danielglejzner/ngx-maintenance
Nx Workspace with all outdated & unmaintained Angular libs that are stuck using ViewEngine. Let's make them work with Angular 16+ ! - GitHub - danielglejzner/ngx-maintenance: Nx Workspace ...
- 356An Angular Typing Animation Library.
https://github.com/shiv-source/ngx-typed2
An Angular Typing Animation Library. Contribute to shiv-source/ngx-typed2 development by creating an account on GitHub.
- 357I18N Library for .NET, and Delphi
https://github.com/soluling/I18N
I18N Library for .NET, and Delphi. Contribute to soluling/I18N development by creating an account on GitHub.
- 358Highly customizable org chart. Integrations available for Angular, React, Vue
https://github.com/bumbeishvili/org-chart
Highly customizable org chart. Integrations available for Angular, React, Vue - bumbeishvili/org-chart
- 359Ngx Facebook Messenger offers a lightweight alternative, optimizing user experiences by significantly reducing the initial load size, ensuring smoother page performance, and enhancing overall website efficiency
https://github.com/SkyZeroZx/ngx-facebook-messenger
Ngx Facebook Messenger offers a lightweight alternative, optimizing user experiences by significantly reducing the initial load size, ensuring smoother page performance, and enhancing overall websi...
- 360Create automatic spies from classes
https://github.com/hirezio/auto-spies
Create automatic spies from classes. Contribute to hirezio/auto-spies development by creating an account on GitHub.
- 361Angular Gallery, Carousel and Lightbox
https://github.com/MurhafSousli/ngx-gallery
Angular Gallery, Carousel and Lightbox. Contribute to MurhafSousli/ngx-gallery development by creating an account on GitHub.
- 362The HTML touch slider carousel with the most native feeling you will get.
https://github.com/rcbyr/keen-slider
The HTML touch slider carousel with the most native feeling you will get. - rcbyr/keen-slider
- 363The official Angular component for FullCalendar
https://github.com/fullcalendar/fullcalendar-angular
The official Angular component for FullCalendar. Contribute to fullcalendar/fullcalendar-angular development by creating an account on GitHub.
- 364Systelab Angular Chart services
https://github.com/systelab/systelab-charts
Systelab Angular Chart services. Contribute to systelab/systelab-charts development by creating an account on GitHub.
- 365Angular 2+ / Ionic 2+ HTML/table element to export it as JSON, XML, PNG, CSV, TXT, MS-Word, Ms-Excel, PDF
https://github.com/wnabil/ngx-export-as
Angular 2+ / Ionic 2+ HTML/table element to export it as JSON, XML, PNG, CSV, TXT, MS-Word, Ms-Excel, PDF - wnabil/ngx-export-as
- 366Angular component for Chartist.js
https://github.com/willsoto/ng-chartist
Angular component for Chartist.js. Contribute to willsoto/ng-chartist development by creating an account on GitHub.
- 367devkit/packages/swc-angular-plugin at main · jscutlery/devkit
https://github.com/jscutlery/devkit/tree/main/packages/swc-angular-plugin
Tools that make Angular developer's life easier. Contribute to jscutlery/devkit development by creating an account on GitHub.
- 368A GraphQL client for Angular built on top of Apollo.
https://github.com/buoy-graphql/buoy
A GraphQL client for Angular built on top of Apollo. - buoy-graphql/buoy
- 369📜 Angular 11.1+ JSON Translations Editor.
https://github.com/navix/ngxe
📜 Angular 11.1+ JSON Translations Editor. Contribute to navix/ngxe development by creating an account on GitHub.
- 370Mutation testing for JavaScript and friends
https://github.com/stryker-mutator/stryker-js
Mutation testing for JavaScript and friends. Contribute to stryker-mutator/stryker-js development by creating an account on GitHub.
- 371Material timepicker based on material design
https://github.com/dhutaryan/ngx-mat-timepicker
Material timepicker based on material design. Contribute to dhutaryan/ngx-mat-timepicker development by creating an account on GitHub.
- 372Angular Table Component
https://github.com/panemu/ngx-panemu-table
Angular Table Component. Contribute to panemu/ngx-panemu-table development by creating an account on GitHub.
- 373Confetti in Angular
https://github.com/ChellappanRajan/ngx-confetti-explosion
Confetti in Angular. Contribute to ChellappanRajan/ngx-confetti-explosion development by creating an account on GitHub.
- 374Angular
https://angular.dev/update-guide
The web development framework for building modern apps.
- 375Angular
https://angular.dev/reference/migrations
The web development framework for building modern apps.
- 376Fully customizable Angular component for rendering After Effects animations. Compatible with Angular 9+ :rocket:
https://github.com/ngx-lottie/ngx-lottie
Fully customizable Angular component for rendering After Effects animations. Compatible with Angular 9+ :rocket: - ngx-lottie/ngx-lottie
- 377Angular Template App with a Router-first architecture
https://github.com/duluca/lemon-mart
Angular Template App with a Router-first architecture - duluca/lemon-mart
- 378A fully-featured, production ready caching GraphQL client for Angular and every GraphQL server 🎁
https://github.com/kamilkisiela/apollo-angular
A fully-featured, production ready caching GraphQL client for Angular and every GraphQL server 🎁 - kamilkisiela/apollo-angular
- 379Free Angular admin template for Berry design
https://github.com/codedthemes/berry-free-angular-admin-template
Free Angular admin template for Berry design. Contribute to codedthemes/berry-free-angular-admin-template development by creating an account on GitHub.
- 380A modern and powerful gantt chart component for Angular
https://github.com/worktile/ngx-gantt
A modern and powerful gantt chart component for Angular - worktile/ngx-gantt
- 381A Github @actions that keeps your @angular CLI-based projects up-to-date via automated PRs based on `ng update`.
https://github.com/fast-facts/ng-update
A Github @actions that keeps your @angular CLI-based projects up-to-date via automated PRs based on `ng update`. - fast-facts/ng-update
- 382Angular extension for Visual Studio Code
https://github.com/angular/vscode-ng-language-service
Angular extension for Visual Studio Code. Contribute to angular/vscode-ng-language-service development by creating an account on GitHub.
- 383carbon-charts/packages/angular at master · carbon-design-system/carbon-charts
https://github.com/carbon-design-system/carbon-charts/tree/master/packages/angular
:bar_chart: :chart_with_upwards_trend:⠀Robust dataviz framework implemented using D3 & typescript - carbon-design-system/carbon-charts
- 384Open-source web application framework for ASP.NET Core! Offers an opinionated architecture to build enterprise software solutions with best practices on top of the .NET. Provides the fundamental infrastructure, cross-cutting-concern implementations, startup templates, application modules, UI themes, tooling and documentation.
https://github.com/abpframework/abp
Open-source web application framework for ASP.NET Core! Offers an opinionated architecture to build enterprise software solutions with best practices on top of the .NET. Provides the fundamental in...
- 385Self-contained, mobile friendly slider component for Angular based on angularjs-slider
https://github.com/angular-slider/ngx-slider
Self-contained, mobile friendly slider component for Angular based on angularjs-slider - angular-slider/ngx-slider
- 386AdminPro is the Most Powerful & Comprehensive free Angular admin template based on Bootstrap Angular !! 🚀
https://github.com/wrappixel/admin-pro-angular-lite
AdminPro is the Most Powerful & Comprehensive free Angular admin template based on Bootstrap Angular !! 🚀 - wrappixel/admin-pro-angular-lite
- 387CoreUI Angular is free Angular 18 admin template based on Bootstrap 5
https://github.com/coreui/coreui-free-angular-admin-template
CoreUI Angular is free Angular 18 admin template based on Bootstrap 5 - coreui/coreui-free-angular-admin-template
- 388Helpers library for marbles testing with Jest
https://github.com/just-jeb/jest-marbles
Helpers library for marbles testing with Jest. Contribute to just-jeb/jest-marbles development by creating an account on GitHub.
- 389Code mutations in your project or schematics were never easier than now.
https://github.com/taiga-family/ng-morph
Code mutations in your project or schematics were never easier than now. - taiga-family/ng-morph
- 390Playwright Angular component testing.
https://github.com/sand4rt/playwright-ct-angular
Playwright Angular component testing. Contribute to sand4rt/playwright-ct-angular development by creating an account on GitHub.
- 391date pipes for Angular
https://github.com/dstelljes/luxon-angular
date pipes for Angular. Contribute to dstelljes/luxon-angular development by creating an account on GitHub.
- 392@ngaox/seo
https://www.npmjs.com/package/@ngaox/seo
Easily generate and manage SEO-friendly meta tags, page title,.... Latest version: 6.0.0-beta-2, last published: 8 days ago. Start using @ngaox/seo in your project by running `npm i @ngaox/seo`. There are no other projects in the npm registry using @ngaox/seo.
- 393Next-gen browser and mobile automation test framework for Node.js
https://github.com/webdriverio/webdriverio
Next-gen browser and mobile automation test framework for Node.js - webdriverio/webdriverio
- 394Cache for HTTP requests in Angular application.
https://github.com/nigrosimone/ng-http-caching
Cache for HTTP requests in Angular application. Contribute to nigrosimone/ng-http-caching development by creating an account on GitHub.
- 395A true material timepicker
https://github.com/tonysamperi/ngx-mat-timepicker
A true material timepicker. Contribute to tonysamperi/ngx-mat-timepicker development by creating an account on GitHub.
- 396WrapPixel's Elite Angular 17 Lite is a competent, powerful, and carefully handcrafted Angular Admin Template with a clean and minimalist design aesthetic.
https://github.com/wrappixel/elite-angular-lite
WrapPixel's Elite Angular 17 Lite is a competent, powerful, and carefully handcrafted Angular Admin Template with a clean and minimalist design aesthetic. - GitHub - wrappixel/elite-angular-li...
- 397🐿 A flexible and straightforward library that caches HTTP requests in Angular
https://github.com/ngneat/cashew
🐿 A flexible and straightforward library that caches HTTP requests in Angular - ngneat/cashew
- 398🦄 The Key to a Better Translation Experience
https://github.com/jsverse/transloco-keys-manager
🦄 The Key to a Better Translation Experience. Contribute to jsverse/transloco-keys-manager development by creating an account on GitHub.
- 399Jest configuration preset for Angular projects.
https://github.com/thymikee/jest-preset-angular
Jest configuration preset for Angular projects. Contribute to thymikee/jest-preset-angular development by creating an account on GitHub.
- 400starter kit with angular
https://github.com/starter-kits-usmb/front-angular
starter kit with angular . Contribute to starter-kits-usmb/front-angular development by creating an account on GitHub.
- 401Highcharts directive for Angular
https://github.com/cebor/angular-highcharts
Highcharts directive for Angular. Contribute to cebor/angular-highcharts development by creating an account on GitHub.
- 402Quickly create dynamic JavaScript charts with ZingChart & Angular.
https://github.com/zingchart/zingchart-angular
Quickly create dynamic JavaScript charts with ZingChart & Angular. - zingchart/zingchart-angular
- 403The internationalization (i18n) library for Angular
https://github.com/ngx-translate/core
The internationalization (i18n) library for Angular - ngx-translate/core
- 404Basic Angular & Lua - FiveM Boilerplate: A streamlined starter kit for web and in-game development with hot builds and utility scripts
https://github.com/FRACTAL-GAME-STUDIOS/fractal_boilerplate_lua_angular
Basic Angular & Lua - FiveM Boilerplate: A streamlined starter kit for web and in-game development with hot builds and utility scripts - FRACTAL-GAME-STUDIOS/fractal_boilerplate_lua_angular
- 405SAP BTP & Fiori App templates implemented with third-party frontend frameworks such as React, Vue, and Angular.
https://github.com/meta-d/sap-fiori-templates
SAP BTP & Fiori App templates implemented with third-party frontend frameworks such as React, Vue, and Angular. - meta-d/sap-fiori-templates
- 406Angular Template For Three.js
https://github.com/makimenko/angular-template-for-threejs
Angular Template For Three.js. Contribute to makimenko/angular-template-for-threejs development by creating an account on GitHub.
- 407Angular testing library for mocking components, directives, pipes, services and facilitating TestBed setup
https://github.com/help-me-mom/ng-mocks
Angular testing library for mocking components, directives, pipes, services and facilitating TestBed setup - help-me-mom/ng-mocks
- 408Angular Libraries Support lists community libs support for each Angular version
https://github.com/eneajaho/ngx-libs
Angular Libraries Support lists community libs support for each Angular version - eneajaho/ngx-libs
- 409Angular 18+ library for D3 based line, bar, donut and date/timeline charts with multiple entry points. A configurable service for token handling is provided.
https://github.com/Angular2Guy/ngx-simple-charts
Angular 18+ library for D3 based line, bar, donut and date/timeline charts with multiple entry points. A configurable service for token handling is provided. - Angular2Guy/ngx-simple-charts
- 410JavaScript API for Chrome and Firefox
https://github.com/puppeteer/puppeteer
JavaScript API for Chrome and Firefox. Contribute to puppeteer/puppeteer development by creating an account on GitHub.
- 411Multiple dates picker based on Angular Material.
https://github.com/lekhmanrus/ngx-multiple-dates
Multiple dates picker based on Angular Material. Contribute to lekhmanrus/ngx-multiple-dates development by creating an account on GitHub.
- 412A Jest transformer with source map support that lets you use Jest to test projects written in TypeScript.
https://github.com/kulshekhar/ts-jest
A Jest transformer with source map support that lets you use Jest to test projects written in TypeScript. - kulshekhar/ts-jest
- 413Simpler, cleaner Angular unit tests
https://github.com/Ninja-Squad/ngx-speculoos
Simpler, cleaner Angular unit tests . Contribute to Ninja-Squad/ngx-speculoos development by creating an account on GitHub.
- 414The FullStacksDev Angular and Firebase base template
https://github.com/FullStacksDev/angular-and-firebase-template
The FullStacksDev Angular and Firebase base template - FullStacksDev/angular-and-firebase-template
- 415Repository representing different testing use cases for Angular and Spring
https://github.com/rainerhahnekamp/how-do-i-test
Repository representing different testing use cases for Angular and Spring - rainerhahnekamp/how-do-i-test
- 416:sparkles: Easy, Reusable Animation Utility library for Angular
https://github.com/filipows/angular-animations
:sparkles: Easy, Reusable Animation Utility library for Angular - filipows/angular-animations
- 417Home
https://www.split.io
Split is a feature delivery platform that powers feature flag management, software experimentation, and continuous delivery.
- 418Free Angular admin template for Mantis design
https://github.com/codedthemes/mantis-free-angular-admin-template
Free Angular admin template for Mantis design. Contribute to codedthemes/mantis-free-angular-admin-template development by creating an account on GitHub.
- 419Darkbox Gallery is a highly configurable lightbox themed gallery library for Angular applications using the ivy engine (Angular 15+).
https://github.com/failed-successfully/ngx-darkbox-gallery-library
Darkbox Gallery is a highly configurable lightbox themed gallery library for Angular applications using the ivy engine (Angular 15+). - failed-successfully/ngx-darkbox-gallery-library
- 420ng-apexcharts is an implementation of apexcharts for angular. It comes with one simple component that enables you to use apexcharts in an angular project.
https://github.com/apexcharts/ng-apexcharts
ng-apexcharts is an implementation of apexcharts for angular. It comes with one simple component that enables you to use apexcharts in an angular project. - apexcharts/ng-apexcharts
- 421Translate routes using ngx-translate
https://github.com/gilsdav/ngx-translate-router
Translate routes using ngx-translate. Contribute to gilsdav/ngx-translate-router development by creating an account on GitHub.
- 422Powerful virtual data grid smartsheet with advanced customization. Best features from excel plus incredible performance 🔋
https://github.com/revolist/revogrid
Powerful virtual data grid smartsheet with advanced customization. Best features from excel plus incredible performance 🔋 - revolist/revogrid
- 423Component testing support for Angular
https://github.com/nightwatchjs/nightwatch-plugin-angular
Component testing support for Angular. Contribute to nightwatchjs/nightwatch-plugin-angular development by creating an account on GitHub.
- 424Beautify the Web with awesome layout animations
https://github.com/Char2sGu/layout-projection
Beautify the Web with awesome layout animations. Contribute to Char2sGu/layout-projection development by creating an account on GitHub.
- 425Datta able free Angular 18 admin template
https://github.com/codedthemes/datta-able-free-angular-admin-template
Datta able free Angular 18 admin template. Contribute to codedthemes/datta-able-free-angular-admin-template development by creating an account on GitHub.
- 426Angular 2 translation module i18n (internationalization) from JSON file with pluralization (Zero value state included)
https://github.com/doorgets/ng-translate
Angular 2 translation module i18n (internationalization) from JSON file with pluralization (Zero value state included) - doorgets/ng-translate
- 427Update SEO title and meta tags easily in Angular apps
https://github.com/avivharuzi/ngx-seo
Update SEO title and meta tags easily in Angular apps - avivharuzi/ngx-seo
- 428Post processor to remove SSR Code from bundles when served
https://github.com/xsip/ngx-ssr-code-remover
Post processor to remove SSR Code from bundles when served - xsip/ngx-ssr-code-remover
- 429Use three.js with your Angular project in a declarative way. ngx-three generates Angular components for many three.js classes
https://github.com/demike/ngx-three
Use three.js with your Angular project in a declarative way. ngx-three generates Angular components for many three.js classes - demike/ngx-three
- 430A wrapper for the Google Charts library written in Angular.
https://github.com/FERNman/angular-google-charts
A wrapper for the Google Charts library written in Angular. - FERNman/angular-google-charts
- 431Angular Image Slider with Lightbox.
https://github.com/sanjayV/ng-image-slider
Angular Image Slider with Lightbox. Contribute to sanjayV/ng-image-slider development by creating an account on GitHub.
- 432devkit/packages/swc-angular at main · jscutlery/devkit
https://github.com/jscutlery/devkit/tree/main/packages/swc-angular
Tools that make Angular developer's life easier. Contribute to jscutlery/devkit development by creating an account on GitHub.
- 433A project with components for create a slide with content in your application angular
https://github.com/criar-art/slidecontent-angular
A project with components for create a slide with content in your application angular - criar-art/slidecontent-angular
- 434Angular testing made easy with shallow rendering and easy mocking. https://getsaf.github.io/shallow-render
https://github.com/getsaf/shallow-render
Angular testing made easy with shallow rendering and easy mocking. https://getsaf.github.io/shallow-render - getsaf/shallow-render
- 435Angular-Slickgrid is a wrapper of the lightning fast & customizable SlickGrid datagrid, it also includes multiple Styling Themes
https://github.com/ghiscoding/Angular-Slickgrid
Angular-Slickgrid is a wrapper of the lightning fast & customizable SlickGrid datagrid, it also includes multiple Styling Themes - ghiscoding/Angular-Slickgrid
- 436Material datetimepicker for @angular/material
https://github.com/kuhnroyal/mat-datetimepicker
Material datetimepicker for @angular/material. Contribute to kuhnroyal/mat-datetimepicker development by creating an account on GitHub.
- 437A scheduler view component for
https://github.com/michelebombardi/angular-calendar-scheduler
A scheduler view component for. Contribute to michelebombardi/angular-calendar-scheduler development by creating an account on GitHub.
- 438Stripe Web Elements
https://stripe.com/docs/stripe-js
Create your own checkout flows with prebuilt UI components.
- 439Customizable admin dashboard template based on Angular 10+
https://github.com/akveo/ngx-admin
Customizable admin dashboard template based on Angular 10+ - akveo/ngx-admin
- 440🐠 An Angular service for managing directed acyclic graphs
https://github.com/ngneat/dag
🐠 An Angular service for managing directed acyclic graphs - ngneat/dag
- 441Beautiful charts for Angular based on Chart.js
https://github.com/valor-software/ng2-charts
Beautiful charts for Angular based on Chart.js. Contribute to valor-software/ng2-charts development by creating an account on GitHub.
- 442Helper functions to help write unit tests in Angular using mocks and spies
https://github.com/duluca/angular-unit-test-helper
Helper functions to help write unit tests in Angular using mocks and spies - duluca/angular-unit-test-helper
- 443Angular Directive (library) for FormKit's Auto Animate. Demo: https://ng-auto-animate.netlify.app
https://github.com/ajitzero/ng-auto-animate
Angular Directive (library) for FormKit's Auto Animate. Demo: https://ng-auto-animate.netlify.app - ajitzero/ng-auto-animate
- 444Highcharts official integration for Angular
https://github.com/highcharts/highcharts-angular
Highcharts official integration for Angular. Contribute to highcharts/highcharts-angular development by creating an account on GitHub.
- 445DataTables with Angular
https://github.com/l-lin/angular-datatables
DataTables with Angular. Contribute to l-lin/angular-datatables development by creating an account on GitHub.
- 446⛩ Nest + Prisma + Apollo + Angular 🏮 Full Stack GraphQL Starter Kit ⛩
https://github.com/ZenSoftware/zen
⛩ Nest + Prisma + Apollo + Angular 🏮 Full Stack GraphQL Starter Kit ⛩ - ZenSoftware/zen
- 447Angular Templates to build top quality web apps!
https://angular-templates.io/
Speed up your front-end web development with premium Angular templates. Angular Bootstrap and Material starters developed and designed by experts!
- 448⏳ date-fns pipes for Angular
https://github.com/joanllenas/ngx-date-fns
⏳ date-fns pipes for Angular. Contribute to joanllenas/ngx-date-fns development by creating an account on GitHub.
- 449A package that elegantly animates number changes, creating a visually engaging transition from one value to another, perfect for counting or displaying real-time data updates.
https://github.com/hm21/ngx-count-animation
A package that elegantly animates number changes, creating a visually engaging transition from one value to another, perfect for counting or displaying real-time data updates. - hm21/ngx-count-anim...
- 450100% unit testing coverage of the popular Angular demo app - Tour of Heroes.
https://github.com/kristiyan-velkov/angular-tour-of-heroes-jest-100-coverage
100% unit testing coverage of the popular Angular demo app - Tour of Heroes. - kristiyan-velkov/angular-tour-of-heroes-jest-100-coverage
- 451Native Angular date/time picker component styled by Twitter Bootstrap
https://github.com/dalelotts/angular-bootstrap-datetimepicker
Native Angular date/time picker component styled by Twitter Bootstrap - dalelotts/angular-bootstrap-datetimepicker
- 452Ignite UI for Angular is a complete library of Angular-native, Material-based Angular UI components with the fastest grids and charts, Pivot Grid, Dock Manager, Hierarchical Grid, and more.
https://github.com/IgniteUI/igniteui-angular
Ignite UI for Angular is a complete library of Angular-native, Material-based Angular UI components with the fastest grids and charts, Pivot Grid, Dock Manager, Hierarchical Grid, and more. - GitH...
- 453Angular Universal carousel
https://github.com/uiuniversal/ngu-carousel
Angular Universal carousel. Contribute to uiuniversal/ngu-carousel development by creating an account on GitHub.
- 454Angular library for easier use of the View Transitions API
https://github.com/DerStimmler/ngx-easy-view-transitions
Angular library for easier use of the View Transitions API - DerStimmler/ngx-easy-view-transitions
- 455Server-side rendering and Prerendering for Angular
https://github.com/angular/universal
Server-side rendering and Prerendering for Angular - angular/universal
- 456Angular Component for FusionCharts JavaScript Charting Library
https://github.com/fusioncharts/angular-fusioncharts
Angular Component for FusionCharts JavaScript Charting Library - fusioncharts/angular-fusioncharts
- 457Seamless REST/GraphQL API mocking library for browser and Node.js.
https://github.com/mswjs/msw
Seamless REST/GraphQL API mocking library for browser and Node.js. - mswjs/msw
- 458A lightweight Angular Library for building drag and drop flow charts. Chart behavior and steps are customizable. Data can be exported or uploaded in json format.
https://github.com/joel-wenzel/ng-flowchart
A lightweight Angular Library for building drag and drop flow charts. Chart behavior and steps are customizable. Data can be exported or uploaded in json format. - joel-wenzel/ng-flowchart
- 459Angular pipes for localizing numbers and dates using Globalize.
https://github.com/code-art-eg/angular-globalize
Angular pipes for localizing numbers and dates using Globalize. - code-art-eg/angular-globalize
- 460Angular material datetime range picker with daily, weekly, monthly, quarterly & yearly levels
https://github.com/BhavinPatel04/ngx-datetime-range-picker
Angular material datetime range picker with daily, weekly, monthly, quarterly & yearly levels - BhavinPatel04/ngx-datetime-range-picker
- 461Official CKEditor 5 Angular 5+ component.
https://github.com/ckeditor/ckeditor5-angular
Official CKEditor 5 Angular 5+ component. Contribute to ckeditor/ckeditor5-angular development by creating an account on GitHub.
- 462📃 The documentation engine for Angular projects
https://github.com/ng-doc/ng-doc
📃 The documentation engine for Angular projects. Contribute to ng-doc/ng-doc development by creating an account on GitHub.
- 463Angular library used to integrate with Descope (Deprecated)
https://github.com/descope/angular-sdk
Angular library used to integrate with Descope (Deprecated) - descope/angular-sdk
- 464🌍 All in one i18n extension for VS Code
https://github.com/lokalise/i18n-ally
🌍 All in one i18n extension for VS Code. Contribute to lokalise/i18n-ally development by creating an account on GitHub.
- 465Highly configurable date picker built for Angular applications
https://github.com/vlio20/angular-datepicker
Highly configurable date picker built for Angular applications - vlio20/angular-datepicker
- 466owl-carousel for Angular >=6
https://github.com/vitalii-andriiovskyi/ngx-owl-carousel-o
owl-carousel for Angular >=6. Contribute to vitalii-andriiovskyi/ngx-owl-carousel-o development by creating an account on GitHub.
- 467UI Grid: an Angular Data Grid
https://github.com/angular-ui/ui-grid
UI Grid: an Angular Data Grid. Contribute to angular-ui/ui-grid development by creating an account on GitHub.
- 468A fully type-safe and lightweight internationalization library for all your TypeScript and JavaScript projects.
https://github.com/ivanhofer/typesafe-i18n
A fully type-safe and lightweight internationalization library for all your TypeScript and JavaScript projects. - ivanhofer/typesafe-i18n
- 469Highly configurable and flexible translations loader for @ngx-translate/core
https://github.com/larscom/ngx-translate-module-loader
Highly configurable and flexible translations loader for @ngx-translate/core - larscom/ngx-translate-module-loader
- 470DevTools for Redux with hot reloading, action replay, and customizable UI
https://github.com/reduxjs/redux-devtools/
DevTools for Redux with hot reloading, action replay, and customizable UI - reduxjs/redux-devtools
- 471The official Angular component for FullCalendar
https://github.com/ng-fullcalendar/ng-fullcalendar
The official Angular component for FullCalendar. Contribute to fullcalendar/fullcalendar-angular development by creating an account on GitHub.
- 472Wrapper for Angular services
https://github.com/jm2097/ngx-generic-rest-service
Wrapper for Angular services. Contribute to ju4n97/ngx-generic-rest-service development by creating an account on GitHub.
- 473A flexible calendar component for angular 15.0+ that can display events on a month, week or day view.
https://github.com/mattlewis92/angular-calendar
A flexible calendar component for angular 15.0+ that can display events on a month, week or day view. - mattlewis92/angular-calendar
- 474An angular (ver >= 2.x) directive for ECharts (ver >= 3.x)
https://github.com/xieziyu/ngx-echarts
An angular (ver >= 2.x) directive for ECharts (ver >= 3.x) - xieziyu/ngx-echarts
- 475scuri
https://www.npmjs.com/package/scuri
A spec generator schematic - Spec Create Update Read (class - component, service, directive and dependencies) Incorporate (them in the result). Latest version: 1.4.0, last published: a year ago. Start using scuri in your project by running `npm i scuri`. There are no other projects in the npm registry using scuri.
- 476How do I test
https://www.youtube.com/playlist?list=PLu062eICIOdGAJ4AgTzHI6EokHnB90bRJ
Videos covering specific testing use cases for Angular and Spring
- 477ag-charts/packages/ag-charts-angular at latest · ag-grid/ag-charts
https://github.com/ag-grid/ag-charts/tree/latest/packages/ag-charts-angular
AG Charts is a fully-featured and highly customizable JavaScript charting library. The professional choice for developers building enterprise applications - ag-grid/ag-charts
- 478Angular Website Templates | ThemeForest
https://themeforest.net/search/angular
Get 683 angular website templates on ThemeForest such as MatDash Material Angular Admin Template, Fuse - Angular 18+ Admin Template, Silicon - Angular Business & Technology Template
- 479Build system optimized for JavaScript and TypeScript, written in Rust
https://github.com/vercel/turbo
Build system optimized for JavaScript and TypeScript, written in Rust - vercel/turborepo
- 480Socket - Secure your dependencies. Ship with confidence.
https://socket.dev/
Socket fights vulnerabilities and provides visibility, defense-in-depth, and proactive supply chain protection for JavaScript, Python, and Go dependencies.
- 481A set of convenient utilities to make Angular testing with Jasmine and Karma simpler.
https://github.com/babybeet/angular-testing-kit
A set of convenient utilities to make Angular testing with Jasmine and Karma simpler. - lazycuh/angular-testing-kit
- 482Testing an NgRx project
https://timdeschryver.dev/blog/testing-an-ngrx-project
How write maintainable NgRx tests within an Angular application
- 483Build software better, together
https://github.com/storybooks/storybook-
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 484JHipster - Full Stack Platform for the Modern Developer! | JHipster
https://www.jhipster.tech
JHipster is a development platform to quickly generate, develop, and deploy modern web applications + microservice architectures
- 485Build software better, together
https://github.com/mrdoob/three.js.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 486Build software better, together
https://github.com/jackocnr/intl-tel-input.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 487Build software better, together
https://github.com/shikijs/shiki.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 488Build software better, together
https://github.com/apache/incubator-echarts.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 489Descope | Authentication and user management platform
https://www.descope.com
Create and customize user journeys for any app – from authentication and authorization to MFA and federation. Get set up in minutes with our no-code CIAM platform.
- 490🦊 🚀 A Powerful Tool to Simplify Your Angular Tests
https://github.com/ngneat/spectator
🦊 🚀 A Powerful Tool to Simplify Your Angular Tests - ngneat/spectator
- 491A Comprehensive Introduction
https://www.youtube.com/watch?v=emnwsVy8wRs
Introduction | Angular Unit Testing Made Easy: A Comprehensive IntroductionDive deep into Angular Unit Testing with this comprehensive tutorial! Master the a...
- 492Testing Frameworks for Javascript | Write, Run, Debug | Cypress
https://www.cypress.io/
Simplify front-end testing with Cypress' open-source app. Explore our versatile testing frameworks for browser-based applications and components.
- 493A powerful, flexible, Markdown-based authoring framework
https://markdoc.dev
From personal blogs to massive documentation sites, Markdoc is a content authoring system that grows with you.
- 494Redux - A JS library for predictable and maintainable global state management | Redux
https://redux.js.org/.
A JS library for predictable and maintainable global state management
- 4955600+ free vector icons for web design
https://tabler.io/icons
Tabler Icons: 5628 free and open source SVG icons. Customizable size, color and stroke. Available for React, SolidJS, Vue, Figma and more for free!
- 496Angular Material
https://material.angular.io/
UI component infrastructure and Material Design components for Angular web applications.
- 497Angular Material
https://material.angular.io/cdk/drag-drop/overview
UI component infrastructure and Material Design components for Angular web applications.
- 498Taiga UI
https://taiga-ui.dev/
A powerful set of open source components for Angular
- 499Angular rich text editor component | CKEditor 5 documentation
https://ckeditor.com/docs/ckeditor5/latest/installation/getting-started/frameworks/angular.html
Learn how to install, integrate, configure, and develop CKEditor 5. Get to know the CKEditor 5 Framework. Browse through the API documentation and online samples.
- 500Angular | Uppy
https://uppy.io/docs/angular/
Angular components for Uppy UI plugins.
- 501NGXUI - UI Library for Angular
https://ngxui.com/docs
Explore NGXUI, a collection of beautiful Angular UI components designed to enhance your web applications looks.
- 502The Most Advanced WYSIWYG Editor | Trusted Rich Text Editor | TinyMCE
https://www.tiny.cloud/
TinyMCE is the most advanced WYSIWYG HTML editor designed to simplify website content creation. The rich text editing platform that helped launched Atlassian, Medium, Evernote and more.
- 503Tiptap - Dev Toolkit Editor Suite
https://tiptap.dev/.
Tiptap is a suite of content editing & real time collaboration tools. Build editor experiences like Notion in weeks, not years.
- 504An example application using Cesium with Angular.
https://github.com/Developer-Plexscape/cesium-angular-example
An example application using Cesium with Angular. Contribute to Developer-Plexscape/cesium-angular-example development by creating an account on GitHub.
- 505Omnedia
https://github.com/omnedia
Omnedia has 33 repositories available. Follow their code on GitHub.
- 506Angular Neomorphism UI kit
https://github.com/koskosergej/ngx-neumorphic
Angular Neomorphism UI kit. Contribute to koskosergej/ngx-neumorphic development by creating an account on GitHub.
- 507Building dynamic form in Angular with Fluent API and JSON
https://github.com/fluent-form/fluent-form
Building dynamic form in Angular with Fluent API and JSON - fluent-form/fluent-form
- 508@unpic/angular – Unpic
https://unpic.pics/img/angular/
High-performance, responsive Angular images
- 509Angular 16+ package for generating and using inline SVG icons in your project
https://github.com/jannicz/ng-svg-icon-sprite
Angular 16+ package for generating and using inline SVG icons in your project - jannicz/ng-svg-icon-sprite
- 510The Platform for 3D Geospatial
https://cesium.com
The Cesium platform provides the foundations any software application needs to utilize 3D geospatial data: visualization, data pipelines, curated data, and analytics. Based on open standards for data formats, open APIs for customization and integration, and built with an open source core, Cesium is open, interoperable, and incredibly precise.
- 511Angular & online library for Microsoft Fluent UI icons
https://github.com/bennymeg/ngx-fluent-ui
Angular & online library for Microsoft Fluent UI icons - bennymeg/ngx-fluent-ui
- 512Heroicons
https://heroicons.com
Beautiful hand-crafted SVG icons, by the makers of Tailwind CSS.
- 513Angular binding of mapbox-gl-js
https://github.com/Wykks/ngx-mapbox-gl
Angular binding of mapbox-gl-js. Contribute to Wykks/ngx-mapbox-gl development by creating an account on GitHub.
- 514Power the Possible with AI-powered Search - Sinequa
https://www.sinequa.com/
Fuel innovation and workplace efficiency the Leading AI-Powered Search Platform. Leading global organizations trust Sinequa.
- 515Angular component to display ellipsis and material tooltip dynamically
https://github.com/wandri/angular-ellipsis-tooltip
Angular component to display ellipsis and material tooltip dynamically - wandri/angular-ellipsis-tooltip
- 516Allows us to check if an element is within the browsers visual viewport
https://github.com/k3nsei/ng-in-viewport
Allows us to check if an element is within the browsers visual viewport - k3nsei/ng-in-viewport
- 517Tons of extensively featured packages for Angular, VUE and React Projects
https://github.com/rxweb/rxweb
Tons of extensively featured packages for Angular, VUE and React Projects - rxweb/rxweb
- 518A simple circle progress component created for Angular based on SVG Graphics.
https://github.com/bootsoon/ng-circle-progress
A simple circle progress component created for Angular based on SVG Graphics. - bootsoon/ng-circle-progress
- 519gridstack.js/angular at master · gridstack/gridstack.js
https://github.com/gridstack/gridstack.js/tree/master/angular/
Build interactive dashboards in minutes. Contribute to gridstack/gridstack.js development by creating an account on GitHub.
- 520Drag and Drop for Angular
https://github.com/ng-dnd/ng-dnd
Drag and Drop for Angular. Contribute to ng-dnd/ng-dnd development by creating an account on GitHub.
- 521imaskjs/packages/angular-imask at master · uNmAnNeR/imaskjs
https://github.com/uNmAnNeR/imaskjs/tree/master/packages/angular-imask
vanilla javascript input mask. Contribute to uNmAnNeR/imaskjs development by creating an account on GitHub.
- 522🤖 A declarative library for handling hotkeys in Angular applications
https://github.com/ngneat/hotkeys
🤖 A declarative library for handling hotkeys in Angular applications - ngneat/hotkeys
- 523dfts-common/libs/dfts-qrcode at main · Dafnik/dfts-common
https://github.com/Dafnik/dfts-common/tree/main/libs/dfts-qrcode
TypeScript and Angular libraries. Contribute to Dafnik/dfts-common development by creating an account on GitHub.
- 524timdeschryver/ng-signal-forms
https://github.com/timdeschryver/ng-signal-forms
Contribute to timdeschryver/ng-signal-forms development by creating an account on GitHub.
- 525Icon Library for Prime UI Libraries
https://github.com/primefaces/primeicons
Icon Library for Prime UI Libraries. Contribute to primefaces/primeicons development by creating an account on GitHub.
- 526Angular wrapper for Remixicon icon library
https://github.com/adisreyaj/angular-remix-icon
Angular wrapper for Remixicon icon library. Contribute to adisreyaj/angular-remix-icon development by creating an account on GitHub.
- 527File input management for Angular Material
https://github.com/daemons88/ngx-custom-material-file-input
File input management for Angular Material. Contribute to daemons88/ngx-custom-material-file-input development by creating an account on GitHub.
- 528Utilities for Angular
https://github.com/ngxtension/ngxtension-platform
Utilities for Angular. Contribute to ngxtension/ngxtension-platform development by creating an account on GitHub.
- 529Simpler, cleaner Angular validation error messages
https://github.com/Ninja-Squad/ngx-valdemort
Simpler, cleaner Angular validation error messages - Ninja-Squad/ngx-valdemort
- 530Color picker widget for the Angular (version 2 and newer)
https://github.com/zefoy/ngx-color-picker
Color picker widget for the Angular (version 2 and newer) - zefoy/ngx-color-picker
- 531📝 CodeMirror 6 wrapper for Angular
https://github.com/acrodata/code-editor
📝 CodeMirror 6 wrapper for Angular. Contribute to acrodata/code-editor development by creating an account on GitHub.
- 532Observable/Promise Cache Decorator
https://github.com/angelnikolov/ts-cacheable
Observable/Promise Cache Decorator. Contribute to angelnikolov/ts-cacheable development by creating an account on GitHub.
- 533Angular Drag Drop Layout is a lightweight, dependency-free Angular library for creating highly customizable, responsive grid layouts with drag-and-drop functionality. Built with Angular 18 and utilizing Angular Signals, this library provides a seamless and optimized experience for building dynamic and interactive layouts.
https://github.com/skutam/angular-drag-drop-layout
Angular Drag Drop Layout is a lightweight, dependency-free Angular library for creating highly customizable, responsive grid layouts with drag-and-drop functionality. Built with Angular 18 and util...
- 534Official TinyMCE Angular Component
https://github.com/tinymce/tinymce-angular
Official TinyMCE Angular Component. Contribute to tinymce/tinymce-angular development by creating an account on GitHub.
- 535Angular solution that allows to stick the elements such as header, menu, sidebar or any other block on the page.
https://github.com/matheo/ngx-sticky-kit
Angular solution that allows to stick the elements such as header, menu, sidebar or any other block on the page. - matheo/ngx-sticky-kit
- 536Angular 8+ module for detecting when elements are resized
https://github.com/fidian/ngx-resize-observer
Angular 8+ module for detecting when elements are resized - fidian/ngx-resize-observer
- 537IBAN directives and pipes for Angular
https://github.com/fundsaccess/angular-iban
IBAN directives and pipes for Angular. Contribute to fundsaccess/angular-iban development by creating an account on GitHub.
- 538An Angular library containing simple input components and a property editor component which automatically builds a form for editing all properties of any object.
https://github.com/heinerwalter/ngx-property-editor
An Angular library containing simple input components and a property editor component which automatically builds a form for editing all properties of any object. - heinerwalter/ngx-property-editor
- 539Angular module that detects when elements are visible. Uses IntersectionObserver.
https://github.com/fidian/ngx-visibility
Angular module that detects when elements are visible. Uses IntersectionObserver. - fidian/ngx-visibility
- 540Angular wrapper for jodit and jodit-pro WYSIWYG editor supporting Angular >=12 and ESM.
https://github.com/julianpoemp/ngx-jodit/
Angular wrapper for jodit and jodit-pro WYSIWYG editor supporting Angular >=12 and ESM. - julianpoemp/ngx-jodit
- 541An Angular component that can be used to create frosted glass effect of icons.
https://github.com/wadie/ngx-icon-blur
An Angular component that can be used to create frosted glass effect of icons. - wadie/ngx-icon-blur
- 542ng-as
https://www.npmjs.com/package/ng-as
Angular pipe and directive for type casting template variables.. Latest version: 18.0.1, last published: a month ago. Start using ng-as in your project by running `npm i ng-as`. There are no other projects in the npm registry using ng-as.
- 543Reactive Extensions for Angular.
https://github.com/rx-angular/rx-angular
Reactive Extensions for Angular. Contribute to rx-angular/rx-angular development by creating an account on GitHub.
- 544roosterjs library for angular!!
https://github.com/insurance-technologies/ng-rooster
roosterjs library for angular!! Contribute to insurance-technologies/ng-rooster development by creating an account on GitHub.
- 545A simple angular material file input component which lets the user drag and drop files, or select files with the native file picker.
https://github.com/telebroad/ngx-file-drag-drop
A simple angular material file input component which lets the user drag and drop files, or select files with the native file picker. - telebroad/ngx-file-drag-drop
- 546Tabler Icons components library for your Angular applications
https://github.com/pierreavn/angular-tabler-icons
Tabler Icons components library for your Angular applications - pierreavn/angular-tabler-icons
- 547Code editor component for Angular applications.
https://github.com/ngstack/code-editor
Code editor component for Angular applications. Contribute to ngstack/code-editor development by creating an account on GitHub.
- 548Keyboard shortcuts for Angular 2 apps
https://github.com/brtnshrdr/angular2-hotkeys
Keyboard shortcuts for Angular 2 apps. Contribute to brtnshrdr/angular2-hotkeys development by creating an account on GitHub.
- 549Simple yet elegant Material color picker for Angular
https://github.com/KroneCorylus/ngx-colors
Simple yet elegant Material color picker for Angular - KroneCorylus/ngx-colors
- 550Angular reactive breakpoint observer based on Signals.
https://github.com/tutkli/ngx-breakpoint-observer
Angular reactive breakpoint observer based on Signals. - tutkli/ngx-breakpoint-observer
- 551🖋️ Rich Text Editor for angular using ProseMirror
https://github.com/sibiraj-s/ngx-editor
🖋️ Rich Text Editor for angular using ProseMirror. Contribute to sibiraj-s/ngx-editor development by creating an account on GitHub.
- 552Angular(also Angular 17) WYSIWYG HTML Rich Text Editor (from ngWig - https://github.com/stevermeister/ngWig)
https://github.com/stevermeister/ngx-wig
Angular(also Angular 17) WYSIWYG HTML Rich Text Editor (from ngWig - https://github.com/stevermeister/ngWig) - stevermeister/ngx-wig
- 553An Angular front-end framework Tailored for your swiss branded business web application, Oblique provides a standardized corporate design look and feel as well as a collection of ready-to-use Angular components. Oblique, through its fully customizable master layout, takes care of the application's structure, letting you focus on the content.
https://github.com/oblique-bit/oblique
An Angular front-end framework Tailored for your swiss branded business web application, Oblique provides a standardized corporate design look and feel as well as a collection of ready-to-use Angul...
- 554Angular Material Library provide extra components for every project
https://github.com/h2qutc/angular-material-components
Angular Material Library provide extra components for every project - h2qutc/angular-material-components
- 555Angular ResizeObserver
https://github.com/ChristianKohler/ng-resize-observer
Angular ResizeObserver. Contribute to ChristianKohler/ng-resize-observer development by creating an account on GitHub.
- 556Use Heroicons (https://heroicons.com) in your Angular application
https://github.com/dimaslz/ng-heroicons
Use Heroicons (https://heroicons.com) in your Angular application - GitHub - dimaslz/ng-heroicons: Use Heroicons (https://heroicons.com) in your Angular application
- 557Multiline text with ellipsis for angular 9+
https://github.com/lentschi/ngx-ellipsis
Multiline text with ellipsis for angular 9+. Contribute to lentschi/ngx-ellipsis development by creating an account on GitHub.
- 558An Angular library based on floating ui with already ready-made components to use
https://github.com/al-march/ngx-popovers
An Angular library based on floating ui with already ready-made components to use - al-march/ngx-popovers
- 559:star: Native angular select component
https://github.com/ng-select/ng-select
:star: Native angular select component. Contribute to ng-select/ng-select development by creating an account on GitHub.
- 560Angular UI kit
https://github.com/skysoft-tech/sky-ui
Angular UI kit. Contribute to skysoft-tech/sky-ui development by creating an account on GitHub.
- 561Angular 11 file and folder drop library
https://github.com/georgipeltekov/ngx-file-drop
Angular 11 file and folder drop library. Contribute to georgipeltekov/ngx-file-drop development by creating an account on GitHub.
- 562Angular pipe for sanitizing your unsafe content
https://github.com/embarq/safe-pipe
Angular pipe for sanitizing your unsafe content. Contribute to embarq/safe-pipe development by creating an account on GitHub.
- 563Easy to use Angular components for files upload
https://github.com/valor-software/ng2-file-upload
Easy to use Angular components for files upload. Contribute to valor-software/ng2-file-upload development by creating an account on GitHub.
- 564is an Angular directive that automatically adjusts the width of an input element. Its is unique because it both shrinks and increases the width.
https://github.com/joshuawwright/ngx-autosize-input
is an Angular directive that automatically adjusts the width of an input element. Its is unique because it both shrinks and increases the width. - joshuawwright/ngx-autosize-input
- 565Angular Share Buttons ☂
https://github.com/MurhafSousli/ngx-sharebuttons
Angular Share Buttons ☂. Contribute to MurhafSousli/ngx-sharebuttons development by creating an account on GitHub.
- 566drag and drop file component
https://github.com/pIvan/file-upload
drag and drop file component. Contribute to pIvan/file-upload development by creating an account on GitHub.
- 567An alternative to `*ngIf; else` directive for simplify conditions into HTML template for Angular application.
https://github.com/nigrosimone/ng-condition
An alternative to `*ngIf; else` directive for simplify conditions into HTML template for Angular application. - nigrosimone/ng-condition
- 568CropperJS integration for Angular +6
https://github.com/matheusdavidson/angular-cropperjs
CropperJS integration for Angular +6. Contribute to matheusdavidson/angular-cropperjs development by creating an account on GitHub.
- 569Angular UI Component library for the Open Insurance Platform
https://github.com/allianz/ng-aquila
Angular UI Component library for the Open Insurance Platform - allianz/ng-aquila
- 570Angular wrapper around azure maps
https://github.com/arnaudleclerc/ng-azure-maps
Angular wrapper around azure maps. Contribute to arnaudleclerc/ng-azure-maps development by creating an account on GitHub.
- 571Star Rating Angular Component written in typescript, based on css only techniques.
https://github.com/BioPhoton/angular-star-rating
Star Rating Angular Component written in typescript, based on css only techniques. - BioPhoton/angular-star-rating
- 572Angular structural directive for sharing data as local variable into html component template.
https://github.com/nigrosimone/ng-let
Angular structural directive for sharing data as local variable into html component template. - nigrosimone/ng-let
- 573Code (number/chars/otp/password) input component for angular 7, 8, 9, 10, 11, 12+ projects including Ionic 4, 5 +
https://github.com/AlexMiniApps/angular-code-input
Code (number/chars/otp/password) input component for angular 7, 8, 9, 10, 11, 12+ projects including Ionic 4, 5 + - AlexMiniApps/angular-code-input
- 574📦 Just a Bootstrap Icons Picker for Angular
https://github.com/gdgvda/ngx-bootstrap-icons-picker
📦 Just a Bootstrap Icons Picker for Angular. Contribute to gdgvda/ngx-bootstrap-icons-picker development by creating an account on GitHub.
- 575Quicklink prefetching strategy for the Angular router
https://github.com/mgechev/ngx-quicklink
Quicklink prefetching strategy for the Angular router - mgechev/ngx-quicklink
- 576Angular Components Library | Responsive & Modern | Syncfusion
https://www.syncfusion.com/angular-components
The Syncfusion Angular components library offers more than 85 cross-browser, responsive, and lightweight components for building modern web applications.
- 577dyte-io/ui-kit
https://github.com/dyte-io/ui-kit/tree/staging/packages/angular-library
Dyte's UI library for creating meeting interfaces. - dyte-io/ui-kit
- 578Minimal Design, a set of components for Angular 16
https://github.com/A-l-y-l-e/Alyle-UI
Minimal Design, a set of components for Angular 16 - A-l-y-l-e/Alyle-UI
- 579Library to support Credit Card input masking and validation
https://github.com/timofei-iatsenko/angular-cc-library
Library to support Credit Card input masking and validation - timofei-iatsenko/angular-cc-library
- 580Utilities to assist in the use of reactive Angular forms
https://github.com/pjlamb12/ngx-reactive-forms-utils
Utilities to assist in the use of reactive Angular forms - pjlamb12/ngx-reactive-forms-utils
- 581Want types in your forms? Want to have nested forms? This is the place to be...
https://github.com/gparlakov/forms-typed
Want types in your forms? Want to have nested forms? This is the place to be... - gparlakov/forms-typed
- 582A tiny color picker component for modern Angular apps
https://github.com/ngx-eco/angular-colorful
A tiny color picker component for modern Angular apps - ngx-eco/angular-colorful
- 583Angular directive that provides file input functionality in Angular forms.
https://github.com/jwelker110/file-input-accessor
Angular directive that provides file input functionality in Angular forms. - jwelker110/file-input-accessor
- 584Cutting-edge tools powering Angular full-stack development.
https://github.com/goetzrobin/spartan
Cutting-edge tools powering Angular full-stack development. - goetzrobin/spartan
- 585The missing file input component for Angular Material.
https://github.com/hackingharold/ngx-dropzone
The missing file input component for Angular Material. - hackingharold/ngx-dropzone
- 586Teradata UI Platform built on Angular Material
https://github.com/Teradata/covalent/
Teradata UI Platform built on Angular Material. Contribute to Teradata/covalent development by creating an account on GitHub.
- 587Angular wrapper for floating-ui
https://github.com/tonysamperi/ngx-float-ui
Angular wrapper for floating-ui. Contribute to tonysamperi/ngx-float-ui development by creating an account on GitHub.
- 588ng-zen is a versatile Angular library and CLI tool offering UI-kit Angular schematics for streamlined integration into projects. The tool is currently in the Alpha phase. ⚠️ See more info here https://github.com/Kordrad/ng-zen/issues/62
https://github.com/Kordrad/ng-zen
ng-zen is a versatile Angular library and CLI tool offering UI-kit Angular schematics for streamlined integration into projects. The tool is currently in the Alpha phase. ⚠️ See more info here http...
- 589Angular Plugin to make masks on form fields and html elements.
https://github.com/JsDaddy/ngx-mask
Angular Plugin to make masks on form fields and html elements. - JsDaddy/ngx-mask
- 590Angular component and service for inlining SVGs allowing them to be easily styled with CSS.
https://github.com/czeckd/angular-svg-icon
Angular component and service for inlining SVGs allowing them to be easily styled with CSS. - czeckd/angular-svg-icon
- 591Angular5 Components and Directives for Fontawesome
https://github.com/travelist/angular2-fontawesome
Angular5 Components and Directives for Fontawesome - travelist/angular2-fontawesome
- 592🔌 A handy FilePond adapter component for Angular
https://github.com/pqina/ngx-filepond
🔌 A handy FilePond adapter component for Angular. Contribute to pqina/ngx-filepond development by creating an account on GitHub.
- 593Angular Material UI numeric range input form field. It is based on control value accessor.
https://github.com/dineeek/ngx-numeric-range-form-field
Angular Material UI numeric range input form field. It is based on control value accessor. - dineeek/ngx-numeric-range-form-field
- 594This Angular module allows you to use the Bootstrap Icons in your angular application without additional dependencies.
https://github.com/avmaisak/ngx-bootstrap-icons
This Angular module allows you to use the Bootstrap Icons in your angular application without additional dependencies. - avmaisak/ngx-bootstrap-icons
- 595Angular Bar Rating
https://github.com/MurhafSousli/ngx-bar-rating
Angular Bar Rating. Contribute to MurhafSousli/ngx-bar-rating development by creating an account on GitHub.
- 596Official Angular component for Font Awesome 5+
https://github.com/FortAwesome/angular-fontawesome
Official Angular component for Font Awesome 5+. Contribute to FortAwesome/angular-fontawesome development by creating an account on GitHub.
- 597Responsive grid with draggable and resizable items for Angular applications.
https://github.com/katoid/angular-grid-layout
Responsive grid with draggable and resizable items for Angular applications. - katoid/angular-grid-layout
- 598Generic pipe for Angular application for use a component method into component template
https://github.com/nigrosimone/ng-generic-pipe
Generic pipe for Angular application for use a component method into component template - nigrosimone/ng-generic-pipe
- 599⚪️ SVG component to create placeholder loading, like Facebook cards loading.
https://github.com/ngneat/content-loader
⚪️ SVG component to create placeholder loading, like Facebook cards loading. - GitHub - ngneat/content-loader: ⚪️ SVG component to create placeholder loading, like Facebook cards loading.
- 600A directive for showing errors in Angular form controls
https://github.com/dgonzalez870/ngx-formcontrol-errors
A directive for showing errors in Angular form controls - dgonzalez870/ngx-formcontrol-errors
- 601Angular bindings for tiptap v2
https://github.com/sibiraj-s/ngx-tiptap
Angular bindings for tiptap v2. Contribute to sibiraj-s/ngx-tiptap development by creating an account on GitHub.
- 602A new take on an Angular implementation for Semantic UI
https://github.com/ngx-semantic/ngx-semantic
A new take on an Angular implementation for Semantic UI - ngx-semantic/ngx-semantic
- 603Angular UI Component Library
https://github.com/winona-ui/winonang
Angular UI Component Library. Contribute to winona-ui/winonang development by creating an account on GitHub.
- 604flow.js file upload for angular
https://github.com/flowjs/ngx-flow
flow.js file upload for angular. Contribute to flowjs/ngx-flow development by creating an account on GitHub.
- 605Simple file save with FileSaver.js
https://github.com/cipchk/ngx-filesaver
Simple file save with FileSaver.js. Contribute to cipchk/ngx-filesaver development by creating an account on GitHub.
- 606A React Rich Text Editor that's block-based (Notion style) and extensible. Built on top of Prosemirror and Tiptap.
https://github.com/TypeCellOS/BlockNote
A React Rich Text Editor that's block-based (Notion style) and extensible. Built on top of Prosemirror and Tiptap. - TypeCellOS/BlockNote
- 607Angular global trackBy property directive with strict type checking.
https://github.com/nigrosimone/ng-for-track-by-property
Angular global trackBy property directive with strict type checking. - nigrosimone/ng-for-track-by-property
- 608Collection of libraries to create an input mask which ensures that user types value according to predefined format.
https://github.com/taiga-family/maskito
Collection of libraries to create an input mask which ensures that user types value according to predefined format. - taiga-family/maskito
- 609validointi/validointi
https://github.com/validointi/validointi
Contribute to validointi/validointi development by creating an account on GitHub.
- 610Angular logger
https://github.com/dbfannin/ngx-logger
Angular logger. Contribute to dbfannin/ngx-logger development by creating an account on GitHub.
- 611Little library to use css variables with @angular/material
https://github.com/johannesjo/angular-material-css-vars
Little library to use css variables with @angular/material - johannesjo/angular-material-css-vars
- 612Ngx Simple Text editor or ST editor is a simple native text editor component for Angular 9+.
https://github.com/Raiper34/ngx-simple-text-editor
Ngx Simple Text editor or ST editor is a simple native text editor component for Angular 9+. - Raiper34/ngx-simple-text-editor
- 613Angular Library for SBB
https://github.com/sbb-design-systems/sbb-angular
Angular Library for SBB. Contribute to sbb-design-systems/sbb-angular development by creating an account on GitHub.
- 614Style agnostic, accessible primitives for Angular.
https://github.com/ng-primitives/ng-primitives
Style agnostic, accessible primitives for Angular. - ng-primitives/ng-primitives
- 615Angular UI Component Library based on DevUI Design
https://github.com/DevCloudFE/ng-devui
Angular UI Component Library based on DevUI Design - DevCloudFE/ng-devui
- 616The tooltip is a pop-up tip that appears when you hover over an item or click on it.
https://github.com/cloudfactorydk/ng2-tooltip-directive
The tooltip is a pop-up tip that appears when you hover over an item or click on it. - cloudfactorydk/ng2-tooltip-directive
- 617Angular wrapper for Tippy.js
https://github.com/farengeyt451/ngx-tippy-wrapper
Angular wrapper for Tippy.js. Contribute to farengeyt451/ngx-tippy-wrapper development by creating an account on GitHub.
- 618🚀 Style and Component Library for Angular
https://github.com/swimlane/ngx-ui
🚀 Style and Component Library for Angular. Contribute to swimlane/ngx-ui development by creating an account on GitHub.
- 619MiniRx - The reactive state management platform
https://github.com/spierala/mini-rx-store
MiniRx - The reactive state management platform. Contribute to spierala/mini-rx-store development by creating an account on GitHub.
- 620Fast and reliable Tethys Design components for Angular(快速可靠的 Tethys Angular 组件库)
https://github.com/atinc/ngx-tethys
Fast and reliable Tethys Design components for Angular(快速可靠的 Tethys Angular 组件库) - atinc/ngx-tethys
- 621Extensible orchestrator for UI and forms for Angular
https://github.com/orchestratora/orchestrator
Extensible orchestrator for UI and forms for Angular - orchestratora/orchestrator
- 622Angular syntax highlighting module
https://github.com/MurhafSousli/ngx-highlightjs
Angular syntax highlighting module. Contribute to MurhafSousli/ngx-highlightjs development by creating an account on GitHub.
- 623🌊 A flexible and fun JavaScript file upload library
https://github.com/pqina/filepond
🌊 A flexible and fun JavaScript file upload library - pqina/filepond
- 624Angular UI Switch (Forked)
https://github.com/OuterlimitsTech/olt-ngx-ui-switch
Angular UI Switch (Forked). Contribute to OuterlimitsTech/olt-ngx-ui-switch development by creating an account on GitHub.
- 625NGWR – Angular UI components library
https://github.com/thekhegay/ngwr
NGWR – Angular UI components library. Contribute to thekhegay/ngwr development by creating an account on GitHub.
- 626Angular view layer for Slate
https://github.com/worktile/slate-angular
Angular view layer for Slate. Contribute to worktile/slate-angular development by creating an account on GitHub.
- 627SignalDB is a local JavaScript database with a MongoDB-like interface and TypeScript support, enabling optimistic UI with signal-based reactivity across multiple frameworks. It integrates easily with libraries like Angular, Solid.js, Preact, and Vue, simplifying data management with schema-less design, in-memory storage, and fast queries.
https://github.com/maxnowack/signaldb
SignalDB is a local JavaScript database with a MongoDB-like interface and TypeScript support, enabling optimistic UI with signal-based reactivity across multiple frameworks. It integrates easily wi...
- 628A JavaScript library providing multiple simultaneous, stable, fault-tolerant and resumable/restartable file uploads via the HTML5 File API.
https://github.com/flowjs/flow.js
A JavaScript library providing multiple simultaneous, stable, fault-tolerant and resumable/restartable file uploads via the HTML5 File API. - flowjs/flow.js
- 629JSON powered forms for Angular
https://github.com/formio/angular
JSON powered forms for Angular. Contribute to formio/angular development by creating an account on GitHub.
- 630Angular QR code, Barcode, DataMatrix, scanner component using ZXing.
https://github.com/zxing-js/ngx-scanner
Angular QR code, Barcode, DataMatrix, scanner component using ZXing. - zxing-js/ngx-scanner
- 631Customizable Slack-like emoji picker for Angular
https://github.com/scttcper/ngx-emoji-mart
Customizable Slack-like emoji picker for Angular. Contribute to scttcper/ngx-emoji-mart development by creating an account on GitHub.
- 632Angular wrappers for IDS Enterprise components
https://github.com/infor-design/enterprise-ng
Angular wrappers for IDS Enterprise components. Contribute to infor-design/enterprise-ng development by creating an account on GitHub.
- 633The Most Complete Angular UI Component Library
https://github.com/primefaces/primeng
The Most Complete Angular UI Component Library. Contribute to primefaces/primeng development by creating an account on GitHub.
- 634🅰️ ngular-RU Software Development Kit
https://github.com/Angular-RU/angular-ru-sdk
🅰️ ngular-RU Software Development Kit. Contribute to Angular-RU/angular-ru-sdk development by creating an account on GitHub.
- 635Angular directives using the native HTML Drag And Drop API
https://github.com/reppners/ngx-drag-drop
Angular directives using the native HTML Drag And Drop API - reppners/ngx-drag-drop
- 636🚀 A mini, yet powerful state management library for Angular.
https://github.com/worktile/store
🚀 A mini, yet powerful state management library for Angular. - worktile/store
- 637Collection of awesome Angular libraries
https://github.com/DmitryEfimenko/ngspot
Collection of awesome Angular libraries. Contribute to DmitryEfimenko/ngspot development by creating an account on GitHub.
- 638A set of common utils for consuming Web APIs with Angular
https://github.com/taiga-family/ng-web-apis
A set of common utils for consuming Web APIs with Angular - taiga-family/ng-web-apis
- 639Angular Resumable Upload Module
https://github.com/kukhariev/ngx-uploadx
Angular Resumable Upload Module. Contribute to kukhariev/ngx-uploadx development by creating an account on GitHub.
- 640Multiple Select Dropdown Component
https://github.com/NileshPatel17/ng-multiselect-dropdown
Multiple Select Dropdown Component. Contribute to NileshPatel17/ng-multiselect-dropdown development by creating an account on GitHub.
- 641Angular loader components
https://github.com/pjlamb12/angular-loaders
Angular loader components. Contribute to pjlamb12/angular-loaders development by creating an account on GitHub.
- 642Simple drag and drop with dragula
https://github.com/valor-software/ng2-dragula
Simple drag and drop with dragula. Contribute to valor-software/ng2-dragula development by creating an account on GitHub.
- 643Bimeister PupaKit ⚠️ API is now stabilizing — Do not use in production! Angular UI Kit based on an atomic approach to building interfaces
https://github.com/bimeister/pupakit
Bimeister PupaKit ⚠️ API is now stabilizing — Do not use in production! Angular UI Kit based on an atomic approach to building interfaces - bimeister/pupakit
- 644A library for loading spinner for Angular 4 - 17.
https://github.com/napster2210/ngx-spinner
A library for loading spinner for Angular 4 - 17. Contribute to Napster2210/ngx-spinner development by creating an account on GitHub.
- 645Angular 2 Dropdown Multiselect
https://github.com/CuppaLabs/angular2-multiselect-dropdown
Angular 2 Dropdown Multiselect. Contribute to CuppaLabs/angular2-multiselect-dropdown development by creating an account on GitHub.
- 646Efficient client-side storage for Angular: simple API + performance + Observables + validation
https://github.com/cyrilletuzi/angular-async-local-storage
Efficient client-side storage for Angular: simple API + performance + Observables + validation - cyrilletuzi/angular-async-local-storage
- 647A configurable Mobile UI components based on Ant Design Mobile and Angular. 🐜
https://github.com/NG-ZORRO/ng-zorro-antd-mobile
A configurable Mobile UI components based on Ant Design Mobile and Angular. 🐜 - NG-ZORRO/ng-zorro-antd-mobile
- 648Angular UI Kit
https://github.com/osspkg/onega-ui
Angular UI Kit. Contribute to osspkg/onega-ui development by creating an account on GitHub.
- 649Declarative, incremental state management library
https://github.com/state-adapt/state-adapt
Declarative, incremental state management library. Contribute to state-adapt/state-adapt development by creating an account on GitHub.
- 650Enterprise level Angular UI framework from Alauda Frontend Team.
https://github.com/alauda/ui
Enterprise level Angular UI framework from Alauda Frontend Team. - alauda/ui
- 651Virtual Keyboard for Javascript, React, Angular, Vue - simple-keyboard
https://github.com/virtual-keyboard-javascript/virtual-keyboard-javascript.github.io
Virtual Keyboard for Javascript, React, Angular, Vue - simple-keyboard - virtual-keyboard-javascript/virtual-keyboard-javascript.github.io
- 652🚀 NGXS - State Management for Angular
https://github.com/ngxs/store
🚀 NGXS - State Management for Angular. Contribute to ngxs/store development by creating an account on GitHub.
- 653Antwerp UI is a component interface library for building user interfaces and responsive web apps.
https://github.com/digipolisantwerp/antwerp-ui_angular
Antwerp UI is a component interface library for building user interfaces and responsive web apps. - digipolisantwerp/antwerp-ui_angular
- 654An Angular implementation of the Carbon Design System for IBM.
https://github.com/carbon-design-system/carbon-components-angular
An Angular implementation of the Carbon Design System for IBM. - carbon-design-system/carbon-components-angular
- 655Repository with logos of languages and tech or company
https://github.com/criar-art/angular-techs-logos
Repository with logos of languages and tech or company - criar-art/angular-techs-logos
- 656ng-web-apis/libs/storage/README.md at main · taiga-family/ng-web-apis
https://github.com/taiga-family/ng-web-apis/blob/main/libs/storage/README.md
A set of common utils for consuming Web APIs with Angular - taiga-family/ng-web-apis
- 657:boom: Customizable Angular UI Library based on Eva Design System :new_moon_with_face::sparkles:Dark Mode
https://github.com/akveo/nebular
:boom: Customizable Angular UI Library based on Eva Design System :new_moon_with_face::sparkles:Dark Mode - akveo/nebular
- 658Popover component for Angular
https://github.com/ncstate-sat/popover
Popover component for Angular. Contribute to ncstate-sat/popover development by creating an account on GitHub.
- 659🚁 A Powerful Tooltip and Popover for Angular Applications
https://github.com/ngneat/helipopper
🚁 A Powerful Tooltip and Popover for Angular Applications - ngneat/helipopper
- 660ngx-sherlock is an Angular tooling library to be used with the @politie/sherlock distributed reactive state management library.
https://github.com/politie/ngx-sherlock
ngx-sherlock is an Angular tooling library to be used with the @politie/sherlock distributed reactive state management library. - politie/ngx-sherlock
- 661🤖 A collection of tools to make your Angular views more modular, scalable, and maintainable
https://github.com/ngneat/overview
🤖 A collection of tools to make your Angular views more modular, scalable, and maintainable - ngneat/overview
- 662An angular 15.0+ bootstrap confirmation popover
https://github.com/mattlewis92/angular-confirmation-popover
An angular 15.0+ bootstrap confirmation popover. Contribute to mattlewis92/angular-confirmation-popover development by creating an account on GitHub.
- 663Simple form development for complex and scalable solutions
https://github.com/simplifiedcourses/ngx-vest-forms
Simple form development for complex and scalable solutions - simplifiedcourses/ngx-vest-forms
- 664Moveable! Draggable! Resizable! Scalable! Rotatable! Warpable! Pinchable! Groupable! Snappable!
https://github.com/daybrush/moveable
Moveable! Draggable! Resizable! Scalable! Rotatable! Warpable! Pinchable! Groupable! Snappable! - daybrush/moveable
- 665A flexible and unopinionated edit in place library for Angular applications
https://github.com/ngneat/edit-in-place
A flexible and unopinionated edit in place library for Angular applications - ngneat/edit-in-place
- 666Angular gridster 2
https://github.com/tiberiuzuld/angular-gridster2
Angular gridster 2. Contribute to tiberiuzuld/angular-gridster2 development by creating an account on GitHub.
- 667ngx-logger is a powerful Angular library designed to streamline the implementation of custom logging functionalities within Angular applications. This library empowers developers to seamlessly integrate custom logging solutions tailored to their specific requirements, enabling efficient debugging, monitoring, and analytics
https://github.com/Xilerth/ngx-logger
ngx-logger is a powerful Angular library designed to streamline the implementation of custom logging functionalities within Angular applications. This library empowers developers to seamlessly inte...
- 668Sinequa's Angular-based Search Based Application (SBA) Framework
https://github.com/sinequa/sba-angular
Sinequa's Angular-based Search Based Application (SBA) Framework - sinequa/sba-angular
- 669A library that provides useful tools for Angular apps development.
https://github.com/pechemann/angular-toolbox
A library that provides useful tools for Angular apps development. - pechemann/angular-toolbox
- 670Clarity Angular is a scalable, accessible, customizable, open-source design system built for Angular.
https://github.com/vmware-clarity/ng-clarity
Clarity Angular is a scalable, accessible, customizable, open-source design system built for Angular. - vmware-clarity/ng-clarity
- 671Kirby Design System
https://github.com/kirbydesign/designsystem
Kirby Design System. Contribute to kirbydesign/designsystem development by creating an account on GitHub.
- 672Angular gRPC framework
https://github.com/smnbbrv/ngx-grpc
Angular gRPC framework. Contribute to smnbbrv/ngx-grpc development by creating an account on GitHub.
- 673ngx-awesome-uploader
https://www.npmjs.com/package/ngx-awesome-uploader
Angular Library for uploading files with Real-Time Progress bar, File Preview, Drag && Drop and Custom Template with Multi Language support. Latest version: 17.1.0, last published: 7 months ago. Start using ngx-awesome-uploader in your project by running `npm i ngx-awesome-uploader`. There are 2 other projects in the npm registry using ngx-awesome-uploader.
- 674Localstorage and sessionstorage manager - Angular service
https://github.com/PillowPillow/ng2-webstorage
Localstorage and sessionstorage manager - Angular service - PillowPillow/ng2-webstorage
- 675Tooltip for Angular, forked from cm-angular-tooltip
https://github.com/chandumaram/tooltip-testing
Tooltip for Angular, forked from cm-angular-tooltip - chandumaram/tooltip-testing
- 676Virtual/infinite scroll for Angular
https://github.com/dhilt/ngx-ui-scroll
Virtual/infinite scroll for Angular. Contribute to dhilt/ngx-ui-scroll development by creating an account on GitHub.
- 677🦄 Making sure your tailor-made error solution is seamless!
https://github.com/ngneat/error-tailor
🦄 Making sure your tailor-made error solution is seamless! - ngneat/error-tailor
- 678Angular UI Switch component
https://github.com/webcat12345/ngx-ui-switch
Angular UI Switch component. Contribute to webcat12345/ngx-ui-switch development by creating an account on GitHub.
- 679A context menu component for Angular
https://github.com/PerfectMemory/ngx-contextmenu
A context menu component for Angular. Contribute to PerfectMemory/ngx-contextmenu development by creating an account on GitHub.
- 680Angular 4, 5, 6, 7, 8 and 9 plugin for Froala WYSIWYG HTML Rich Text Editor.
https://github.com/froala/angular-froala-wysiwyg
Angular 4, 5, 6, 7, 8 and 9 plugin for Froala WYSIWYG HTML Rich Text Editor. - froala/angular-froala-wysiwyg
- 681A library of useful pipes for Angular apps
https://github.com/nglrx/pipes
A library of useful pipes for Angular apps. Contribute to nglrx/pipes development by creating an account on GitHub.
- 682Tools that make Angular developer's life easier.
https://github.com/jscutlery/devkit
Tools that make Angular developer's life easier. Contribute to jscutlery/devkit development by creating an account on GitHub.
- 683Modular map components for angular
https://github.com/ng-maps/ng-maps
Modular map components for angular. Contribute to ng-maps/ng-maps development by creating an account on GitHub.
- 684Official Angular bindings for Redux
https://github.com/reduxjs/angular-redux
Official Angular bindings for Redux. Contribute to reduxjs/angular-redux development by creating an account on GitHub.
- 685Actor-based state management & orchestration for complex app logic.
https://github.com/statelyai/xstate
Actor-based state management & orchestration for complex app logic. - statelyai/xstate
- 686e-oz/ngx-reactive-storage
https://github.com/e-oz/ngx-reactive-storage
Contribute to e-oz/ngx-reactive-storage development by creating an account on GitHub.
- 687Angular VCL ‒ an Angular component library based on the VCL CSS eco system
https://github.com/vcl/ng-vcl
Angular VCL ‒ an Angular component library based on the VCL CSS eco system - vcl/ng-vcl
- 688Open Source Angular Libraries contributed by @myndpm
https://github.com/myndpm/open-source
Open Source Angular Libraries contributed by @myndpm - myndpm/open-source
- 689📝 JSON powered / Dynamic forms for Angular
https://github.com/ngx-formly/ngx-formly
📝 JSON powered / Dynamic forms for Angular. Contribute to ngx-formly/ngx-formly development by creating an account on GitHub.
- 690Angular Markdown Editor. All-in-one Markdown Editor and Preview
https://github.com/ghiscoding/angular-markdown-editor
Angular Markdown Editor. All-in-one Markdown Editor and Preview - ghiscoding/angular-markdown-editor
- 691TypeScript and Angular libraries
https://github.com/Dafnik/dfts-common
TypeScript and Angular libraries. Contribute to Dafnik/dfts-common development by creating an account on GitHub.
- 692Clone of the Angular FlexLayout
https://github.com/ngbracket/ngx-layout
Clone of the Angular FlexLayout . Contribute to ngbracket/ngx-layout development by creating an account on GitHub.
- 693The ultimate icon library for Angular
https://github.com/ng-icons/ng-icons
The ultimate icon library for Angular. Contribute to ng-icons/ng-icons development by creating an account on GitHub.
- 694Angular (>=2) components for the Quill Rich Text Editor
https://github.com/KillerCodeMonkey/ngx-quill
Angular (>=2) components for the Quill Rich Text Editor - KillerCodeMonkey/ngx-quill
- 695🔔 A Simple Notification Service for Angular applications
https://github.com/sibiraj-s/ngx-notifier
🔔 A Simple Notification Service for Angular applications - sibiraj-s/ngx-notifier
- 696Reactive components made easy! Lithium provides utilities that enable seamless reactive state and event interactions for Angular components.
https://github.com/lVlyke/lithium-angular
Reactive components made easy! Lithium provides utilities that enable seamless reactive state and event interactions for Angular components. - lVlyke/lithium-angular
- 697A logger built with angular in mind
https://github.com/avernixtechnologies/angular-logger
A logger built with angular in mind. Contribute to avernixtechnologies/angular-logger development by creating an account on GitHub.
- 698Angular 14+ wrapper for RxDB
https://github.com/voznik/ngx-odm
Angular 14+ wrapper for RxDB. Contribute to voznik/ngx-odm development by creating an account on GitHub.
- 699Collection State Management Service for Angular
https://github.com/e-oz/ngx-collection
Collection State Management Service for Angular. Contribute to e-oz/ngx-collection development by creating an account on GitHub.
- 700Show busy/loading indicators on any element during a promise/Observable.
https://github.com/tiberiuzuld/angular-busy
Show busy/loading indicators on any element during a promise/Observable. - tiberiuzuld/angular-busy
- 701trellisorg/platform
https://github.com/trellisorg/platform
Contribute to trellisorg/platform development by creating an account on GitHub.
- 702Pretend to be using Angular
https://github.com/ngify/ngify
Pretend to be using Angular. Contribute to ngify/ngify development by creating an account on GitHub.
- 703Lazy load Angular component into HTML template without routing.
https://github.com/nigrosimone/ng-lazy-load-component
Lazy load Angular component into HTML template without routing. - nigrosimone/ng-lazy-load-component
- 704QR Code implementation based on Angular
https://github.com/OriginRing/qrcode.angular
QR Code implementation based on Angular. Contribute to OriginRing/qrcode.angular development by creating an account on GitHub.
- 705A service that wraps IndexedDB database in an Angular service. It exposes very simple observables API to enable the usage of IndexedDB without most of it plumbing.
https://github.com/assuncaocharles/ngx-indexed-db
A service that wraps IndexedDB database in an Angular service. It exposes very simple observables API to enable the usage of IndexedDB without most of it plumbing. - assuncaocharles/ngx-indexed-db
- 706A package to implement hero animations, allowing users to click on images and smoothly zoom them into a larger, immersive view, enhancing the user experience and interaction with images.
https://github.com/hm21/ngx-image-hero
A package to implement hero animations, allowing users to click on images and smoothly zoom them into a larger, immersive view, enhancing the user experience and interaction with images. - hm21/ngx...
- 707A light and easy to use notifications library for Angular.
https://github.com/flauc/angular2-notifications
A light and easy to use notifications library for Angular. - flauc/angular2-notifications
- 708A suite of dynamic and efficient tools designed for Angular 17+, enhancing application interactivity and aesthetics through innovative open-source libraries. 🚀
https://github.com/boris-jenicek/ng-vibe
A suite of dynamic and efficient tools designed for Angular 17+, enhancing application interactivity and aesthetics through innovative open-source libraries. 🚀 - boris-jenicek/ng-vibe
- 709🧙♀️ A Reactive Store with Magical Powers
https://github.com/ngneat/elf
🧙♀️ A Reactive Store with Magical Powers. Contribute to ngneat/elf development by creating an account on GitHub.
- 710(Angular Reactive) Forms with Benefits 😉
https://github.com/ngneat/reactive-forms
(Angular Reactive) Forms with Benefits 😉. Contribute to ngneat/reactive-forms development by creating an account on GitHub.
- 711An Angular component for displaying SVG icons.
https://github.com/anedomansky/ngx-icon
An Angular component for displaying SVG icons. Contribute to anedomansky/ngx-icon development by creating an account on GitHub.
- 712📜 Angular directive for infinite scrolling.
https://github.com/robingenz/ngx-infinite-scroll
📜 Angular directive for infinite scrolling. . Contribute to robingenz/ngx-infinite-scroll development by creating an account on GitHub.
- 713Simple QR code generator (SVG only) for Angular
https://github.com/larscom/ng-qrcode-svg
Simple QR code generator (SVG only) for Angular. Contribute to larscom/ng-qrcode-svg development by creating an account on GitHub.
- 714Render markdown with custom Angular templates
https://github.com/ericleib/ngx-remark
Render markdown with custom Angular templates. Contribute to ericleib/ngx-remark development by creating an account on GitHub.
- 715🍞 Angular project for sending Bootstrap based toast notifications including Vercel deployment
https://github.com/svierk/angular-bootstrap-toast-service
🍞 Angular project for sending Bootstrap based toast notifications including Vercel deployment - svierk/angular-bootstrap-toast-service
- 716🚀 Open-source - The world's easiest, most powerful Angular dialog modal framework. Confirmation box, Alert box, Toast notification, Cookie banner, Any dynamic dialog content.
https://github.com/boris-jenicek/ngx-awesome-popup
🚀 Open-source - The world's easiest, most powerful Angular dialog modal framework. Confirmation box, Alert box, Toast notification, Cookie banner, Any dynamic dialog content. - boris-jenicek/ng...
- 717Angular builder to build svg-icons using svg-to-ts
https://github.com/angular-extensions/svg-icons-builder
Angular builder to build svg-icons using svg-to-ts - angular-extensions/svg-icons-builder
- 718A loading spinner for Angular applications.
https://github.com/zak-c/ngx-loading
A loading spinner for Angular applications. Contribute to Zak-C/ngx-loading development by creating an account on GitHub.
- 719Markdoc for Angular
https://github.com/notiz-dev/ngx-markdoc
Markdoc for Angular. Contribute to notiz-dev/ngx-markdoc development by creating an account on GitHub.
- 720Easy to use AOT compatible QR code generator for Angular projects.
https://github.com/mnahkies/ng-qrcode
Easy to use AOT compatible QR code generator for Angular projects. - mnahkies/ng-qrcode
- 721Small tool to generate a Framework component (React, Preact, Angular, Svelte or Vue) from a SVG icons. Copy and paste the SVG icon content to the tool and you will have a basic framework template to use the SVG icon in your project.
https://github.com/dimaslz/icon-lib-builder
Small tool to generate a Framework component (React, Preact, Angular, Svelte or Vue) from a SVG icons. Copy and paste the SVG icon content to the tool and you will have a basic framework template t...
- 722Declarative, reactive, and template-driven SweetAlert2 integration for Angular
https://github.com/sweetalert2/ngx-sweetalert2
Declarative, reactive, and template-driven SweetAlert2 integration for Angular - sweetalert2/ngx-sweetalert2
- 723Opinionated simple state management for Angular
https://github.com/simplifiedcourses/ngx-signal-state
Opinionated simple state management for Angular. Contribute to simplifiedcourses/ngx-signal-state development by creating an account on GitHub.
- 724An Angular Tooltip Library
https://github.com/mkeller1992/ngx-tooltip-directives
An Angular Tooltip Library. Contribute to mkeller1992/ngx-tooltip-directives development by creating an account on GitHub.
- 725Angular 7+ Validator, a library handle validation messages easy and automatic
https://github.com/why520crazy/ngx-validator
Angular 7+ Validator, a library handle validation messages easy and automatic - why520crazy/ngx-validator
- 726📦 SVGs, fast and developer friendly in Angular
https://github.com/push-based/ngx-fast-svg
📦 SVGs, fast and developer friendly in Angular. Contribute to push-based/ngx-fast-svg development by creating an account on GitHub.
- 727Get Angular 8+ events fired when an element is mutated in the DOM.
https://github.com/fidian/ngx-mutation-observer
Get Angular 8+ events fired when an element is mutated in the DOM. - fidian/ngx-mutation-observer
- 728This minimalistic Angular directive, free from external dependencies, empowers you to effortlessly implement CSS animations on elements. These animations trigger when an element comes into view through scrolling on the page. It seamlessly integrates with your choice of CSS animations.
https://github.com/hm21/ngx-scroll-animations
This minimalistic Angular directive, free from external dependencies, empowers you to effortlessly implement CSS animations on elements. These animations trigger when an element comes into view thr...
- 729Monorepo for the @ngworker NPM organization. Packages for Angular applications and testing.
https://github.com/ngworker/ngworker
Monorepo for the @ngworker NPM organization. Packages for Angular applications and testing. - ngworker/ngworker
- 730🧐 A grid that allows you to sort all items via drag & drop. Items can be sorted in all directions (↕️ and ↔️). You can also select and sort multiple items at the same time by pressing ctrl and click on the item.
https://github.com/kreuzerk/ng-sortgrid
🧐 A grid that allows you to sort all items via drag & drop. Items can be sorted in all directions (↕️ and ↔️). You can also select and sort multiple items at the same time by pressing ctrl and ...
- 731JavaScript UI Components | Powerful UI Controls for Web Applications | Wijmo
http://wijmo.com/products/wijmo-5/
Use our JavaScript UI components to build advanced HTML5/JavaScript apps fast with zero dependencies. Learn more about Wijmo today.
- 732A comprehensive Angular Component Development Kit (CDK) offering a wide range of reusable UI components, built for minimal configuration and React-like ease of use. Fully customizable, themable, and continuously updated for seamless integration into your Angular projects.
https://github.com/WindMillCode/Windmillcode-Angular-CDK
A comprehensive Angular Component Development Kit (CDK) offering a wide range of reusable UI components, built for minimal configuration and React-like ease of use. Fully customizable, themable, an...
- 733Autocomplete input component and directive for google-maps built with angular and material design |
https://github.com/angular-material-extensions/google-maps-autocomplete
Autocomplete input component and directive for google-maps built with angular and material design | - angular-material-extensions/google-maps-autocomplete
- 734Fundamental Library for Angular is SAP Design System Angular component library
https://github.com/SAP/fundamental-ngx
Fundamental Library for Angular is SAP Design System Angular component library - SAP/fundamental-ngx
- 735Angular Line Awesome is an Angular component to manage Line Awesome icons.
https://github.com/marco-martins/angular-line-awesome
Angular Line Awesome is an Angular component to manage Line Awesome icons. - marco-martins/angular-line-awesome
- 736Awesome loader for angular applications. No wrappers only you elements
https://github.com/jsdaddy/ngx-loader-indicator
Awesome loader for angular applications. No wrappers only you elements - JsDaddy/ngx-loader-indicator
- 737🎨 Simplify SVG icon usage in your Angular project! Enhance the flexibility and manageability of your Angular project by using SVG sprites.
https://github.com/bodnya29179/Angular-Svg-Sprite
🎨 Simplify SVG icon usage in your Angular project! Enhance the flexibility and manageability of your Angular project by using SVG sprites. - bodnya29179/Angular-Svg-Sprite
- 738File uploader for Angular 18+
https://github.com/ZouYouShun/ngxf-uploader
File uploader for Angular 18+. Contribute to ZouYouShun/ngxf-uploader development by creating an account on GitHub.
- 739Angular progress bar ☄
https://github.com/MurhafSousli/ngx-progressbar
Angular progress bar ☄. Contribute to MurhafSousli/ngx-progressbar development by creating an account on GitHub.
- 740Build software better, together
https://github.com/eligrey/FileSaver.js.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 741Signal-based state management for Angular applications
https://github.com/zuriscript/signalstory
Signal-based state management for Angular applications - GitHub - zuriscript/signalstory: Signal-based state management for Angular applications
- 742✨🦊 NgComponentOutlet + Data-Binding + Full Lifecycle = NgxComponentOutlet for Angular 7, 8, 9, 10, 11, 12, 13, 14, 15, 16+
https://github.com/Indigosoft/ngxd
✨🦊 NgComponentOutlet + Data-Binding + Full Lifecycle = NgxComponentOutlet for Angular 7, 8, 9, 10, 11, 12, 13, 14, 15, 16+ - Indigosoft/ngxd
- 743Custom overlay-scrollbars with native scrolling mechanism
https://github.com/MurhafSousli/ngx-scrollbar
Custom overlay-scrollbars with native scrolling mechanism - MurhafSousli/ngx-scrollbar
- 744A fast and lightweight virtual scrolling solution for Angular that supports single column lists, grid lists and view caching.
https://github.com/lVlyke/lithium-ngx-virtual-scroll
A fast and lightweight virtual scrolling solution for Angular that supports single column lists, grid lists and view caching. - lVlyke/lithium-ngx-virtual-scroll
- 745Angular binding of maplibre-gl
https://github.com/maplibre/ngx-maplibre-gl
Angular binding of maplibre-gl. Contribute to maplibre/ngx-maplibre-gl development by creating an account on GitHub.
- 746Angular library for uploading and compressing images
https://github.com/dfa1234/ngx-image-compress
Angular library for uploading and compressing images - dfa1234/ngx-image-compress
- 747Build software better, together
https://github.com/ianstormtaylor/slate.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 748Angular UI Component Library based on Ant Design
https://github.com/NG-ZORRO/ng-zorro-antd
Angular UI Component Library based on Ant Design. Contribute to NG-ZORRO/ng-zorro-antd development by creating an account on GitHub.
- 749tansu is a lightweight, push-based state management library. It borrows the ideas and APIs originally designed and implemented by Svelte stores.
https://github.com/AmadeusITGroup/tansu
tansu is a lightweight, push-based state management library. It borrows the ideas and APIs originally designed and implemented by Svelte stores. - AmadeusITGroup/tansu
- 750Monaco Code Editor for Angular
https://github.com/jean-merelis/ngx-monaco-editor
Monaco Code Editor for Angular. Contribute to jean-merelis/ngx-monaco-editor development by creating an account on GitHub.
- 751Angular Ecommerce PWA Framework
https://github.com/graycoreio/daffodil
Angular Ecommerce PWA Framework. Contribute to graycoreio/daffodil development by creating an account on GitHub.
- 752Simply beautiful open-source icons
https://github.com/feathericons/feather
Simply beautiful open-source icons. Contribute to feathericons/feather development by creating an account on GitHub.
- 753Chop and cut Angular logs like a professional lumberjack.
https://github.com/ngworker/lumberjack
Chop and cut Angular logs like a professional lumberjack. - ngworker/lumberjack
- 754Action Logger
https://github.com/TALRACE/ngx-action-logger
Action Logger. Contribute to TALRACE/ngx-action-logger development by creating an account on GitHub.
- 755Library to provide tools to work with focus and focusable elements to improve user interfaces and accessibility.
https://github.com/Raiper34/ngx-focus-control
Library to provide tools to work with focus and focusable elements to improve user interfaces and accessibility. - Raiper34/ngx-focus-control
- 756A lightweight Angular library to add a loading spinner to your Angular Material buttons. 🙊
https://github.com/dkreider/ngx-loading-buttons
A lightweight Angular library to add a loading spinner to your Angular Material buttons. 🙊 - dkreider/ngx-loading-buttons
- 757A set of powerful angular tools 🔥
https://github.com/mikelgo/angular-kit
A set of powerful angular tools 🔥. Contribute to mikelgo/angular-kit development by creating an account on GitHub.
- 758Animated scrolling functionality for angular written in pure typescript
https://github.com/Nolanus/ngx-page-scroll
Animated scrolling functionality for angular written in pure typescript - Nolanus/ngx-page-scroll
- 759Infinite Scroll Directive for Angular
https://github.com/orizens/ngx-infinite-scroll
Infinite Scroll Directive for Angular. Contribute to orizens/ngx-infinite-scroll development by creating an account on GitHub.
- 760Reactive Forms validation shouldn't require the developer to write lots of HTML to show validation messages. This library makes it easy.
https://github.com/davidwalschots/angular-reactive-validation
Reactive Forms validation shouldn't require the developer to write lots of HTML to show validation messages. This library makes it easy. - davidwalschots/angular-reactive-validation
- 761Automatic page loading / progress bar for Angular
https://github.com/aitboudad/ngx-loading-bar
Automatic page loading / progress bar for Angular. Contribute to aitboudad/ngx-loading-bar development by creating an account on GitHub.
- 762Lightweight, Material Design inspired "go to top button". No dependencies. Pure Angular!
https://github.com/bartholomej/ngx-scrolltop
Lightweight, Material Design inspired "go to top button". No dependencies. Pure Angular! - bartholomej/ngx-scrolltop
- 763Angular module that uses SVG to create a circular progressbar
https://github.com/crisbeto/angular-svg-round-progressbar
Angular module that uses SVG to create a circular progressbar - crisbeto/angular-svg-round-progressbar
- 764Virtual Keyboard for Angular applications.
https://github.com/mohsen77sk/angular-touch-keyboard
Virtual Keyboard for Angular applications. Contribute to mohsen77sk/angular-touch-keyboard development by creating an account on GitHub.
- 765🖼 Load placeholder image on image error, Angular 5+
https://github.com/VadimDez/ngx-img-fallback
🖼 Load placeholder image on image error, Angular 5+ - VadimDez/ngx-img-fallback
- 766A reactive programming library for JavaScript applications, built with TypeScript.
https://github.com/politie/sherlock
A reactive programming library for JavaScript applications, built with TypeScript. - politie/sherlock
- 767Biblioteca de componentes Angular.
https://github.com/po-ui/po-angular
Biblioteca de componentes Angular. Contribute to po-ui/po-angular development by creating an account on GitHub.
- 768A fast and easy-to-use Angular QR Code Generator library with Ivy support
https://github.com/cordobo/angularx-qrcode
A fast and easy-to-use Angular QR Code Generator library with Ivy support - Cordobo/angularx-qrcode
- 769egjs-infinitegrid/packages/ngx-infinitegrid at master · naver/egjs-infinitegrid
https://github.com/naver/egjs-infinitegrid/tree/master/packages/ngx-infinitegrid
A module used to arrange card elements including content infinitely on a grid layout. - naver/egjs-infinitegrid
- 770Chilled loading buttons for angular2
https://github.com/johannesjo/angular2-promise-buttons
Chilled loading buttons for angular2. Contribute to johannesjo/angular2-promise-buttons development by creating an account on GitHub.
- 771Block UI Loader/Spinner for Angular
https://github.com/kuuurt13/ng-block-ui
Block UI Loader/Spinner for Angular. Contribute to kuuurt13/ng-block-ui development by creating an account on GitHub.
- 772The next open source file uploader for web browsers
https://github.com/transloadit/uppy
The next open source file uploader for web browsers :dog: - GitHub - transloadit/uppy: The next open source file uploader for web browsers
- 773Angular Material Extensions Library.
https://github.com/ng-matero/extensions
Angular Material Extensions Library. Contribute to ng-matero/extensions development by creating an account on GitHub.
- 774Jodit - Best WYSIWYG Editor for You
https://github.com/xdan/jodit
Jodit - Best WYSIWYG Editor for You. Contribute to xdan/jodit development by creating an account on GitHub.
- 775🎨 Color Pickers from Sketch, Photoshop, Chrome, Github, Twitter & more
https://github.com/scttcper/ngx-color
🎨 Color Pickers from Sketch, Photoshop, Chrome, Github, Twitter & more - scttcper/ngx-color
- 776Official Angular wrapper for fullPage.js https://alvarotrigo.com/angular-fullpage/
https://github.com/alvarotrigo/angular-fullpage
Official Angular wrapper for fullPage.js https://alvarotrigo.com/angular-fullpage/ - alvarotrigo/angular-fullpage
- 777Angular markdown editor based on ace editor
https://github.com/instance-oom/ngx-markdown-editor
Angular markdown editor based on ace editor . Contribute to instance-oom/ngx-markdown-editor development by creating an account on GitHub.
- 778An image cropper for Angular
https://github.com/Mawi137/ngx-image-cropper
An image cropper for Angular. Contribute to Mawi137/ngx-image-cropper development by creating an account on GitHub.
- 779ngx-modal-ease is a versatile Angular library providing a lightweight, simple, and performant modal.
https://github.com/GreenFlag31/modal-library
ngx-modal-ease is a versatile Angular library providing a lightweight, simple, and performant modal. - GreenFlag31/modal-library
- 780👻 A simple to use, highly customizable, and powerful modal for Angular Applications
https://github.com/ngneat/dialog
👻 A simple to use, highly customizable, and powerful modal for Angular Applications - ngneat/dialog
- 781Core Leaflet package for Angular.io
https://github.com/bluehalo/ngx-leaflet
Core Leaflet package for Angular.io. Contribute to bluehalo/ngx-leaflet development by creating an account on GitHub.
- 782Utility library for breaking down an Angular form into multiple components
https://github.com/cloudnc/ngx-sub-form
Utility library for breaking down an Angular form into multiple components - cloudnc/ngx-sub-form
- 783components/src/google-maps/README.md at main · angular/components
https://github.com/angular/components/blob/main/src/google-maps/README.md
Component infrastructure and Material Design components for Angular - angular/components
- 784OneSignal Angular
https://github.com/OneSignal/onesignal-ngx
OneSignal Angular. Contribute to OneSignal/onesignal-ngx development by creating an account on GitHub.
- 785Syncfusion Angular UI components library offer more than 50+ cross-browser, responsive, and lightweight angular UI controls for building modern web applications.
https://github.com/syncfusion/ej2-angular-ui-components
Syncfusion Angular UI components library offer more than 50+ cross-browser, responsive, and lightweight angular UI controls for building modern web applications. - GitHub - syncfusion/ej2-angular-...
- 786🔅 State manager for deeply nested states
https://github.com/Marcisbee/exome
🔅 State manager for deeply nested states. Contribute to Marcisbee/exome development by creating an account on GitHub.
- 787dotglitch-ngx/packages/common/src/components/lazy-loader at main · knackstedt/dotglitch-ngx
https://github.com/knackstedt/dotglitch-ngx/tree/main/packages/common/src/components/lazy-loader
A monorepo consisting of all the utilities for @dotglitch productions - knackstedt/dotglitch-ngx
- 788🍞 Smoking hot toast notifications for Angular.
https://github.com/ngxpert/hot-toast
🍞 Smoking hot toast notifications for Angular. Contribute to ngxpert/hot-toast development by creating an account on GitHub.
- 789Build software better, together
https://github.com/seiyria/ng2-fontawesome-
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 790⚡️ Useful pipes for Angular with no external dependencies!
https://github.com/danrevah/ngx-pipes
⚡️ Useful pipes for Angular with no external dependencies! - GitHub - danrevah/ngx-pipes: ⚡️ Useful pipes for Angular with no external dependencies!
- 791Component infrastructure and Material Design components for Angular
https://github.com/angular/components
Component infrastructure and Material Design components for Angular - angular/components
- 792👻 A lightweight library that makes it easier to use SVG icons in your Angular Application
https://github.com/ngneat/svg-icon
👻 A lightweight library that makes it easier to use SVG icons in your Angular Application - ngneat/svg-icon
- 793Angular implementation of BlockNote
https://github.com/dytab/BlockNoteAngular
Angular implementation of BlockNote. Contribute to dytab/ngx-blocknote development by creating an account on GitHub.
- 794Cloudimage responsive plugin will make your website load the exact image size you need depending on your user's screen size. Multiple pixel ratios are supported. Any questions or issues, please report to https://github.com/scaleflex/ng-cloudimage-responsive/issues
https://github.com/scaleflex/ng-cloudimage-responsive
Cloudimage responsive plugin will make your website load the exact image size you need depending on your user's screen size. Multiple pixel ratios are supported. Any questions or issues, please...
- 795Ignite UI for Angular is a complete library of Angular-native, Material-based Angular UI components with the fastest grids and charts, Pivot Grid, Dock Manager, Hierarchical Grid, and more.
https://github.com/IgniteUI/igniteui-angular
Ignite UI for Angular is a complete library of Angular-native, Material-based Angular UI components with the fastest grids and charts, Pivot Grid, Dock Manager, Hierarchical Grid, and more. - GitH...
- 796rdlabo-team/ionic-angular-collect-icons
https://github.com/rdlabo-team/ionic-angular-collect-icons
Contribute to rdlabo-team/ionic-angular-collect-icons development by creating an account on GitHub.
- 797Angular component for zoomable images
https://github.com/wittlock/ngx-image-zoom
Angular component for zoomable images. Contribute to wittlock/ngx-image-zoom development by creating an account on GitHub.
- 798Make beautiful, animated loading skeletons that automatically adapt to your Angular apps
https://github.com/willmendesneto/ngx-skeleton-loader
Make beautiful, animated loading skeletons that automatically adapt to your Angular apps - willmendesneto/ngx-skeleton-loader
- 799🍞 Angular Toastr
https://github.com/scttcper/ngx-toastr
🍞 Angular Toastr. Contribute to scttcper/ngx-toastr development by creating an account on GitHub.
- 800Modal/Dialog component crafted for Angular (Ivy-compatible)
https://github.com/maximelafarie/ngx-smart-modal
Modal/Dialog component crafted for Angular (Ivy-compatible) - maximelafarie/ngx-smart-modal
- 801Customizable JSON Schema-based forms with React, Angular and Vue support out of the box.
https://github.com/eclipsesource/jsonforms
Customizable JSON Schema-based forms with React, Angular and Vue support out of the box. - eclipsesource/jsonforms
- 802An opinionated toast component for Angular. A port of @emilkowalski's sonner.
https://github.com/tutkli/ngx-sonner
An opinionated toast component for Angular. A port of @emilkowalski's sonner. - tutkli/ngx-sonner
- 803Angular tree component
https://github.com/valor-software/ng2-tree
Angular tree component. Contribute to valor-software/ng2-tree development by creating an account on GitHub.
- 804A module that allows you to pretty print the inner HTML of ComponentFixtures, DebugElements, NativeElements or even plain HTML strings to the console. This is very useful for debugging Angular component tests in Jest
https://github.com/angular-extensions/pretty-html-log
A module that allows you to pretty print the inner HTML of ComponentFixtures, DebugElements, NativeElements or even plain HTML strings to the console. This is very useful for debugging Angular comp...
- 805Codemirror Wrapper for Angular
https://github.com/TypeCtrl/ngx-codemirror
Codemirror Wrapper for Angular. Contribute to scttcper/ngx-codemirror development by creating an account on GitHub.
- 806Automatically insert live Angular components into dynamic strings (based on their selector or any pattern of your choice) and render the result in the DOM.
https://github.com/MTobisch/ngx-dynamic-hooks
Automatically insert live Angular components into dynamic strings (based on their selector or any pattern of your choice) and render the result in the DOM. - Angular-Dynamic-Hooks/ngx-dynamic-hooks
- 807Angular 16 & Bootstrap 5 & Material Design 2.0 UI KIT
https://github.com/mdbootstrap/mdb-angular-ui-kit
Angular 16 & Bootstrap 5 & Material Design 2.0 UI KIT - mdbootstrap/mdb-angular-ui-kit
- 808Virtual Scroll for Angular Material Table
https://github.com/diprokon/ng-table-virtual-scroll
Virtual Scroll for Angular Material Table. Contribute to diprokon/ng-table-virtual-scroll development by creating an account on GitHub.
- 809Angular markdown component/directive/pipe/service to parse static, dynamic or remote content to HTML with syntax highlight and more...
https://github.com/jfcere/ngx-markdown
Angular markdown component/directive/pipe/service to parse static, dynamic or remote content to HTML with syntax highlight and more... - jfcere/ngx-markdown
- 810Image comparison slider. Compare images before and after. Supports React, Vue, Angular.
https://github.com/sneas/img-comparison-slider
Image comparison slider. Compare images before and after. Supports React, Vue, Angular. - sneas/img-comparison-slider
- 811🍌 Angular UI library to split views and allow dragging to resize areas using CSS flexbox layout.
https://github.com/bertrandg/angular-split
🍌 Angular UI library to split views and allow dragging to resize areas using CSS flexbox layout. - angular-split/angular-split
- 812🤖 Powerful asynchronous state management, server-state utilities and data fetching for the web. TS/JS, React Query, Solid Query, Svelte Query and Vue Query.
https://github.com/TanStack/query
🤖 Powerful asynchronous state management, server-state utilities and data fetching for the web. TS/JS, React Query, Solid Query, Svelte Query and Vue Query. - TanStack/query
- 813Simple state management in Angular with only Services and RxJS or Signal.
https://github.com/nigrosimone/ng-simple-state
Simple state management in Angular with only Services and RxJS or Signal. - nigrosimone/ng-simple-state
- 814Universal Model for Angular
https://github.com/universal-model/universal-model-angular
Universal Model for Angular. Contribute to universal-model/universal-model-angular development by creating an account on GitHub.
- 815A collection of useful components, directives and pipes for Angular.
https://github.com/Jaspero/ng-helpers
A collection of useful components, directives and pipes for Angular. - Jaspero/ng-helpers
- 816JavaScript image cropper.
https://github.com/fengyuanchen/cropperjs
JavaScript image cropper. Contribute to fengyuanchen/cropperjs development by creating an account on GitHub.
- 817Build software better, together
https://github.com/drozhzhin-n-e/ng2-tooltip-directive.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 818Reactive State for Angular
https://github.com/ngrx/platform
Reactive State for Angular. Contribute to ngrx/platform development by creating an account on GitHub.
- 819Easily show tooltips programmatically and/or declaratively in Angular
https://github.com/babybeet/angular-tooltip
Easily show tooltips programmatically and/or declaratively in Angular - lazycuh/angular-tooltip
- 820Rapid form development library for Angular
https://github.com/udos86/ng-dynamic-forms
Rapid form development library for Angular. Contribute to udos86/ng-dynamic-forms development by creating an account on GitHub.
- 821JavaScript library for creating map based web apps using Cesium and Angular
https://github.com/TGFTech/angular-cesium
JavaScript library for creating map based web apps using Cesium and Angular - articodeltd/angular-cesium
- 822Angular UI library to illustrate and validate a password's strength with material design
https://github.com/angular-material-extensions/password-strength
Angular UI library to illustrate and validate a password's strength with material design - GitHub - angular-material-extensions/password-strength: Angular UI library to illustrate and validate...
- 823Angular Material component that allow users to select a country or nationality with an autocomplete feature
https://github.com/angular-material-extensions/select-country
Angular Material component that allow users to select a country or nationality with an autocomplete feature - angular-material-extensions/select-country
- 824A javascript scrollbar plugin that hides the native scrollbars, provides custom styleable overlay scrollbars, and preserves the native functionality and feel.
https://github.com/KingSora/OverlayScrollbars
A javascript scrollbar plugin that hides the native scrollbars, provides custom styleable overlay scrollbars, and preserves the native functionality and feel. - KingSora/OverlayScrollbars
- 825Angular Setup
https://documentation.onesignal.com/docs/angular-setup
The Angular OneSignal Plugin is a JavaScript module that can be used to easily include OneSignal code in a website that uses Angular for its front-end codebase. Requirements OneSignal Account Your OneSignal App Id, available in Settings > Keys & IDs . If you have not done so already, you may ...
- 826ITER IDEA
https://www.iter-idea.com/
We build affordable yet powerful cloud services which innovate businesses, regardless of their size, nature or location. We are your bright IDEA!
- 827Tailwind CSS Angular - Flowbite
https://flowbite.com/docs/getting-started/angular/
Read this guide to learn how to install Tailwind CSS with Angular and set up the UI components from Flowbite to build enterprise-level web applications
- 828Build next level Ionic apps
https://eliteionic.com/
Elite Ionic provides the education and resources necessary to empower Ionic developers to create remarkable apps
- 829devkit/packages/microwave at main · jscutlery/devkit
https://github.com/jscutlery/devkit/tree/main/packages/microwave
Tools that make Angular developer's life easier. Contribute to jscutlery/devkit development by creating an account on GitHub.
- 830Bootstrap 5 & Angular - Free Material Design UI KIT
https://mdbootstrap.com/docs/angular/
700+ components, stunning templates, 1-min installation, extensive tutorials & huge community. MIT license - free for personal & commercial use.
- 831Learn to build professional-grade Ionic and Angular Applications
https://ionicstart.com/
Learn to build professional-grade Ionic and Angular Applications
- 832Build software better, together
https://github.com/shepherd-pro/shepherd.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 833Tailwind CSS Components, Templates and Tools - Tailkit
https://tailkit.com/
Carefully crafted UI Components, Templates and Tools for your next Tailwind CSS based project.
- 834Learn Ionic Framework with free step by step Ionic tutorials
https://ionicthemes.com/tutorials
All you need to know about Ionic Framework, the best tips and free code examples so you can get the most out of Ionic Framework.
- 835Ionic Framework - The Cross-Platform App Development Leader
https://ionicframework.com
Ionic empowers web developers to build leading cross-platform mobile apps and Progressive Web Apps (PWAs)
- 836A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
https://github.com/ionic-team/ionic-framework
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript. - ionic-team/ionic-framework
- 837The companion repo for the upcoming Total TypeScript book
https://github.com/total-typescript/total-typescript-book
The companion repo for the upcoming Total TypeScript book - total-typescript/total-typescript-book
- 838Bind your model types to angular FormGroup type
https://github.com/iamguid/ngx-mf
Bind your model types to angular FormGroup type. Contribute to iamguid/ngx-mf development by creating an account on GitHub.
- 839✈️ UI tour for Angular apps
https://github.com/hakimio/ngx-ui-tour
✈️ UI tour for Angular apps. Contribute to hakimio/ngx-ui-tour development by creating an account on GitHub.
- 840Real world application built with Angular 18, NgRx 18, nrwl/nx 18
https://github.com/stefanoslig/angular-ngrx-nx-realworld-example-app
Real world application built with Angular 18, NgRx 18, nrwl/nx 18 - stefanoslig/angular-ngrx-nx-realworld-example-app
- 841document-viewer/packages/ngx-doc-viewer at main · Marcelh1983/document-viewer
https://github.com/Marcelh1983/document-viewer/tree/main/packages/ngx-doc-viewer
Contribute to Marcelh1983/document-viewer development by creating an account on GitHub.
- 842JSON formatter and viewer in HTML for Angular
https://github.com/hivivo/ngx-json-viewer
JSON formatter and viewer in HTML for Angular. Contribute to hivivo/ngx-json-viewer development by creating an account on GitHub.
- 843devkit/packages/operators at main · jscutlery/devkit
https://github.com/jscutlery/devkit/tree/main/packages/operators
Tools that make Angular developer's life easier. Contribute to jscutlery/devkit development by creating an account on GitHub.
- 844Cookie Consent module for Angular.
https://github.com/tinesoft/ngx-cookieconsent
Cookie :cookie: Consent module for Angular. Contribute to tinesoft/ngx-cookieconsent development by creating an account on GitHub.
- 845UI-Router for Angular: State-based routing for Angular (v2+)
https://github.com/ui-router/angular
UI-Router for Angular: State-based routing for Angular (v2+) - ui-router/angular
- 846A set of little RxJS puzzles to practice your Observable skills
https://github.com/AngularWave/rxjs-challenge
A set of little RxJS puzzles to practice your Observable skills - AngularWave/rxjs-challenge
- 847angular-architects/module-federation-plugin
https://github.com/angular-architects/module-federation-plugin
Contribute to angular-architects/module-federation-plugin development by creating an account on GitHub.
- 848Angular Public Twitter Timeline Widget
https://github.com/mustafaer/angular-twitter-timeline
Angular Public Twitter Timeline Widget. Contribute to mustafaer/angular-twitter-timeline development by creating an account on GitHub.
- 849Angular utility for ensuring exhaustive checks on TypeScript discriminated unions, enhancing type safety and reliability.
https://github.com/soc221b/ngx-exhaustive-check
Angular utility for ensuring exhaustive checks on TypeScript discriminated unions, enhancing type safety and reliability. - soc221b/ngx-exhaustive-check
- 850Connect, collaborate, and grow with a community of TypeScript developers
https://github.com/typehero/typehero
Connect, collaborate, and grow with a community of TypeScript developers - typehero/typehero
- 851An Angular wrapper for the Web Audio API's AudioContext.
https://github.com/chrisguttandin/angular-audio-context
An Angular wrapper for the Web Audio API's AudioContext. - chrisguttandin/angular-audio-context
- 852⏲ SVG gauge component for Angular
https://github.com/hawkgs/ng2-gauge
⏲ SVG gauge component for Angular. Contribute to hawkgs/ng2-gauge development by creating an account on GitHub.
- 853🚩 Effortless feature flag management in Angular
https://github.com/pBouillon/ngx-flagr
🚩 Effortless feature flag management in Angular. Contribute to pBouillon/ngx-flagr development by creating an account on GitHub.
- 854A collection of @ngrx addons, including state persistence.
https://github.com/Michsior14/ngrx-addons
A collection of @ngrx addons, including state persistence. - GitHub - Michsior14/ngrx-addons: A collection of @ngrx addons, including state persistence.
- 855The fastest way to learn Ionic
https://ionicacademy.com/
Whether it’s for work or launching your own app - learn Ionic Angular quickly with step-by-step video courses and join our private community today.
- 856An open source library to build node-based UI with Angular 16+
https://github.com/artem-mangilev/ngx-vflow
An open source library to build node-based UI with Angular 16+ - artem-mangilev/ngx-vflow
- 857An angular component for PDFJS and ViewerJS (Supports all versions of angular)
https://github.com/intbot/ng2-pdfjs-viewer
An angular component for PDFJS and ViewerJS (Supports all versions of angular) - intbot/ng2-pdfjs-viewer
- 858NGRX Traits is a library to help you compose and reuse state logic in your angular app. There is two versions, @ngrx-traits/signals supports ngrx-signals, and @ngrx-traits/{core, common} supports ngrx.
https://github.com/gabrielguerrero/ngrx-traits
NGRX Traits is a library to help you compose and reuse state logic in your angular app. There is two versions, @ngrx-traits/signals supports ngrx-signals, and @ngrx-traits/{core, common} supports ...
- 859RxJS-toolbox - set of custom operators and handy factory functions for RxJS
https://github.com/kievsash/rxjs-toolbox
RxJS-toolbox - set of custom operators and handy factory functions for RxJS - kievsash/rxjs-toolbox
- 860Angular service for saving data to CSV file.
https://github.com/rars/ng2csv
Angular service for saving data to CSV file. Contribute to rars/ng2csv development by creating an account on GitHub.
- 861See through the observables.
https://github.com/ksz-ksz/rxjs-insights
See through the observables. Contribute to ksz-ksz/rxjs-insights development by creating an account on GitHub.
- 862devkit/packages/rx-computed at main · jscutlery/devkit
https://github.com/jscutlery/devkit/tree/main/packages/rx-computed
Tools that make Angular developer's life easier. Contribute to jscutlery/devkit development by creating an account on GitHub.
- 863RxJS-based message bus service for Angular.
https://github.com/cristiammercado/ng-event-bus
RxJS-based message bus service for Angular. Contribute to cristiammercado/ng-event-bus development by creating an account on GitHub.
- 864Google fonts font picker widget for Angular (version 2 and newer)
https://github.com/zefoy/ngx-font-picker
Google fonts font picker widget for Angular (version 2 and newer) - zefoy/ngx-font-picker
- 865Lightweight Modularity for TypeScript Projects
https://github.com/softarc-consulting/sheriff
Lightweight Modularity for TypeScript Projects. Contribute to softarc-consulting/sheriff development by creating an account on GitHub.
- 866📄 PDF Viewer Component for Angular
https://github.com/VadimDez/ng2-pdf-viewer
📄 PDF Viewer Component for Angular. Contribute to VadimDez/ng2-pdf-viewer development by creating an account on GitHub.
- 867Parse your Angular code base to JSON abstraction - Great for displaying APIs and running custom analysis
https://github.com/angular-experts-io/ng-parsel
Parse your Angular code base to JSON abstraction - Great for displaying APIs and running custom analysis - angular-experts-io/ng-parsel
- 868Angular component library for displaying diffs of text.
https://github.com/rars/ngx-diff
Angular component library for displaying diffs of text. - rars/ngx-diff
- 869Still afraid to do something with AST? Mutates the AST, not your brain.
https://github.com/IKatsuba/mutates
Still afraid to do something with AST? Mutates the AST, not your brain. - IKatsuba/mutates
- 870Preline UI is an open-source set of prebuilt UI components based on the utility-first Tailwind CSS framework.
https://github.com/htmlstreamofficial/preline
Preline UI is an open-source set of prebuilt UI components based on the utility-first Tailwind CSS framework. - htmlstreamofficial/preline
- 871Immer wrappers around NgRx methods createReducer, on, and ComponentStore
https://github.com/timdeschryver/ngrx-immer
Immer wrappers around NgRx methods createReducer, on, and ComponentStore - timdeschryver/ngrx-immer
- 872A full-blown PDF viewer for Angular 16, 17, and beyond
https://github.com/stephanrauh/ngx-extended-pdf-viewer
A full-blown PDF viewer for Angular 16, 17, and beyond - stephanrauh/ngx-extended-pdf-viewer
- 873Clear examples, explanations, and resources for RxJS
https://github.com/btroncone/learn-rxjs
Clear examples, explanations, and resources for RxJS - btroncone/learn-rxjs
- 874An Angular v7+ library to detect the device, OS, and browser details.
https://github.com/AhsanAyaz/ngx-device-detector
An Angular v7+ library to detect the device, OS, and browser details. - GitHub - AhsanAyaz/ngx-device-detector: An Angular v7+ library to detect the device, OS, and browser details.
- 875Flame graph for stack trace visualization written in Angular
https://github.com/mgechev/ngx-flamegraph
Flame graph for stack trace visualization written in Angular - mgechev/ngx-flamegraph
- 876Rxjs operator to debounce and audit simultaenously
https://github.com/loreanvictor/audit-debounce
Rxjs operator to debounce and audit simultaenously - loreanvictor/audit-debounce
- 877An Angular treeview component with checkbox
https://github.com/TapBeep/ngx-treeview2
An Angular treeview component with checkbox. Contribute to TapBeep/ngx-treeview2 development by creating an account on GitHub.
- 878Build software better, together
https://github.com/benmarch/angular-ui-tour.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 879RxJs In Practice Course - https://angular-university.io/course/rxjs-course
https://github.com/angular-university/rxjs-course
RxJs In Practice Course - https://angular-university.io/course/rxjs-course - angular-university/rxjs-course
- 880Simple syncing between @ngrx store and local storage
https://github.com/btroncone/ngrx-store-localstorage
Simple syncing between @ngrx store and local storage - btroncone/ngrx-store-localstorage
- 881SKY UX components for Angular
https://github.com/blackbaud/skyux
SKY UX components for Angular. Contribute to blackbaud/skyux development by creating an account on GitHub.
- 882A lightweight, configurable and reactive breadcrumbs for Angular 2+
https://github.com/udayvunnam/xng-breadcrumb
A lightweight, configurable and reactive breadcrumbs for Angular 2+ - udayvunnam/xng-breadcrumb
- 883Synchronize any reactive form to @ngrx/store (Angular)
https://github.com/larscom/ngrx-store-formsync
Synchronize any reactive form to @ngrx/store (Angular) - larscom/ngrx-store-formsync
- 884NgRx (with NgRx Data) -The Complete Guide
https://github.com/angular-university/ngrx-course
NgRx (with NgRx Data) -The Complete Guide. Contribute to angular-university/ngrx-course development by creating an account on GitHub.
- 885A modernized Angular 4+ query builder based on jquery QueryBuilder
https://github.com/zebzhao/Angular-QueryBuilder
A modernized Angular 4+ query builder based on jquery QueryBuilder - zebzhao/Angular-QueryBuilder
- 886Compile-time and runtime immutability for Angular signals
https://github.com/zuriscript/ngx-signal-immutability
Compile-time and runtime immutability for Angular signals - zuriscript/ngx-signal-immutability
- 887All the Fake Data for All Your Real Needs 🙂
https://github.com/ngneat/falso
All the Fake Data for All Your Real Needs 🙂. Contribute to ngneat/falso development by creating an account on GitHub.
- 888Highly configurable state sync library between localStorage/sessionStorage and @ngrx/store (Angular)
https://github.com/larscom/ngrx-store-storagesync
Highly configurable state sync library between localStorage/sessionStorage and @ngrx/store (Angular) - larscom/ngrx-store-storagesync
- 889Responding to idle users in Angular (not AngularJS) applications.
https://github.com/moribvndvs/ng2-idle
Responding to idle users in Angular (not AngularJS) applications. - moribvndvs/ng2-idle
- 890🦊 RxJS operator that unsubscribe from observables on destroy
https://github.com/ngneat/until-destroy
🦊 RxJS operator that unsubscribe from observables on destroy - ngneat/until-destroy
- 891Fast and reliable Bootstrap widgets in Angular (supports Ivy engine)
https://github.com/valor-software/ngx-bootstrap
Fast and reliable Bootstrap widgets in Angular (supports Ivy engine) - valor-software/ngx-bootstrap
- 892Generate types and converters from JSON, Schema, and GraphQL
https://github.com/glideapps/quicktype
Generate types and converters from JSON, Schema, and GraphQL - glideapps/quicktype
- 893RxJS operators for Angular
https://github.com/nilsmehlhorn/ngx-operators
RxJS operators for Angular. Contribute to nilsmehlhorn/ngx-operators development by creating an account on GitHub.
- 894A collection of reactive wrappers for various browser APIs.
https://github.com/chrisguttandin/subscribable-things
A collection of reactive wrappers for various browser APIs. - chrisguttandin/subscribable-things
- 895CSV Parser for Angular by Developers Hive
https://github.com/tofiqquadri/ngx-csv-parser
CSV Parser for Angular by Developers Hive. Contribute to tofiqquadri/ngx-csv-parser development by creating an account on GitHub.
- 896Simplified API for working with Web Workers with RxJS
https://github.com/cloudnc/observable-webworker
Simplified API for working with Web Workers with RxJS - cloudnc/observable-webworker
- 897Your module to handle with feature toggles in Angular applications easier.
https://github.com/willmendesneto/ngx-feature-toggle
Your module to handle with feature toggles in Angular applications easier. - willmendesneto/ngx-feature-toggle
- 898The modular and type safe schema library for validating structural data 🤖
https://github.com/fabian-hiller/valibot
The modular and type safe schema library for validating structural data 🤖 - fabian-hiller/valibot
- 899Tailwind CSS components for Angular. Super easy to use and customize.
https://github.com/William-Mba/ngxtw
Tailwind CSS components for Angular. Super easy to use and customize. - William-Mba/ngxtw
- 900🧙♀️ Move Fast and Break Nothing. End-to-end typesafe APIs made easy.
https://github.com/trpc/trpc
🧙♀️ Move Fast and Break Nothing. End-to-end typesafe APIs made easy. - GitHub - trpc/trpc: 🧙♀️ Move Fast and Break Nothing. End-to-end typesafe APIs made easy.
- 901Native features for mobile apps built with Cordova/PhoneGap and open web technologies. Complete with TypeScript support.
https://github.com/danielsogl/awesome-cordova-plugins
Native features for mobile apps built with Cordova/PhoneGap and open web technologies. Complete with TypeScript support. - GitHub - danielsogl/awesome-cordova-plugins: Native features for mobile a...
- 902The components library for enterprise-level projects based on Tailwind CSS and Angular.
https://github.com/david-ui-org/david-ui-angular
The components library for enterprise-level projects based on Tailwind CSS and Angular. - GitHub - david-ui-org/david-ui-angular: The components library for enterprise-level projects based on Tail...
- 903All essential TypeScript types in one place 🤙
https://github.com/ts-essentials/ts-essentials
All essential TypeScript types in one place 🤙. Contribute to ts-essentials/ts-essentials development by creating an account on GitHub.
- 904This library allows you to reduce ngrx boilerplate by generating action bundles for common ngrx redux store scenarios and allows you to easily connect state, dispatch actions and listen for actions everywhere across your applications
https://github.com/IliaIdakiev/ngrx-action-bundles
This library allows you to reduce ngrx boilerplate by generating action bundles for common ngrx redux store scenarios and allows you to easily connect state, dispatch actions and listen for actions...
- 905IDEA's extra components and services built on Ionic
https://github.com/iter-idea/IDEA-Ionic8-extra
IDEA's extra components and services built on Ionic - iter-idea/IDEA-Ionic8-extra
- 906A version of angular2-query-builder to work with Angular 12+ (Need maintainers)
https://github.com/raysuelzer/ngx-angular-query-builder
A version of angular2-query-builder to work with Angular 12+ (Need maintainers) - raysuelzer/ngx-angular-query-builder
- 907Simple library to deal with cookies in Angular2
https://github.com/BCJTI/ng2-cookies
Simple library to deal with cookies in Angular2. Contribute to BCJTI/ng2-cookies development by creating an account on GitHub.
- 908Infinite Viewer is Document Viewer Component with infinite scrolling.
https://github.com/daybrush/infinite-viewer
Infinite Viewer is Document Viewer Component with infinite scrolling. - daybrush/infinite-viewer
- 909This angular module contains a component which generates tag clouds.
https://github.com/d-koppenhagen/angular-tag-cloud-module
This angular module contains a component which generates tag clouds. - d-koppenhagen/angular-tag-cloud-module
- 910Library for observing the lifecycle of an (ivy compiled) angular component
https://github.com/cloudnc/ngx-observable-lifecycle
Library for observing the lifecycle of an (ivy compiled) angular component - cloudnc/ngx-observable-lifecycle
- 911ngx-view-state library for handling Loading/Success/Error in NgRx
https://github.com/yurakhomitsky/ngx-view-state
ngx-view-state library for handling Loading/Success/Error in NgRx - yurakhomitsky/ngx-view-state
- 912JeanMeche/angular-compiler-output
https://github.com/JeanMeche/angular-compiler-output
Contribute to JeanMeche/angular-compiler-output development by creating an account on GitHub.
- 913Photo Editor using @ionic/angular
https://github.com/rdlabo-team/ionic-angular-library
Photo Editor using @ionic/angular. Contribute to rdlabo-team/ionic-angular-library development by creating an account on GitHub.
- 914ftischler/ngx-rxjs-zone-scheduler
https://github.com/ftischler/ngx-rxjs-zone-scheduler
Contribute to ftischler/ngx-rxjs-zone-scheduler development by creating an account on GitHub.
- 915ng-lock
https://www.npmjs.com/package/ng-lock
Angular decorator for lock a function and user interface while a task running.. Latest version: 18.0.15, last published: 17 days ago. Start using ng-lock in your project by running `npm i ng-lock`. There are no other projects in the npm registry using ng-lock.
- 916Signal Store feature that bridges with Angular Query
https://github.com/k3nsei/ngx-signal-store-query
Signal Store feature that bridges with Angular Query - k3nsei/ngx-signal-store-query
- 917This is an Angular library that provides an easy way to load a configuration JSON file for runtime configuration.
https://github.com/pjlamb12/runtime-config-loader
This is an Angular library that provides an easy way to load a configuration JSON file for runtime configuration. - pjlamb12/runtime-config-loader
- 918📖 Pagination Component for Angular applications
https://github.com/sibiraj-s/angular-paginator
📖 Pagination Component for Angular applications. Contribute to sibiraj-s/angular-paginator development by creating an account on GitHub.
- 919TypeScript is a superset of JavaScript that compiles to clean JavaScript output.
https://github.com/Microsoft/TypeScript
TypeScript is a superset of JavaScript that compiles to clean JavaScript output. - microsoft/TypeScript
- 920An RxJS message broker for WebRTC DataChannels and WebSockets.
https://github.com/chrisguttandin/rxjs-broker
An RxJS message broker for WebRTC DataChannels and WebSockets. - chrisguttandin/rxjs-broker
- 921🎛️ JSON powered GUI for configurable panels.
https://github.com/acrodata/gui
🎛️ JSON powered GUI for configurable panels. Contribute to acrodata/gui development by creating an account on GitHub.
- 922Product Tour Wizard built with Angular
https://github.com/tonysamperi/ngx-tour-wizard
Product Tour Wizard built with Angular. Contribute to tonysamperi/ngx-tour-wizard development by creating an account on GitHub.
- 923Lightweight undo-redo for Angular with NgRx & immer.js
https://github.com/nilsmehlhorn/ngrx-wieder
Lightweight undo-redo for Angular with NgRx & immer.js - nilsmehlhorn/ngrx-wieder
- 924🪄 A framework-agnostic RxJS effects implementation
https://github.com/ngneat/effects
🪄 A framework-agnostic RxJS effects implementation - ngneat/effects
- 925Various Extensions for the NgRx Signal Store
https://github.com/angular-architects/ngrx-toolkit
Various Extensions for the NgRx Signal Store. Contribute to angular-architects/ngrx-toolkit development by creating an account on GitHub.
- 926RxJS middleware for action side effects in Redux using "Epics"
https://github.com/redux-observable/redux-observable
RxJS middleware for action side effects in Redux using "Epics" - redux-observable/redux-observable
- 927The repository for high quality TypeScript type definitions.
https://github.com/DefinitelyTyped/DefinitelyTyped
The repository for high quality TypeScript type definitions. - DefinitelyTyped/DefinitelyTyped
- 928Truly dynamic modules at runtime with Module Federation
https://github.com/LoaderB0T/ng-dynamic-mf
Truly dynamic modules at runtime with Module Federation - LoaderB0T/ng-dynamic-mf
- 929Make RTK Query with Hooks works in Angular Applications
https://github.com/SaulMoro/ngrx-rtk-query
Make RTK Query with Hooks works in Angular Applications - SaulMoro/ngrx-rtk-query
- 930Polymorpheus is a tiny library for polymorphic templates in Angular.
https://github.com/taiga-family/ng-polymorpheus
Polymorpheus is a tiny library for polymorphic templates in Angular. - taiga-family/ng-polymorpheus
- 931Easily use lorem-ipsum dummy texts in your angular app as directive, component or by using a service
https://github.com/d-koppenhagen/ngx-lipsum
Easily use lorem-ipsum dummy texts in your angular app as directive, component or by using a service - d-koppenhagen/ngx-lipsum
- 932Angular (4.2+ ...12) service for cookies. Originally based on the `ng2-cookies` library.
https://github.com/stevermeister/ngx-cookie-service
Angular (4.2+ ...12) service for cookies. Originally based on the `ng2-cookies` library. - stevermeister/ngx-cookie-service
- 933rdlabo-team/ionic-angular-collect-icons
https://github.com/rdlabo-team/ionic-angular-collect-icons
Contribute to rdlabo-team/ionic-angular-collect-icons development by creating an account on GitHub.
- 934Angular library to create beautiful stars with parallax effect
https://github.com/DerStimmler/ngx-parallax-stars
Angular library to create beautiful stars with parallax effect - DerStimmler/ngx-parallax-stars
- 935ORM selectors for redux, @ngrx/store, @ngrx/entity and @ngrx/data. Ease of relationships with entities.
https://github.com/satanTime/ngrx-entity-relationship
ORM selectors for redux, @ngrx/store, @ngrx/entity and @ngrx/data. Ease of relationships with entities. - satanTime/ngrx-entity-relationship
- 936Component property connection in Angular application wherever they are.
https://github.com/nigrosimone/ng-portal
Component property connection in Angular application wherever they are. - nigrosimone/ng-portal
- 937A simple and composable way to validate data in JavaScript (and TypeScript).
https://github.com/ianstormtaylor/superstruct
A simple and composable way to validate data in JavaScript (and TypeScript). - ianstormtaylor/superstruct
- 938Code mutations in your project or schematics were never easier than now.
https://github.com/taiga-family/ng-morph
Code mutations in your project or schematics were never easier than now. - taiga-family/ng-morph
- 939Simple, easy and performance countdown for angular
https://github.com/cipchk/ngx-countdown
Simple, easy and performance countdown for angular - cipchk/ngx-countdown
- 940Il toolkit Angular conforme alle linee guida di design per i servizi web della PA.
https://github.com/italia/design-angular-kit
Il toolkit Angular conforme alle linee guida di design per i servizi web della PA. - italia/design-angular-kit
- 941An Angular Material library for displaying walk-through pop-ups and dialogs using a declarative way.
https://github.com/Broadcom/bdc-walkthrough
An Angular Material library for displaying walk-through pop-ups and dialogs using a declarative way. - Broadcom/bdc-walkthrough
- 942Adds an abstraction layer / facade between Angular components and the @ngrx store
https://github.com/ngxp/store-service
Adds an abstraction layer / facade between Angular components and the @ngrx store - ngxp/store-service
- 943A set of challenges helping you understand TypeScript
https://github.com/psmyrdek/typescript-challenges
A set of challenges helping you understand TypeScript - przeprogramowani/typescript-challenges
- 944Marble diagram DSL based test suite for RxJS
https://github.com/kwonoj/rx-sandbox
Marble diagram DSL based test suite for RxJS. Contribute to kwonoj/rx-sandbox development by creating an account on GitHub.
- 945An Angular wrapper for the site tour library Shepherd
https://github.com/shepherd-pro/angular-shepherd
An Angular wrapper for the site tour library Shepherd - shipshapecode/angular-shepherd
- 946This library makes RxJS Observables testing easy!
https://github.com/hirezio/observer-spy
This library makes RxJS Observables testing easy! Contribute to hirezio/observer-spy development by creating an account on GitHub.
- 947Collection of TypeScript type challenges with online judge
https://github.com/type-challenges/type-challenges
Collection of TypeScript type challenges with online judge - type-challenges/type-challenges
- 948🎨 The exhaustive Pattern Matching library for TypeScript, with smart type inference.
https://github.com/gvergnaud/ts-pattern
🎨 The exhaustive Pattern Matching library for TypeScript, with smart type inference. - gvergnaud/ts-pattern
- 949Json Schema Type Builder with Static Type Resolution for TypeScript
https://github.com/sinclairzx81/typebox
Json Schema Type Builder with Static Type Resolution for TypeScript - sinclairzx81/typebox
- 950TypeScript-first schema validation with static type inference
https://github.com/colinhacks/zod
TypeScript-first schema validation with static type inference - colinhacks/zod
Related Articlesto learn about angular.
- 1Getting Started with AngularJS: Building Your First Application
- 2AngularJS Directives: Building Dynamic Web Pages
- 3AngularJS Services: Creating Reusable Business Logic
- 4AngularJS Controllers vs Components: Choosing the Right Approach
- 5Building Scalable AngularJS Applications: Application Architecture
- 6AngularJS Routing: Building Single-Page Applications (SPA)
- 7State Management in AngularJS: Handling Complex Application State
- 8Using HTTP in AngularJS: Fetching Data from APIs
- 9AngularJS Performance: Guide to Optimizing Your App
- 10Integrating AngularJS with Modern Development Tools
FAQ'sto learn more about Angular JS.
mail [email protected] to add more queries here 🔍.
- 1
how to create an array in angular js
- 2
is angular js easy to learn
- 3
can you use jsx in angular
- 4
why node js for angular
- 5
is angularjs still supported
- 6
do angularjs unit testing
- 7
when does angularjs support end
- 8
what is zone.js in angular
- 9
what is angular js and node js
- 10
why do we use angular js
- 11
who uses angular js
- 12
who made angularjs
- 13
is angular js deprecated
- 14
can we use js in angular
- 15
why node js is required for angular
- 16
does angularjs support es6
- 17
how to install angular js
- 18
where is angular.json
- 19
how to create json file in angular
- 20
is angular js supported
- 21
does angular have jsx
- 22
is angularjs dead
- 23
what is the latest version of angular js
- 24
how to make an ajax call using angular js
- 25
which is the latest version of angular js
- 26
why did angularjs die
- 27
why angularjs is discontinued
- 28
when was angularjs discontinued
- 29
are angular and angular js the same
- 30
which of the following is not a valid angularjs filter
- 31
is angularjs end of life
- 32
what does angularjs do
- 33
what is tsconfig.json in angular
- 34
which angularjs directive
- 35
when was angularjs released
- 36
when to use angular js
- 37
does angularjs use typescript
- 38
who wrote angular
- 39
what is routing in angular js
- 40
is angular declining
- 41
is angular js still used
- 42
where to learn angular js
- 43
what do you mean by angularjs
- 44
who developed angularjs
- 45
is angularjs the same as angular
- 46
when did angularjs become angular
- 47
what is the difference between angular and angular js
- 48
where to use angular.js
- 49
what is angular.json file
- 50
how angularjs works
- 51
how to check angular version in package.json
- 52
why angular is faster than angularjs
- 53
when did angularjs come out
- 54
why angular need node js
- 55
does angularjs support typescript
- 56
when in angular js
- 57
what is package.json in angular
- 58
where is angular-cli.json
- 59
who created angular js
- 60
what are angular js directives
- 61
is angular js discontinued
- 62
what are angular js
- 63
why was angularjs deprecated
- 64
how to install angular js in windows
- 65
why do we need node js for angular
- 66
who supports angular
- 67
what are the features of angular js
- 68
why use angular js
- 69
can we write js code in angular
- 70
is angularjs deprecated
- 71
can angularjs and angular coexist
- 72
should i commit angular.json
- 73
what are filters in angular js
- 74
what are the advantages of angular js
- 75
is angularjs still used
- 76
what are services in angular js
- 77
is angular js and angular same
- 78
is angular js dead
- 79
what does angular.json file contains
- 80
should i use angularjs
- 81
which community angularjs belongs to
- 82
where is main.js in angular
- 83
does angularjs use jquery
- 84
how to update zone.js version in angular
- 85
what is angular.json
- 86
does angularjs support dependency injection
- 87
why angular js is used
- 88
how to import json file in angular
- 89
which of the following community angularjs belong to
- 90
which of the following statement is correct for angular js
- 91
is angular js frontend or backend
- 92
what does angular.json do
- 93
where to check angular version in package.json
- 94
why angular is better than javascript
- 95
who uses angular
- 96
what does zone.js do in angular
- 97
does angularjs use es6
- 98
what does angular.json file do
- 99
is angular js a framework
- 100
where to find webpack.config.js in angular
- 101
how to download angular js
- 102
why we use angular js
- 103
how to do angularjs in javascript
- 104
how angularjs integrates with html
- 105
which version of node js is supported by angular
- 106
how to do sorting in angular js
- 107
how to run json server in angular
More Sitesto check out once you're finished browsing here.
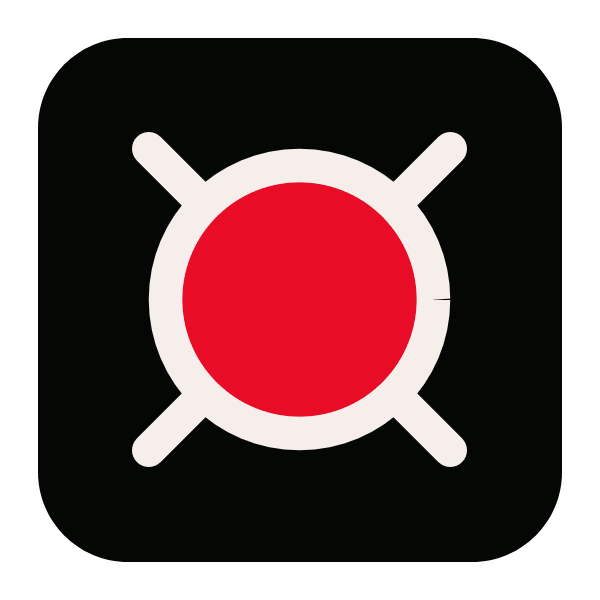
https://www.0x3d.site/
0x3d is designed for aggregating information.
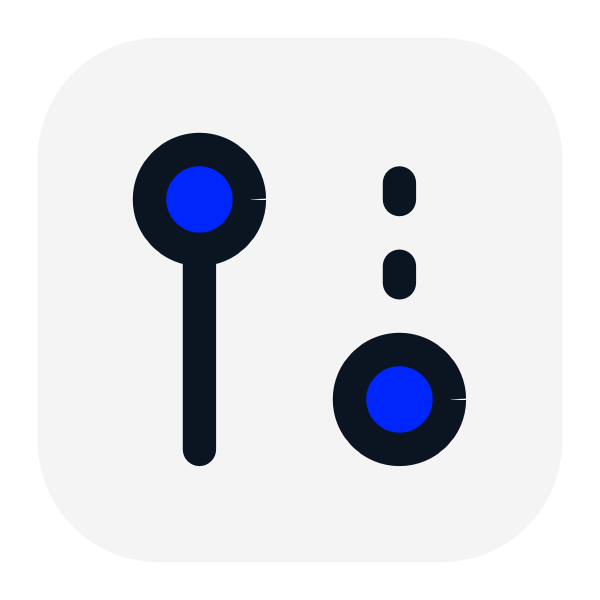
https://nodejs.0x3d.site/
NodeJS Online Directory

https://cross-platform.0x3d.site/
Cross Platform Online Directory
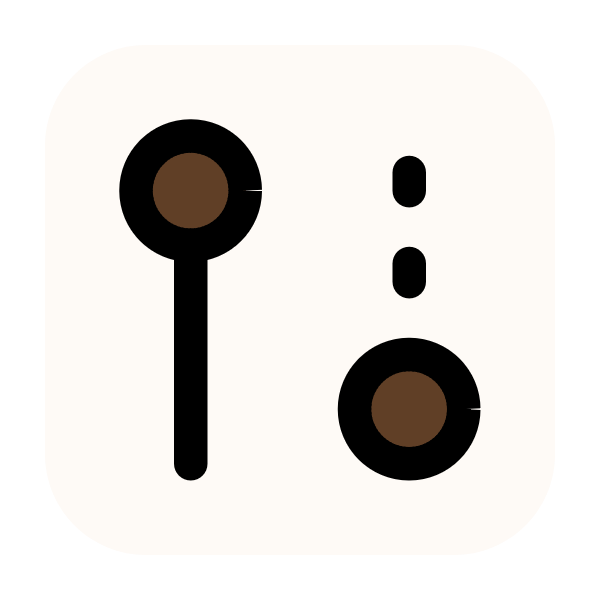
https://open-source.0x3d.site/
Open Source Online Directory
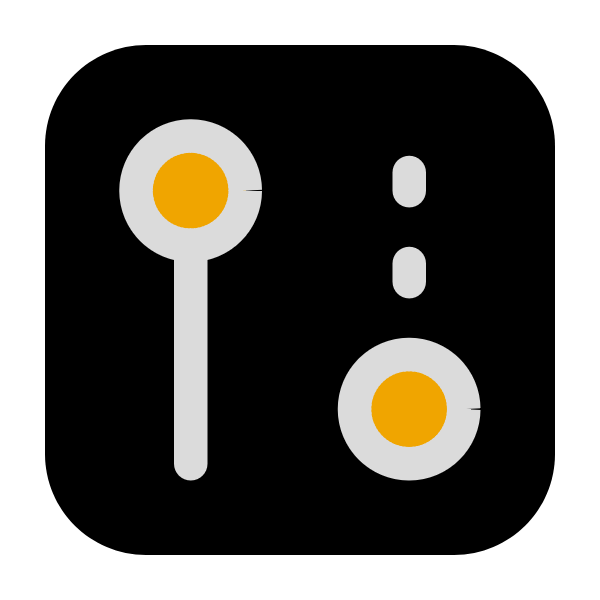
https://analytics.0x3d.site/
Analytics Online Directory
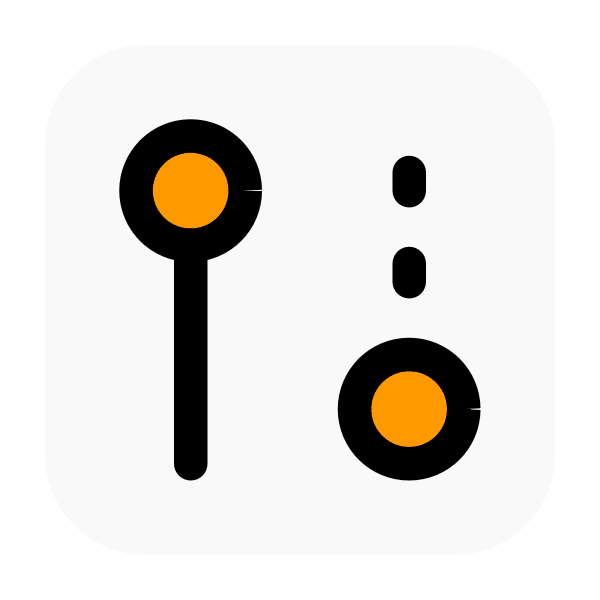
https://javascript.0x3d.site/
JavaScript Online Directory

https://golang.0x3d.site/
GoLang Online Directory
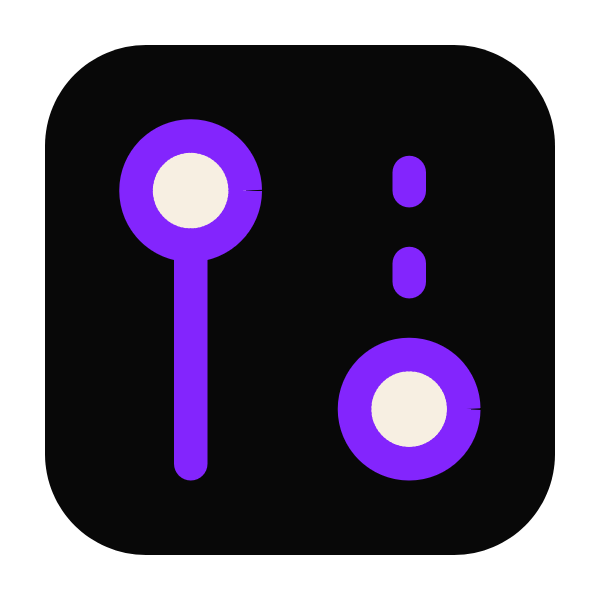
https://python.0x3d.site/
Python Online Directory

https://swift.0x3d.site/
Swift Online Directory

https://rust.0x3d.site/
Rust Online Directory
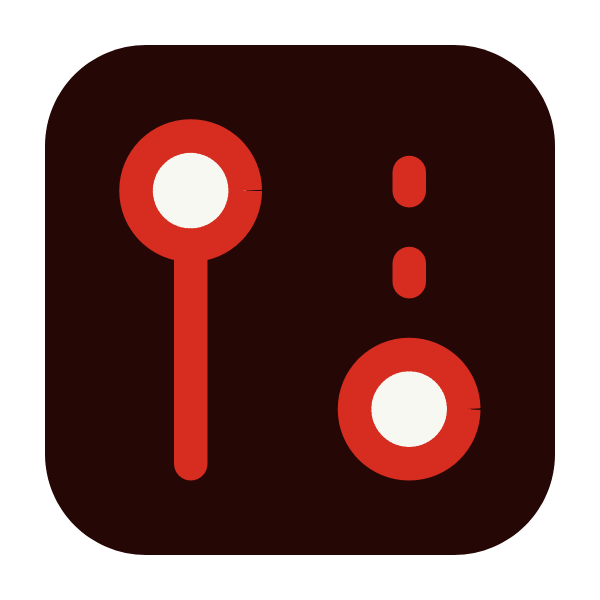
https://scala.0x3d.site/
Scala Online Directory

https://ruby.0x3d.site/
Ruby Online Directory

https://clojure.0x3d.site/
Clojure Online Directory
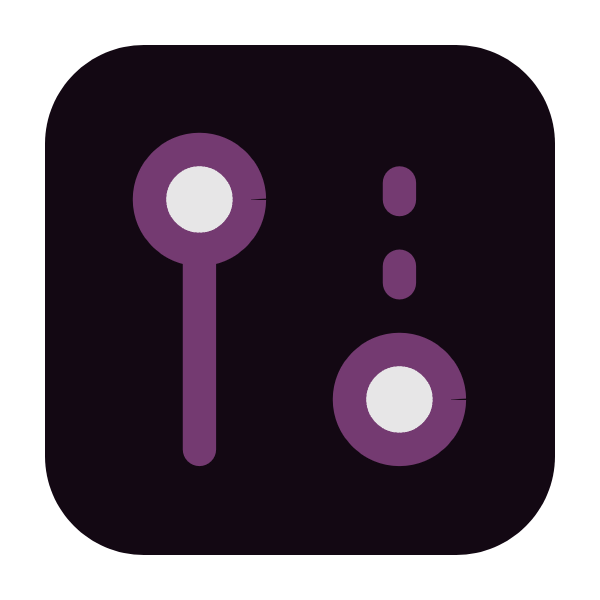
https://elixir.0x3d.site/
Elixir Online Directory
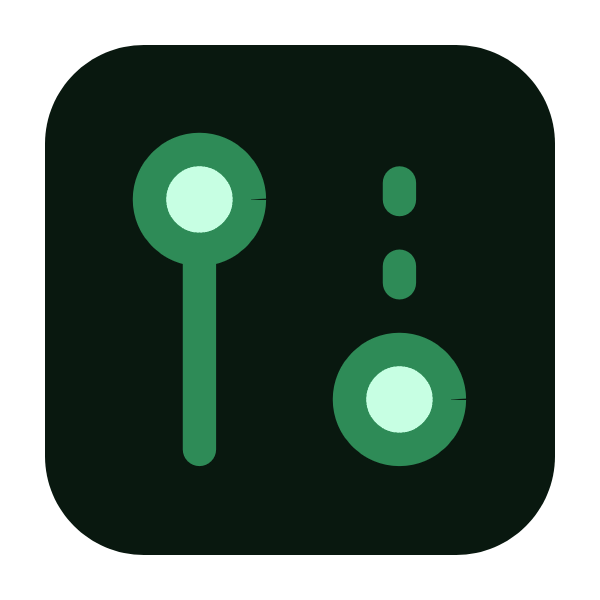
https://elm.0x3d.site/
Elm Online Directory

https://lua.0x3d.site/
Lua Online Directory
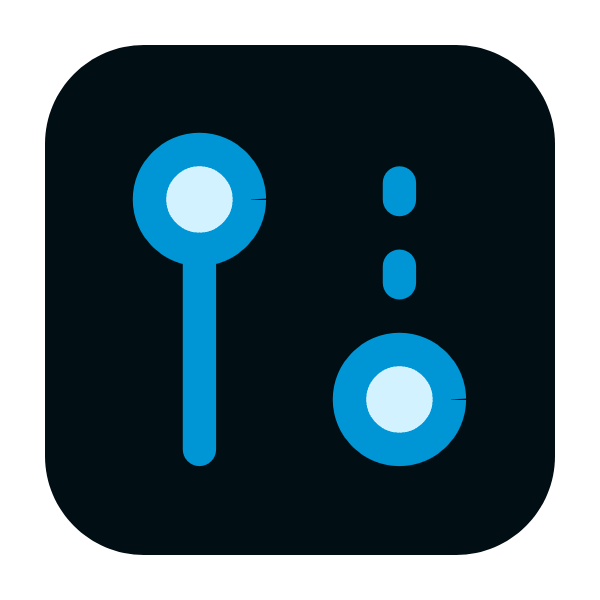
https://c-programming.0x3d.site/
C Programming Online Directory
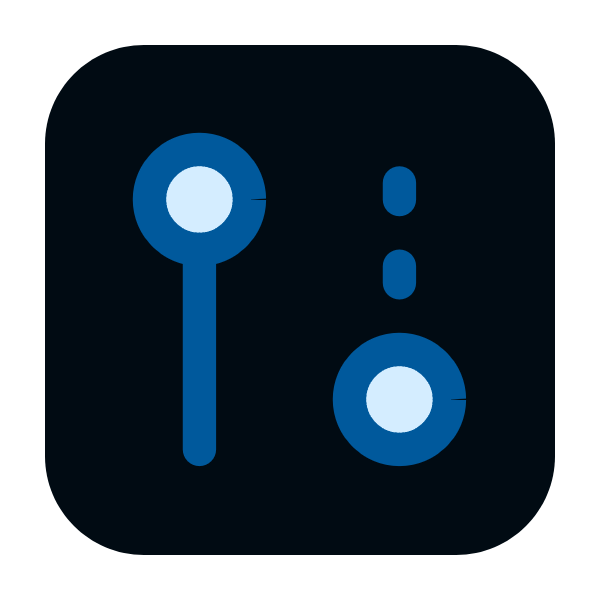
https://cpp-programming.0x3d.site/
C++ Programming Online Directory

https://r-programming.0x3d.site/
R Programming Online Directory

https://perl.0x3d.site/
Perl Online Directory
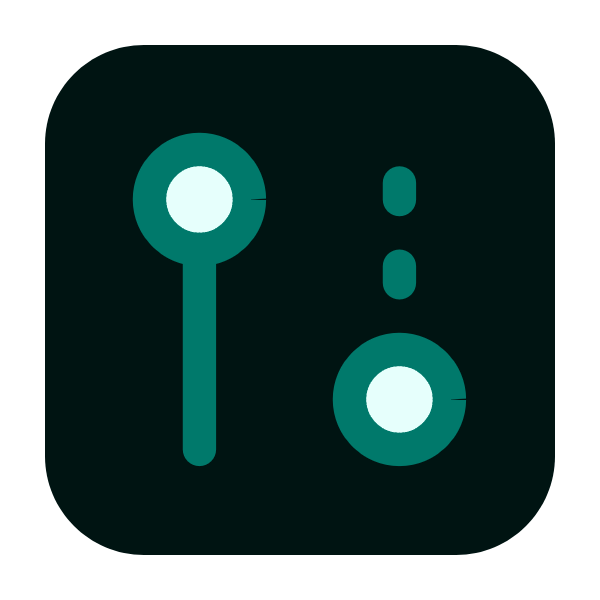
https://java.0x3d.site/
Java Online Directory

https://kotlin.0x3d.site/
Kotlin Online Directory

https://php.0x3d.site/
PHP Online Directory

https://react.0x3d.site/
React JS Online Directory
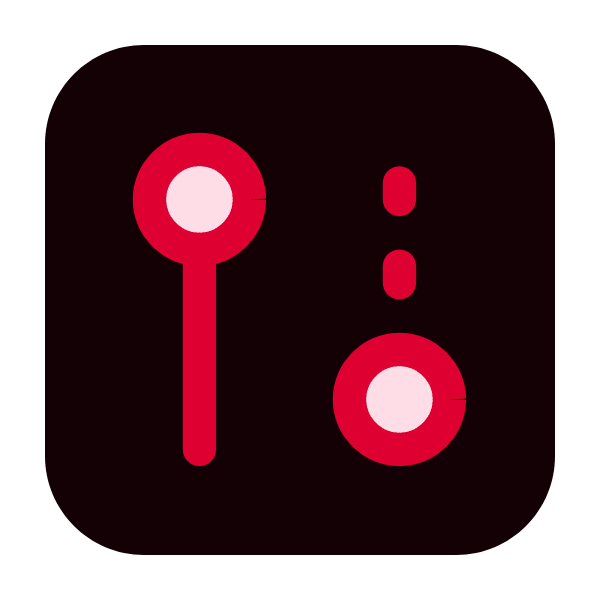
https://angular.0x3d.site/
Angular JS Online Directory